Understanding MVC Architecture
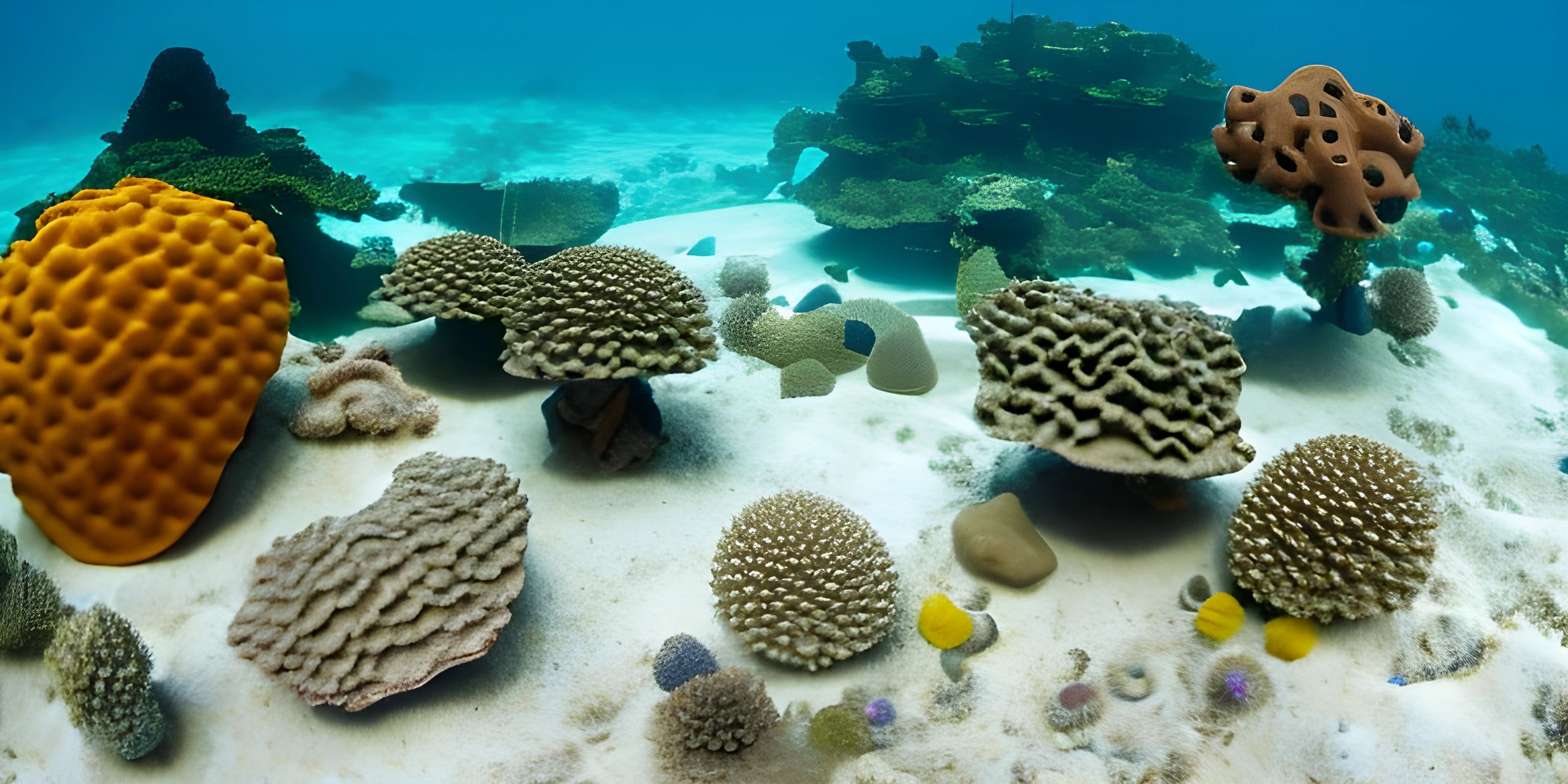
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
When it comes to organizing code for web applications, one term you'll often hear is "MVC." It sounds like a new brand of sneakers or maybe a secret government agency, but it actually stands for Model-View-Controller. This architectural pattern is a superhero in the world of software design, swooping in to save the day by keeping your code clean, modular, and maintainable.
Breaking Down MVC
The MVC architecture divides your application into three interconnected components. Each section has a distinct role, allowing developers to manage complexity and promote a clear separation of concerns.
Model
Think of the Model as the brain of your application. It's the part that thinks, remembers, and processes data. The Model represents the data and the business logic of your application. It's like the backstage crew in a theater production—unseen by the audience but pivotal for the show.
Let's look at a simple example in JavaScript:
class UserModel { constructor() { this.users = []; // An array to store users } addUser(user) { this.users.push(user); // Add a user to the array } getUsers() { return this.users; // Retrieve all users } }
View
If the Model is the brain, the View is the face. The View is responsible for what the user sees and interacts with. It's the user interface of your application—the HTML, CSS, and JavaScript that create the visual and interactive parts.
Here's a simple HTML snippet that could be part of a View:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>User List</title> </head> <body> <h1>Users</h1> <ul id="userList"></ul> <script src="view.js"></script> </body> </html>
And to dynamically update the View using JavaScript:
function renderUsers(users) { const userList = document.getElementById("userList"); userList.innerHTML = ""; // Clear the list users.forEach(user => { const li = document.createElement("li"); li.textContent = user.name; userList.appendChild(li); }); }
Controller
The Controller is the mediator, the middleman, the one who keeps everything running smoothly. It's the part that listens to user input, processes it (often consulting the Model), and updates the View accordingly. Think of the Controller as the director of the play, ensuring that the actors (Model and View) perform seamlessly together.
Here's a simple Controller in JavaScript:
class UserController { constructor(model, view) { this.model = model; this.view = view; this.initialize(); } initialize() { // Initial rendering of users this.view.renderUsers(this.model.getUsers()); // Example: Adding a user and re-rendering this.model.addUser({ name: "Alice" }); this.view.renderUsers(this.model.getUsers()); } } // Initialize the components const model = new UserModel(); const view = { renderUsers }; const controller = new UserController(model, view);
Benefits of MVC
The separation of concerns in MVC brings several advantages:
- Organized Code: Each component has a clear role, making your code easier to manage and understand.
- Reusability: Components can be reused across different parts of the application.
- Parallel Development: Different team members can work on Models, Views, and Controllers simultaneously without stepping on each other's toes.
- Testability: Isolated components mean you can test each part individually, ensuring robustness.
Real-World Applications
MVC is used extensively in web development frameworks. For instance, Ruby on Rails, Django, and ASP.NET all leverage MVC principles to help developers create robust and scalable web applications.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Full-stack Web Frameworks (Next.js) (psst, it's free!).
FAQ
What is the main purpose of the MVC architecture?
MVC architecture is designed to separate concerns within an application, specifically dividing it into three interconnected components: Model, View, and Controller. This separation makes code more organized, maintainable, and scalable.
Can you use MVC with any programming language?
Yes, MVC is a design pattern and can be implemented in virtually any programming language. Common languages that use MVC include JavaScript, Python, Ruby, and C#.
How does MVC improve testability?
MVC improves testability by isolating different parts of the application. Since Models, Views, and Controllers are separate, you can test each component independently, ensuring that each part functions correctly without interference from others.
Are there any drawbacks to using MVC?
While MVC has many benefits, it can add some complexity and overhead, particularly for smaller applications. Additionally, it might require a steeper learning curve for beginners who are new to the design pattern.
What is the role of the Controller in MVC?
The Controller acts as the intermediary between the Model and the View. It processes user input, interacts with the Model to fetch or update data, and subsequently updates the View to reflect any changes.