Rust Enums
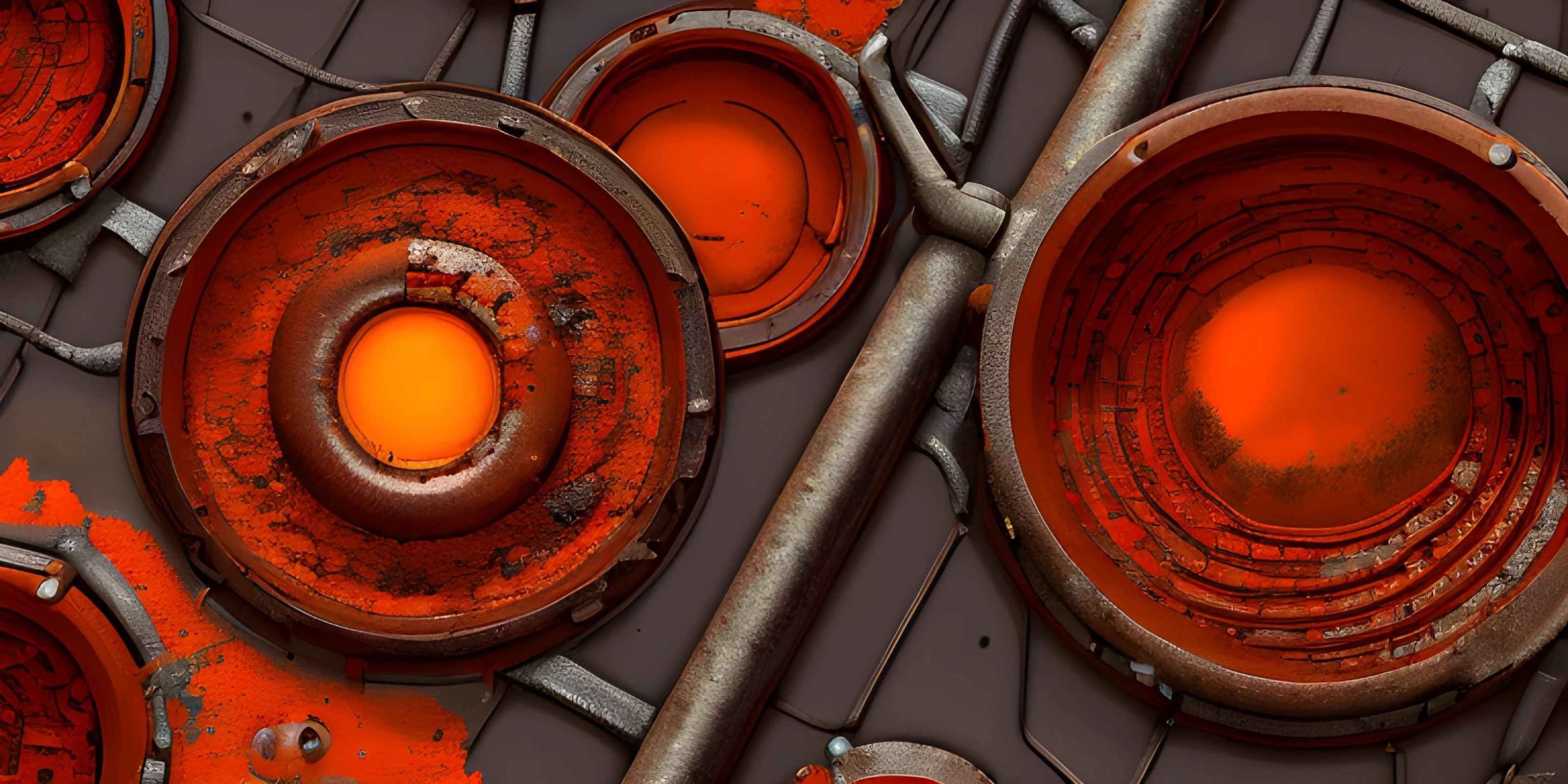
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Enums, short for enumerations, are a powerful feature in the Rust programming language. Enums provide a way to represent a value from a set of possible variants. Each variant in the set can hold different types and amounts of data. This allows for type-safe and expressive code, without having to rely on separate structs or loose constants.
Defining an Enum
To define an enum in Rust, use the enum
keyword followed by the name of the enum and a pair of curly braces {}
. Inside the braces, list the variants separated by commas. Let's create a simple example with an enum Animal
:
enum Animal { Dog, Cat, Fish, }
In this example, we have defined an Animal
enum with three possible variants: Dog
, Cat
, and Fish
. We can now use Animal
as a type in our code.
Creating Enum Instances
To create an instance of an enum, specify the enum name followed by the double colon ::
and the variant name. For example:
let my_pet = Animal::Dog;
Here, we create a variable my_pet
with the type Animal
and set it to the Dog
variant.
Enums with Data
One powerful feature of Rust enums is the ability to store data within the variants. Each variant can have different data types and structures. This is useful for representing complex data relationships. Here's an example of an enum with data:
enum Animal { Dog { breed: String, age: u32 }, Cat { name: String, is_outdoor: bool }, Fish { species: String }, }
In this version of the Animal
enum, each variant stores different data. The Dog
variant now contains a breed
and an age
, while the Cat
variant has a name
and a is_outdoor
flag. The Fish
variant only contains the species
.
Pattern Matching with Enums
To work with the data in enums, Rust provides a powerful feature called pattern matching. Pattern matching allows you to destructure and handle the different variants of an enum in a clean and concise way. We can use the match
keyword to perform pattern matching on an enum instance:
fn describe(animal: Animal) { match animal { Animal::Dog { breed, age } => println!("A {} dog, {} years old", breed, age), Animal::Cat { name, is_outdoor } => { let outdoor_status = if is_outdoor { "outdoor" } else { "indoor" }; println!("A {} cat named {}", outdoor_status, name); } Animal::Fish { species } => println!("A fish of species {}", species), } }
In the example above, we define a describe
function that takes an Animal
as an argument. Inside the function, we use a match
statement to destructure the enum and handle each variant separately. The output will vary based on the provided Animal
instance.
Conclusion
Rust enums are a versatile and expressive way to represent values with multiple variants. They provide type safety, pattern matching, and the ability to store data within each variant, making them an essential tool in any Rust programmer's toolkit. Don't be afraid to dive in and explore the wonderful world of enums!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Enums (psst, it's free!).
FAQ
What are Rust enums?
Enums, short for enumerations, are a powerful feature in Rust that allow you to define a type by enumerating its possible values. They provide better type safety and are more expressive compared to traditional integer-based enums. Enums can have associated data, and you can use pattern matching to handle different variants easily.
How do I define an enum in Rust?
To define an enum in Rust, use the enum
keyword followed by the name and a block containing the possible variants. Each variant can have associated data. For example:
enum Message { Quit, Move { x: i32, y: i32 }, Write(String), ChangeColor(u8, u8, u8), }
How can I use pattern matching with Rust enums?
Pattern matching is a powerful feature in Rust that allows you to destructure and handle different enum variants. You can use the match
expression to perform pattern matching on enums. Here's an example:
fn process_message(msg: Message) { match msg { Message::Quit => println!("Quit"), Message::Move { x, y } => println!("Move to ({}, {})", x, y), Message::Write(text) => println!("Write: {}", text), Message::ChangeColor(r, g, b) => println!("Change color to ({}, {}, {})", r, g, b), } }
What is the Option enum and how do I use it?
The Option
enum is a built-in Rust enum that represents optional values. It has two variants: Some
and None
. Some
contains a value, while None
represents the absence of a value. The Option
enum is commonly used in Rust to represent optional or nullable values without using null pointers. Here's an example:
fn find_user_by_id(id: u64) -> Option<String> { // Fetch user from the database if id == 42 { Some(String::from("Alice")) } else { None } }
How can I use the Result enum for error handling in Rust?
The Result
enum is another built-in Rust enum that represents the result of an operation that can either succeed or fail. It has two variants: Ok
and Err
. Ok
contains the successful result, while Err
contains an error value. The Result
enum is commonly used for error handling in Rust. Here's an example:
use std::fs::File; fn open_file(filename: &str) -> Result<File, std::io::Error> { File::open(filename) }