React & TypeScript: Components and State
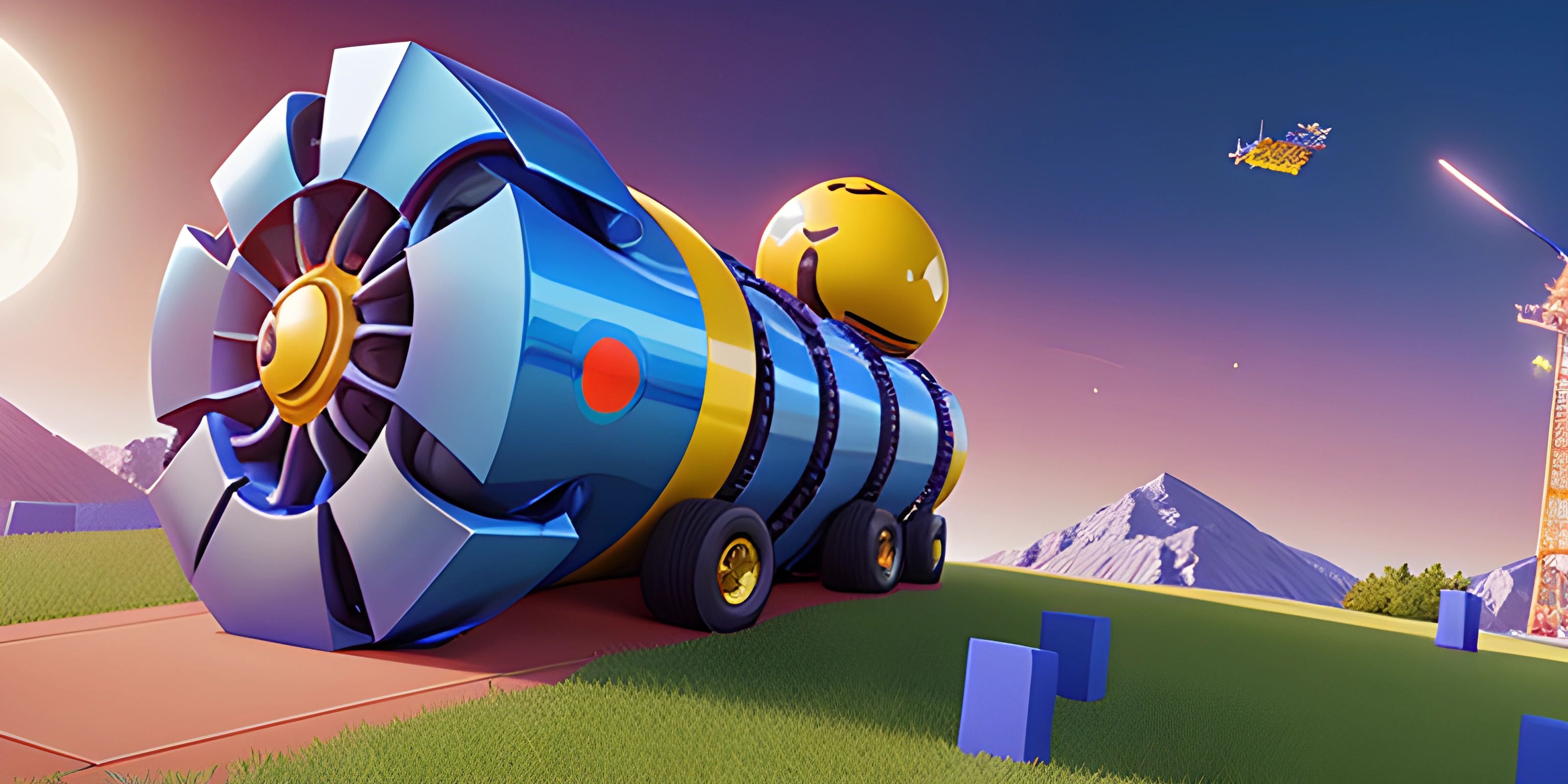
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Diving into the world of React using TypeScript, we come across two significant players in the game - components and state. Let's brush up on our understanding of these entities!
Components
In React, everything you see on the screen is a component. Think of your web application like a giant Lego set. Each component is a Lego brick, and by assembling these bricks in different ways, you can create magnificent structures (or in our case, a dazzling web application).
Now, toss TypeScript into the mix, and suddenly our Lego bricks are smarter. They have an understanding of what type of brick they are, and how they should interact with other bricks, ensuring that a rectangular brick will never try to fit into a circular hole.
Here's a basic example of a React component written in TypeScript:
import React from "react"; interface GreetingProps { name: string; } const Greeting: React.FC<GreetingProps> = ({ name }) => { return <h1>Hello, {name}!</h1>; } export default Greeting;
In this snippet, Greeting
is a functional component. It takes in a name
as a prop and uses that to display a personalized greeting. Notice the GreetingProps
interface? That's TypeScript giving our Lego brick its identity, ensuring we always pass in a name
, and that it's always a string.
State
State in React is like the secret sauce in your grandma's special recipe. It's what gives your application life, allowing it to interact and respond to the user's actions.
In the world of TypeScript and React, state management gets a bit more exciting. TypeScript ensures that our state always behaves as expected, adding an extra layer of predictability to our application. Let's take a look at how we can use the useState
hook with TypeScript:
import React, { useState } from "react"; const Counter: React.FC = () => { const [count, setCount] = useState<number>(0); return ( <div> <p>You clicked {count} times</p> <button onClick={() => setCount(count + 1)}> Click me </button> </div> ); } export default Counter;
In this example, count
is a state variable that's always a number. The setCount
function is how we update count
. TypeScript ensures that setCount
only accepts numbers, preventing any surprises.
In essence, components are the building blocks of our application, and state is what makes these blocks interactive. Add TypeScript to the equation, and you get predictable, type-safe applications that are a joy to develop!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Full-stack Web Frameworks (Next.js) (psst, it's free!).
FAQ
What is a component in React?
In React, a component is a self-contained piece of code that represents a part of the user interface (UI). It's like a Lego brick that you can reuse and combine to build a complex structure.
What is state in React?
State in React refers to the data stored within a component that can change over time. It's the component's memory and can affect the component's behavior, output, and rendered HTML.
How does TypeScript benefit React development?
TypeScript provides static type checking to React. This means it checks the types of your variables, state, props, etc., at compile time, helping catch errors early. It also enhances code autocompletion, making development faster and more efficient.
Can I use TypeScript with functional components in React?
Absolutely! Just like in our examples, TypeScript can be used with both functional and class-based components in React.
How do I define state in a React component using TypeScript?
You define state in a React component using TypeScript by using the useState
hook, and specify the type of the state inside the angle brackets, like so: useState<number>(0)
. This tells TypeScript that the state will always be a number.