Regular Expressions: The Magic of Pattern Matching
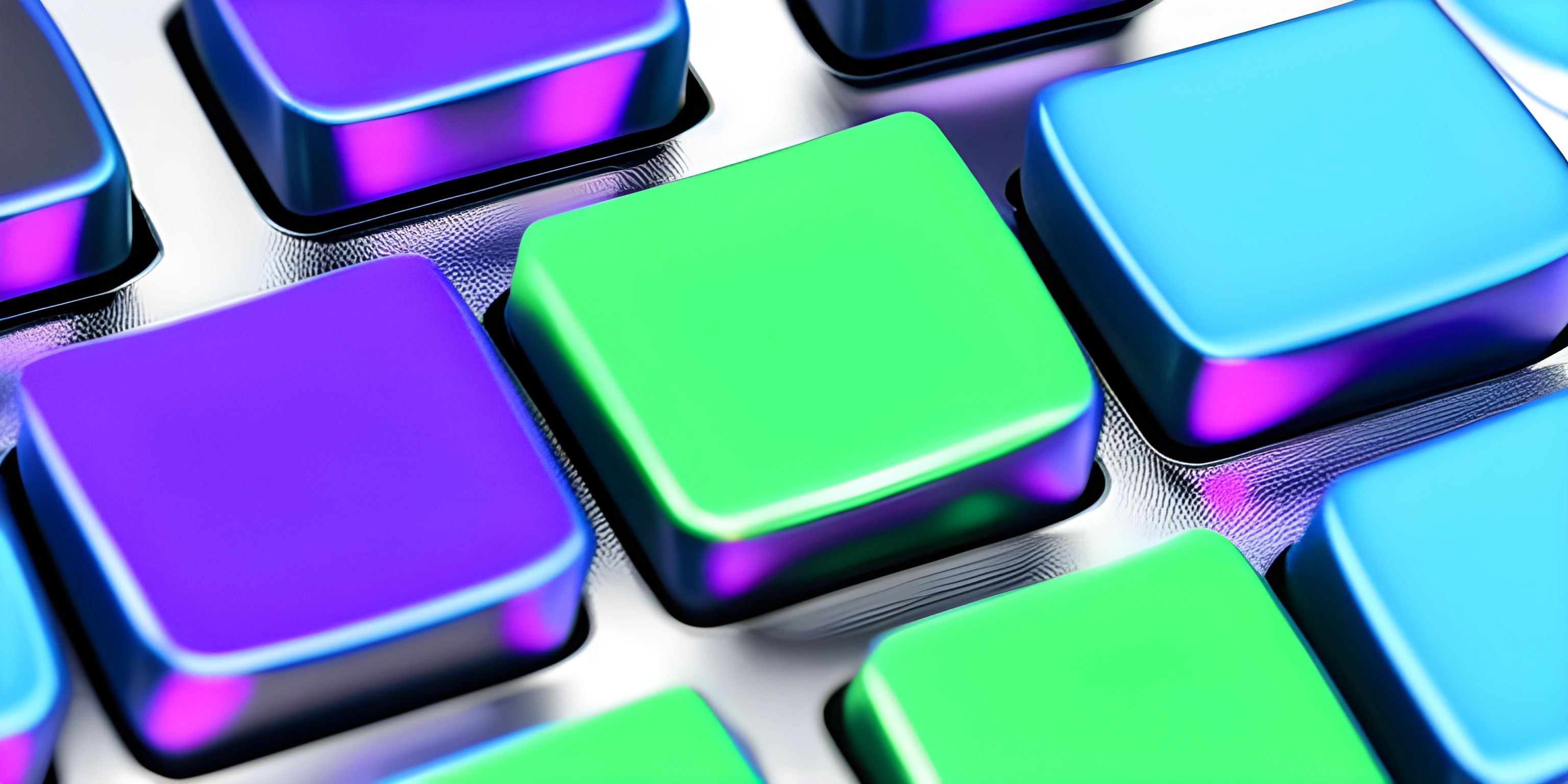
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
To truly understand the magic of manipulating text in programming, you must get to know regular expressions. Get excited, because this is like the 'Where's Waldo?' of the coding world, but way more fun!
What are Regular Expressions?
Regular expressions, often shortened to regex, are sequences of characters that form a search pattern. They are the bloodhounds of the code universe, seeking out specific bits of information in a text, file, or data stream. But unlike bloodhounds, regular expressions don't slobber!
If you're thinking, "Wow, that sounds useful!", then you're absolutely right. If you're thinking, "Wow, that sounds complex!", then you're also correct. But don't worry, we'll break it down.
Basic Syntax
Regular expressions come with their own syntax. Here's a simple example:
/^The/
In this case, the carat ^
indicates the start of a line. So, this regex will match any line that starts with "The". Simple enough, right?
Special Characters
In regex, certain characters have special meanings. The carat ^
is one example, but there are many others:
.
: Matches any single character, except for line breaks.*
: Matches zero or more of the preceding element.$
: Matches the end of a line.
Learning all these special characters is like learning a new language. But once you've mastered it, you'll be able to write powerful search patterns that can do much more than just find Waldo.
Uses of Regular Expressions
From validating user input to extracting parts of strings, regular expressions are used in many areas of programming. They are especially useful in scripting languages like JavaScript and Python.
For example, imagine you have a text file full of email addresses. You could use a regular expression to extract all the email addresses, or to check that they're valid.
Here's a simple regex that could be used to check if a string is a minimally valid email address:
/^[\w.-]+@[\w.-]+\.\w+$/
This checks that the string starts with one or more word characters, a dot or a dash, followed by an '@', followed by one or more word characters, a dot or a dash, followed by a dot, a word and the end of the line.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Enums (psst, it's free!).
FAQ
What are regular expressions?
Regular expressions, often shortened to regex, are sequences of characters that form a search pattern. They are used for finding specific bits of information in texts, files, or data streams.
What are some special characters in regular expressions?
Some special characters in regular expressions include the carat ^
which matches the start of a line, the period .
which matches any single character (except for line breaks), the asterisk *
which matches zero or more of the preceding element, and the dollar sign $
which matches the end of a line.
What are some uses of regular expressions?
Regular expressions are used in many areas of programming. They can validate user input, extract parts of strings, search for patterns in text, and much more. They are especially useful in scripting languages like JavaScript and Python.
How can regular expressions be used to validate email addresses?
A regular expression can be used to check if a string meets the basic criteria for an email address. For example, a simple regex to check for an email might look like this: ^[\w.-]+@[\w.-]+\.\w+$
. This checks that the string starts with one or more word characters, a dot, or a dash, followed by an '@', followed by one or more word characters, a dot, or a dash, followed by a dot, a word, and the end of the line.