React Lifecycle Methods
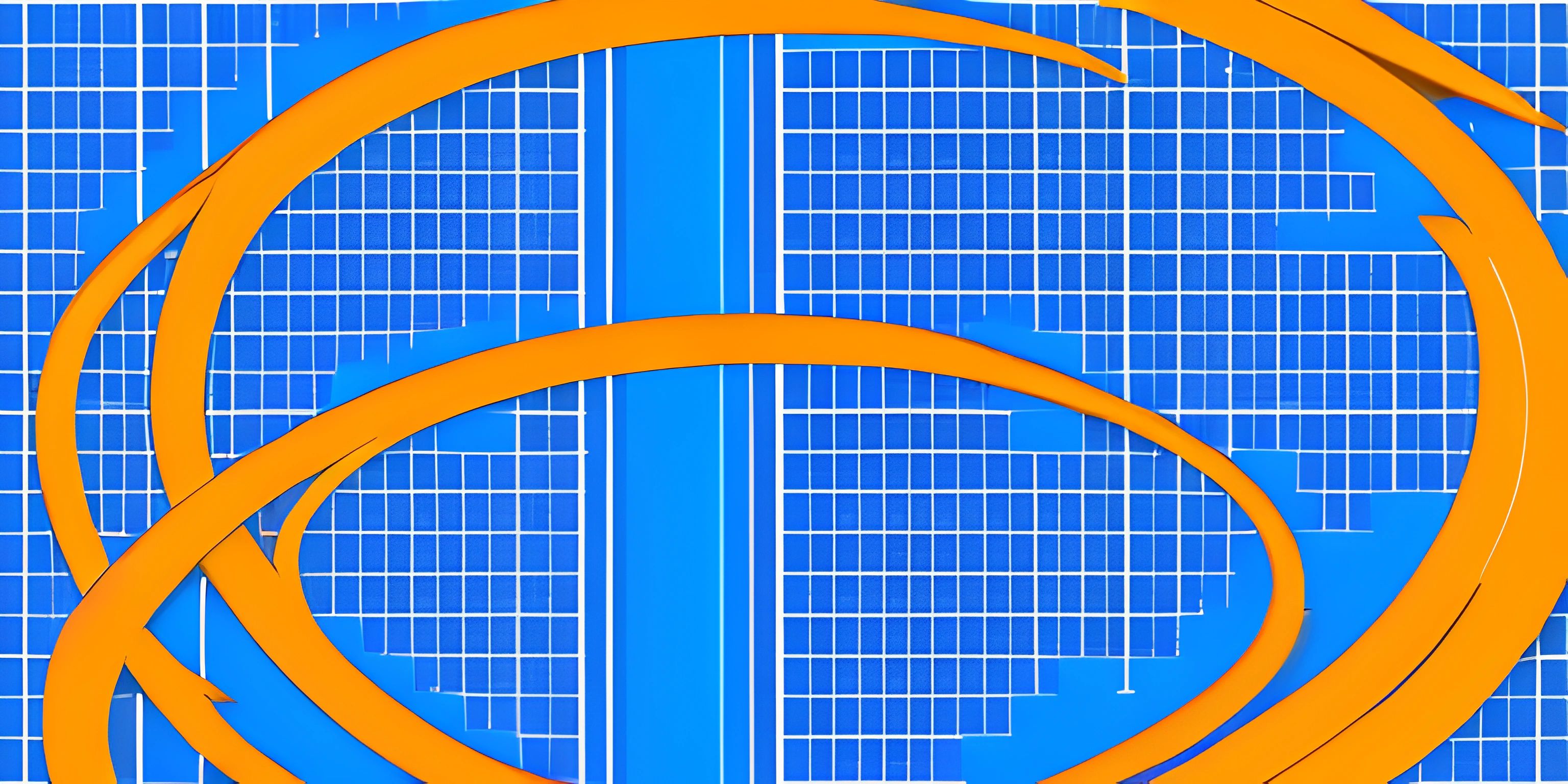
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
React is all about creating and managing components in a user interface. To have full control over these components and their behavior, we need to understand React Component Lifecycle Methods. These methods are special functions provided by React that allow us to respond to specific events during a component's life, such as mounting, updating, and unmounting.
Component Lifecycle Overview
A React component goes through several stages during its life, from being created and added to the DOM to being updated and finally removed. These stages can be divided into three main categories: Mounting, Updating, and Unmounting.
Mounting
Mounting is the phase when a component is created and inserted into the DOM. During this phase, the following lifecycle methods are called:
constructor
static getDerivedStateFromProps
render
componentDidMount
Updating
Updating occurs when a component's state or props change, causing a re-render. The following lifecycle methods are called during this phase:
static getDerivedStateFromProps
shouldComponentUpdate
render
getSnapshotBeforeUpdate
componentDidUpdate
Unmounting
Unmounting is the final phase when a component is removed from the DOM. The only lifecycle method called during this phase is componentWillUnmount
.
Lifecycle Methods in Detail
Now that we have a broad understanding of the component lifecycle, let's dive into the individual lifecycle methods and explore their use cases.
constructor
The constructor
is the first method called when a component is instantiated. It's typically used for setting up the component's initial state and binding event handlers.
class MyComponent extends React.Component { constructor(props) { super(props); this.state = { count: 0 }; this.handleClick = this.handleClick.bind(this); } // ... rest of the component ... }
static getDerivedStateFromProps
static getDerivedStateFromProps
is called when a component is created and whenever its props change. This method is useful when we need to update the component's state based on changes in its props.
class MyComponent extends React.Component { // ... constructor and other methods ... static getDerivedStateFromProps(props, state) { return { count: props.initialCount }; } }
shouldComponentUpdate
shouldComponentUpdate
is called before rendering when new props or state are being received. This method allows us to optimize performance by preventing unnecessary re-renders.
class MyComponent extends React.Component { // ... other methods ... shouldComponentUpdate(nextProps, nextState) { return nextProps.value !== this.props.value; } }
render
render
is the most important lifecycle method, responsible for describing what the component should look like. It returns the JSX representation of the component's UI.
class MyComponent extends React.Component { // ... other methods ... render() { return ( <div> <p>Count: {this.state.count}</p> <button onClick={this.handleClick}>Increment</button> </div> ); } }
componentDidMount
componentDidMount
is called once the component has been rendered and added to the DOM. This is where we perform any necessary setup, such as fetching data or adding event listeners.
class MyComponent extends React.Component { // ... other methods ... componentDidMount() { this.interval = setInterval(() => this.tick(), 1000); } tick() { this.setState({ count: this.state.count + 1 }); } }
componentDidUpdate
componentDidUpdate
is called after a component has been updated and re-rendered. This is the perfect place to perform side effects based on prop or state changes.
class MyComponent extends React.Component { // ... other methods ... componentDidUpdate(prevProps, prevState) { if (prevProps.value !== this.props.value) { this.fetchData(this.props.value); } } }
componentWillUnmount
componentWillUnmount
is called right before a component is removed from the DOM. This is where we clean up any resources, such as timers or event listeners, that were created in componentDidMount
.
class MyComponent extends React.Component { // ... other methods ... componentWillUnmount() { clearInterval(this.interval); } }
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Full-stack Web Frameworks (Next.js) (psst, it's free!).
FAQ
What are React Component Lifecycle Methods?
React Component Lifecycle Methods are special functions provided by React that allow developers to respond to specific events in a component's life, such as mounting, updating, and unmounting. They help control the behavior of components and handle side effects throughout the application.
What are the three main categories of the component lifecycle?
The three main categories of the component lifecycle are Mounting (component creation and insertion into the DOM), Updating (when a component's state or props change, causing a re-render), and Unmounting (when a component is removed from the DOM).
What is the purpose of the `render` method in a React component?
The render
method is responsible for describing what the component's user interface should look like. It returns the JSX representation of the component's UI, which React uses to update the actual DOM.