Understanding Recursive Functions
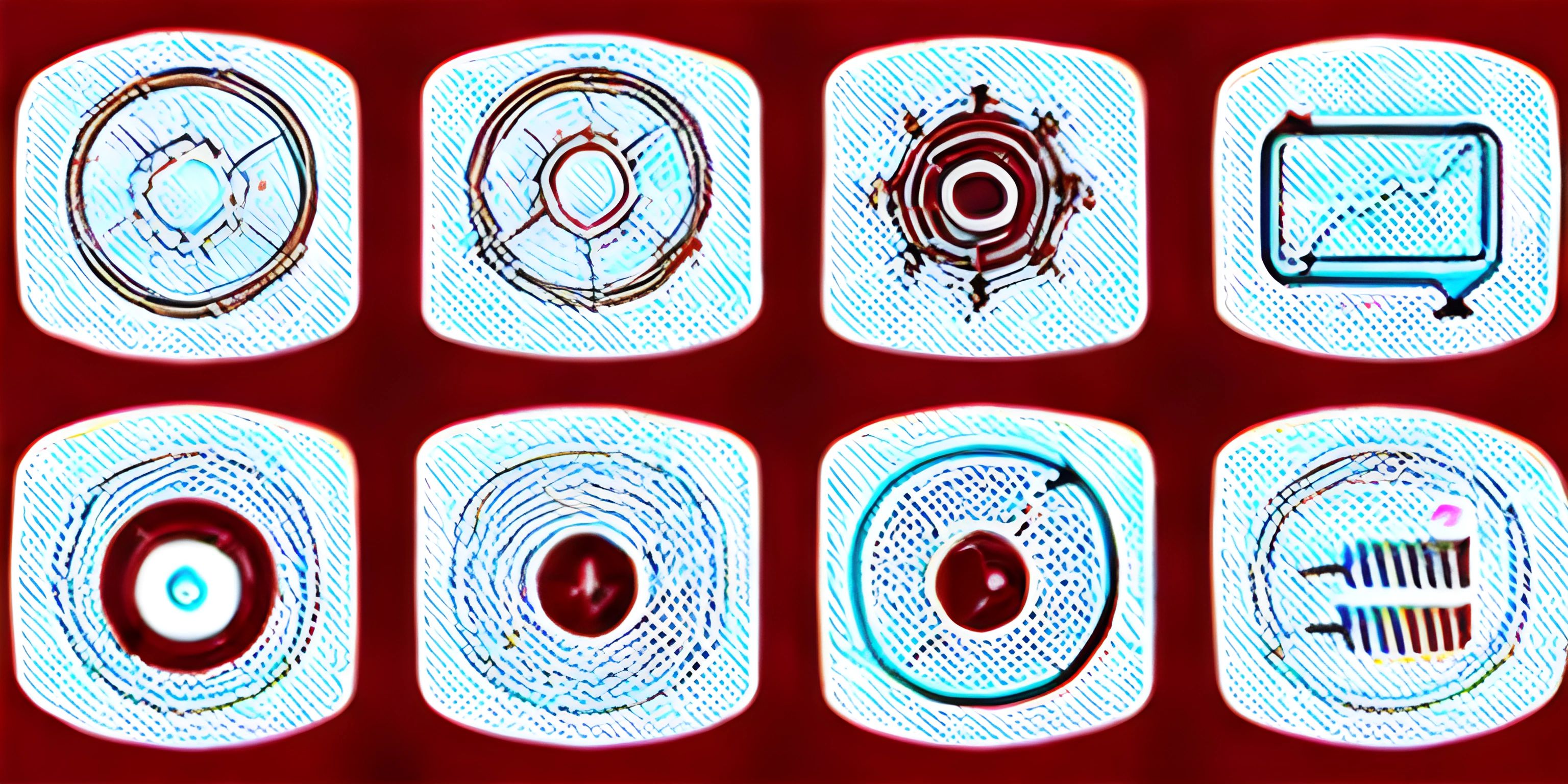
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Have you ever seen an image of a person holding a picture of themselves holding a picture, and so on? That's recursion in a nutshell—something that refers to itself. In programming, recursive functions are functions that call themselves to solve a problem. But why would you want a function to be so self-obsessed?
What is Recursion?
A recursive function is like a matryoshka doll. It opens itself, revealing a smaller version inside, which again reveals an even smaller version, and so forth, until the smallest doll is reached. Each call to the function peels away a layer, working towards a base case that stops the recursion.
Let's consider an example in Python, where recursion is frequently used.
Factorial Example
Calculating the factorial of a number is a classic example of recursion. The factorial of n
(denoted as n!
) is the product of all positive integers less than or equal to n
.
Here’s how you can compute it recursively:
def factorial(n): # Base case: if n is 1, the factorial is 1 if n == 1: return 1 # Recursive case: n * factorial of (n-1) return n * factorial(n - 1) print(factorial(5)) # Output will be 120
In this example, the function factorial
calls itself with n-1
until it reaches the base case where n
is 1. At that point, it returns 1, and all the stacked calls resolve, multiplying their results as they "unwind" back up the call stack.
When to Use Recursion
Recursion is particularly useful for problems that can be broken down into smaller, similar subproblems. Some common scenarios include:
- Tree Traversal: Navigating hierarchical structures like file systems or organizational charts.
- Divide and Conquer Algorithms: Algorithms like quicksort and mergesort.
- Dynamic Programming: Problems like the Fibonacci sequence and combinatorial problems.
Tree Traversal Example
Traversing a binary tree is a classic use of recursion. Here's how you might implement an in-order traversal in Python:
class Node: def __init__(self, data): self.left = None self.right = None self.data = data def in_order_traversal(root): if root: # Traverse left subtree in_order_traversal(root.left) # Visit the root node print(root.data) # Traverse right subtree in_order_traversal(root.right) # Example usage root = Node(1) root.left = Node(2) root.right = Node(3) root.left.left = Node(4) root.left.right = Node(5) in_order_traversal(root) # Output will be 4 2 5 1 3
In this example, the in_order_traversal
function calls itself to visit the left subtree, the root, and then the right subtree.
The Importance of Base Cases
One of the most crucial aspects of a recursive function is the base case. This is the condition under which the function stops calling itself, preventing an infinite loop and eventually causing a stack overflow error. Think of the base case as the "exit" sign in a recursive maze.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Recursion Intro (psst, it's free!).
FAQ
What is a base case in recursion?
A base case is a condition that stops the recursion. It is essential to prevent infinite loops and stack overflow errors. Every recursive function must have at least one base case.
Can recursion be substituted with loops?
Yes, many problems that are solved using recursion can also be solved using loops. However, recursion can simplify the code for problems that have a natural recursive structure, like tree traversal.
What are the downsides of using recursion?
Recursion can lead to performance issues like stack overflow or increased memory usage due to the call stack. It can also be slower compared to iterative solutions because of the overhead of repeated function calls.
How can I debug a recursive function?
Debugging a recursive function can be challenging. Use print statements to trace the function calls and understand the flow. Tools like debuggers can also help by allowing you to step through each recursive call.
Are there any languages better suited for recursion?
Functional programming languages like Haskell and Lisp are particularly well-suited for recursion because they optimize recursive calls. However, most modern languages, including Python, JavaScript, and C++, support recursion effectively.