Rust Functions: Understanding and Using Them
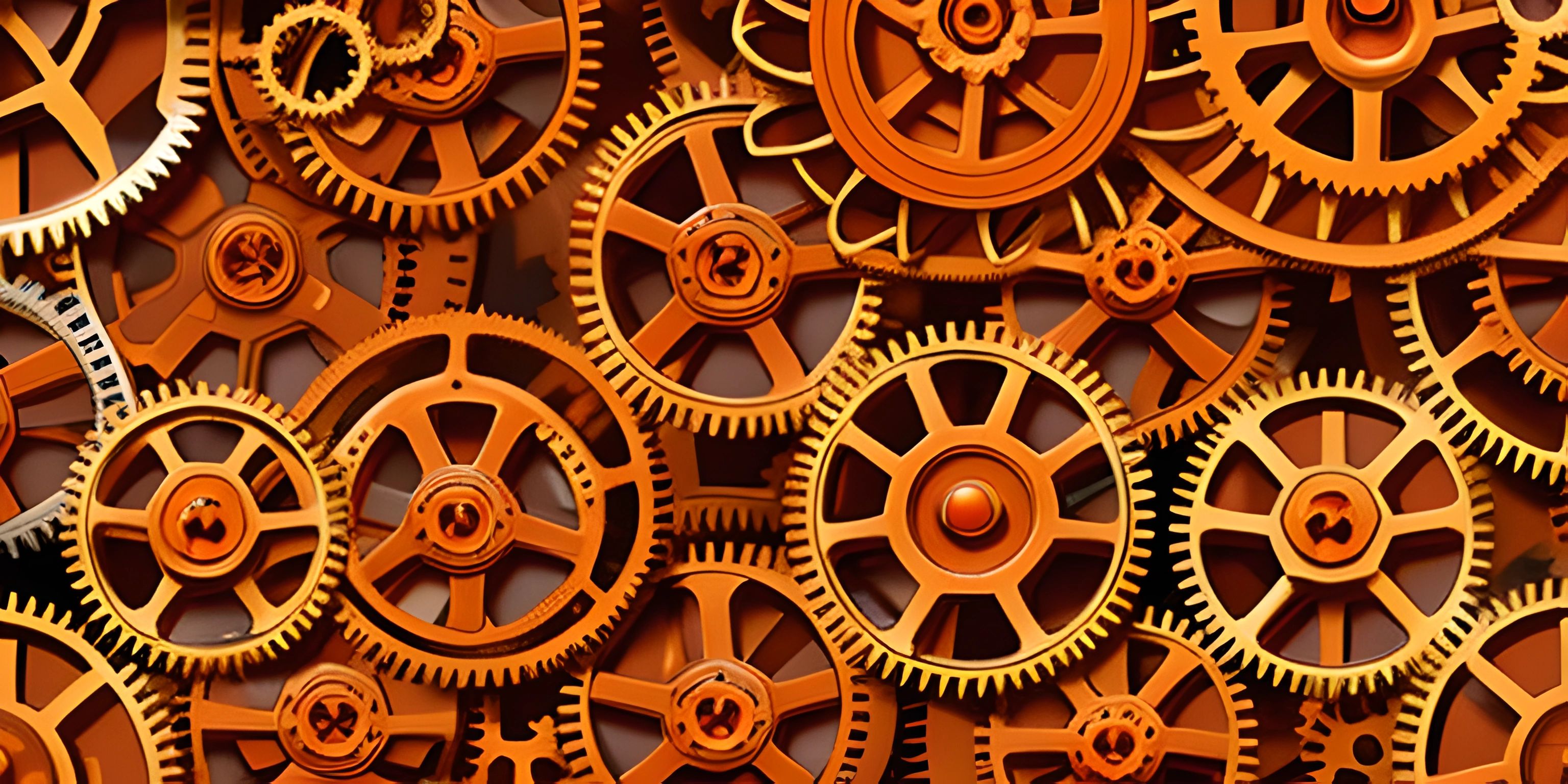
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
One of Rust's core concepts is the function, a building block that lets you organize and reuse your code. Functions in Rust follow a few simple rules, making them easy to create and understand. Let's jump in and learn the basics of Rust functions, how to write them, and how to use them effectively.
What is a Function?
In Rust, a function is a named sequence of statements that takes a set of input values, performs some operations, and returns an output value. Functions provide a way to break up your code into reusable, modular components. They're similar to methods in other programming languages.
Defining a Function in Rust
In Rust, you create a function using the fn
keyword, followed by the function name, a parenthesized list of input parameters, a return type (optional), and a code block. Here's a simple example of a Rust function that takes two integers as input and returns their sum:
fn add(a: i32, b: i32) -> i32 { a + b }
This function is called add
, and it takes two parameters, a
and b
, both of type i32
. The return type is also i32
. The function body consists of a single expression, a + b
, which is the value that gets returned.
Calling a Function
To call a function in Rust, you simply write the function name followed by a parenthesized list of arguments. Here's an example of how to call our add
function:
fn main() { let sum = add(3, 5); println!("The sum is: {}", sum); }
This program defines a main
function, which is the entry point of every Rust program. Inside main
, we call the add
function with the arguments 3
and 5
and store the result in a variable called sum
. We then use the println!
macro to print the sum.
Functions with No Return Value
In Rust, functions that don't explicitly return a value are considered to return the unit type ()
. This is similar to void
in languages like C and Java. Here's an example of a function that doesn't return anything and just prints a message:
fn greet() { println!("Hello, Rustacean!"); } fn main() { greet(); }
The greet
function takes no parameters and has no return type specified. It simply prints a greeting message. In the main
function, we call the greet
function to display the message.
Function Ownership and Borrowing
In Rust, functions also interact with the ownership and borrowing system. When you pass a value to a function, the ownership of that value is transferred to the function. Similarly, when a function returns a value, ownership is transferred back to the caller.
Here's an example that demonstrates ownership transfer between functions:
fn consume_string(s: String) { println!("Consumed: {}", s); } fn main() { let my_string = String::from("Hello, Rustacean!"); consume_string(my_string); // The next line would produce an error, because `my_string` is no longer valid // println!("Trying to print my_string: {}", my_string); }
In this example, consume_string
takes a String
as a parameter. When we call this function in main
, the ownership of my_string
is transferred to consume_string
. After the function call, my_string
is no longer valid, and trying to use it again would result in a compile-time error.
To avoid transferring ownership in a function, you can use borrowing by passing a reference to the value instead. For more information on borrowing, check out the Rust borrowing article.
Now that you have a solid grasp of Rust functions, you can start building modular and reusable Rust programs. Don't forget to explore other Rust concepts like pattern matching and error handling to further improve your Rust-fu!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Syntax (psst, it's free!).
FAQ
What is a Rust function and why do we use them?
A Rust function is a named sequence of statements that takes a specific set of inputs, performs a task, and returns a value. Functions are essential building blocks in Rust, allowing you to write modular, reusable, and maintainable code. By using functions, you can break down complex tasks into smaller, more manageable pieces, which makes your code easier to understand and debug.
How do I define a function in Rust?
To define a function in Rust, you use the fn
keyword followed by the function name, a parenthesized list of input parameters, a return type (if the function returns a value), and a block of code. Here's a simple example:
fn greet(name: &str) { println!("Hello, {}!", name); }
In this example, we define a function called greet
that takes a single input parameter name
of type &str
(a string slice) and has no return value (as indicated by the absence of an arrow ->
and a return type).
How do I call a function in Rust?
To call a function in Rust, you simply use its name followed by a parenthesized list of argument values that match the function's input parameters. Here's an example of how to call the greet
function defined earlier:
fn main() { greet("Alice"); }
In this example, we call the greet
function with the argument "Alice" within the main
function, which is the entry point of a Rust program.
How do I return a value from a Rust function?
To return a value from a Rust function, you need to specify the return type using the arrow ->
followed by the type, and then use the return
keyword followed by the value you want to return. Alternatively, you can omit the return
keyword and end the function with an expression that evaluates to the return value. Here's an example:
fn add(a: i32, b: i32) -> i32 { a + b }
In this example, we define a function called add
that takes two input parameters a
and b
of type i32
(32-bit integers) and returns a value of the same type. The function simply adds the two input values and returns the result.
How can I use functions to create more modular and maintainable code in Rust?
By breaking down complex tasks into smaller functions, you can make your Rust code more modular and maintainable. Each function should have a single, well-defined purpose, making it easier to understand, test, and debug. You can also reuse functions in multiple places within your code, which helps to reduce duplication and improve code maintainability. Here's an example of using functions to create a more modular Rust program:
fn main() { let result = add(5, 7); print_result(result); } fn add(a: i32, b: i32) -> i32 { a + b } fn print_result(result: i32) { println!("The result is: {}", result); }
In this example, we define two functions, add
and print_result
, each with a specific purpose. The main
function then calls these functions to perform the desired task, making the code more modular and easier to understand.