ReactJS for the Impatient
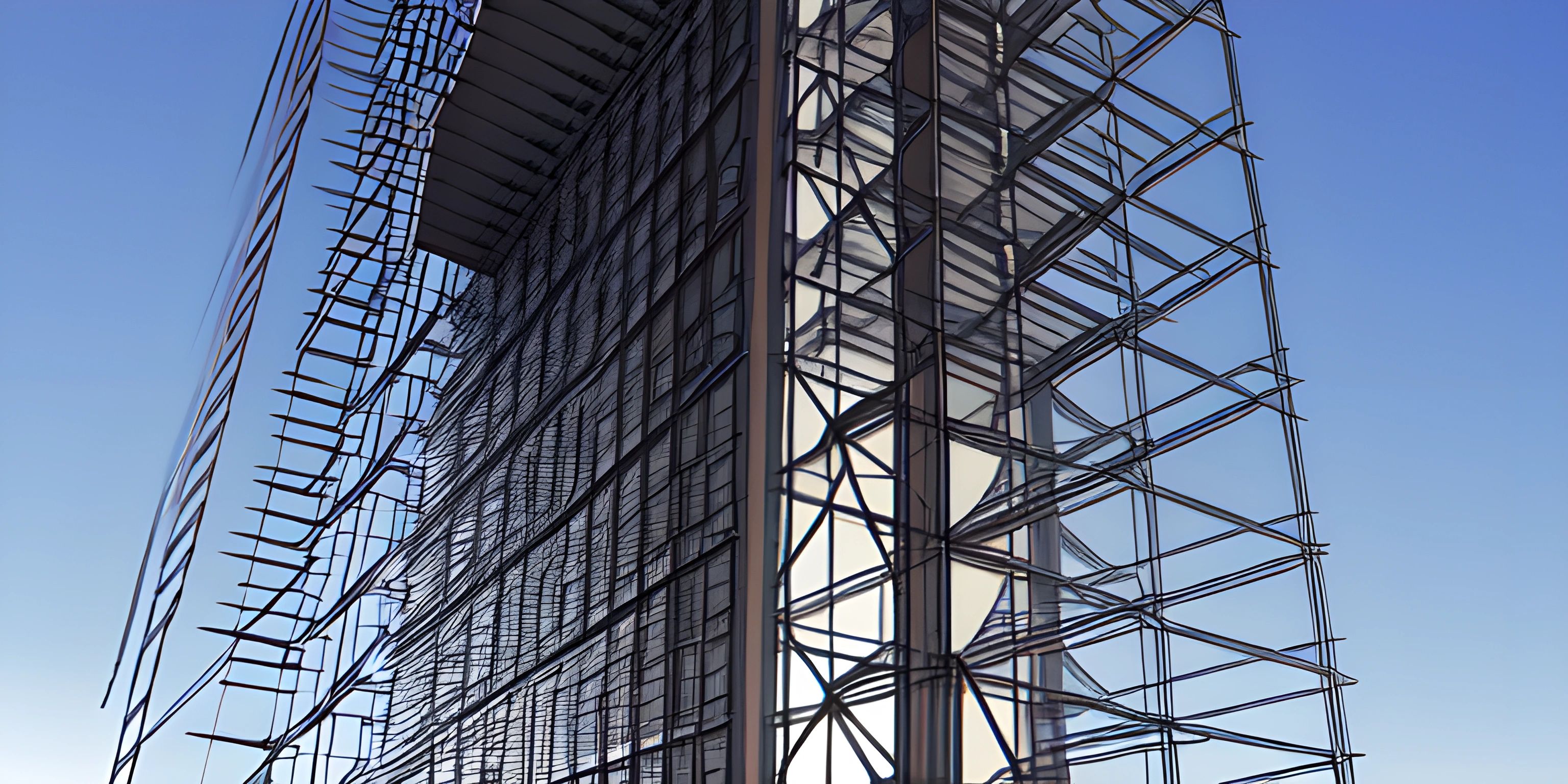
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Patience may be a virtue, but sometimes you just want to dive right into a new technology and get started. Enter ReactJS, a popular JavaScript library for building user interfaces. With its component-based structure and powerful JSX syntax, you'll be creating interactive web applications in no time. Let's jump right in.
What is ReactJS?
ReactJS is an open-source JavaScript library developed by Facebook for building user interfaces. Its primary philosophy is to create reusable UI components that manage their own state, making it easier to compose complex UIs. ReactJS is particularly useful for building single-page applications, where the user interacts with the page without having to refresh it.
JSX: The Secret Sauce
JSX is a syntax extension for JavaScript that allows you to write HTML-like code within your JavaScript code. It's not required when using React, but it makes writing components more comfortable and more readable.
Here's a simple example of JSX:
const element = ( <h1>Hello, Cratecode!</h1> );
JSX is converted to JavaScript during the build process, so the above code becomes:
const element = React.createElement( 'h1', null, 'Hello, Cratecode!' );
Components: The Building Blocks of a React App
Components are the foundation of any React application. They're reusable, self-contained pieces of UI that receive input (called "props") and return React elements describing what should appear on the screen.
Here's an example of a simple React component:
function Welcome(props) { return <h1>Hello, {props.name}!</h1>; }
You can create a more complex component by composing other components together:
function App() { return ( <div> <Welcome name="Cratecode" /> <Welcome name="Reader" /> <Welcome name="ReactJS" /> </div> ); }
State: Local Storage for Components
State is an essential concept in React. It represents the local storage for a component, holding data that can change over time. When a component's state changes, React will automatically re-render the component.
Here's an example of a component using state:
class Counter extends React.Component { constructor(props) { super(props); this.state = {count: 0}; } incrementCount() { this.setState({count: this.state.count + 1}); } render() { return ( <div> <h1>Count: {this.state.count}</h1> <button onClick={() => this.incrementCount()}>Increment</button> </div> ); } }
In this example, the Counter
component has a state object with a count
property. When the "Increment" button is clicked, the incrementCount
method is called, updating the state and causing the component to re-render with the new count.
Time to Assemble Your Web Application
Now that you're equipped with the basics of ReactJS, JSX, components, and state, you're ready to build your web application. Remember, practice makes perfect, so don't be afraid to experiment and iterate on your designs. The more you work with React, the faster and more efficient you'll become.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Full-stack Web Frameworks (Next.js) (psst, it's free!).
FAQ
What is ReactJS?
ReactJS is an open-source JavaScript library developed by Facebook for building user interfaces. It focuses on creating reusable UI components that manage their own state, making it easier to compose complex UIs.
What is JSX?
JSX is a syntax extension for JavaScript that allows you to write HTML-like code within your JavaScript code. It's not required when using React, but it makes writing components more comfortable and more readable.
How do components work in ReactJS?
Components are the foundation of any React application. They're reusable, self-contained pieces of UI that receive input (called "props") and return React elements describing what should appear on the screen.
What is state in React?
State is an essential concept in React. It represents the local storage for a component, holding data that can change over time. When a component's state changes, React will automatically re-render the component.