Exploring Different Searching Algorithms
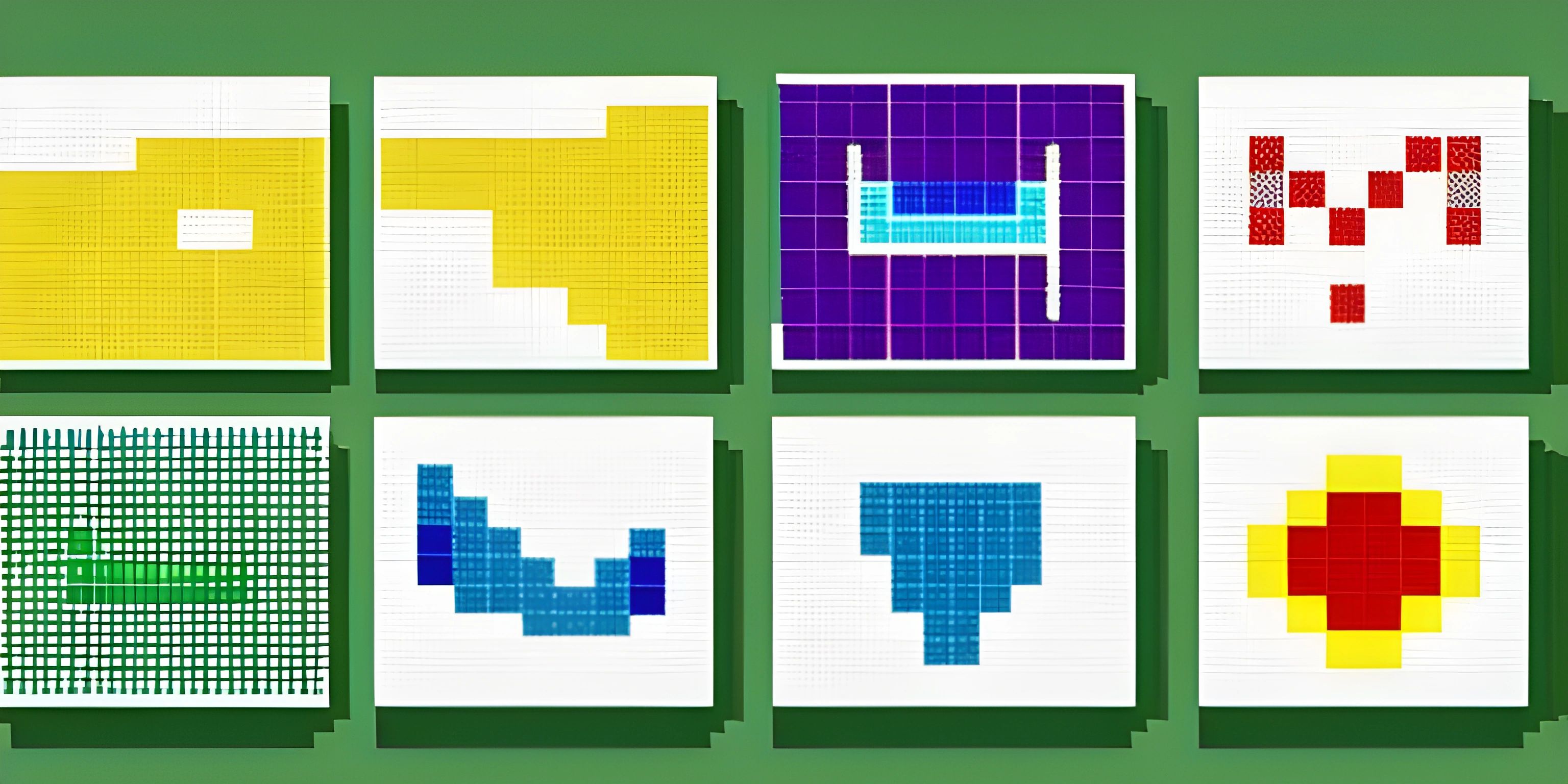
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Searching for a needle in a haystack might sound tedious, but with the right techniques, you can make it a breeze. Whether you're hunting through a list of names or a vast database, knowing how to efficiently find what you're looking for is invaluable. Let's embark on an adventure to explore some of the most common searching algorithms and see how they can make your life easier.
What is a Searching Algorithm?
A searching algorithm is a method used to find a specific element within a data structure. Imagine you have a library of books, and you want to find "Harry Potter and the Philosopher's Stone." You could either start from the beginning and check each book one by one, or you could use a more sophisticated approach if the books are sorted.
Linear Search
Linear search, also known as sequential search, is the simplest searching algorithm. It checks each element in the data structure one by one until it finds the target element or reaches the end.
Here's how it works in JavaScript:
function linearSearch(arr, target) { // Iterate through each element in the array for (let i = 0; i < arr.length; i++) { // Check if the current element matches the target if (arr[i] === target) { return i; // Return the index of the target element } } return -1; // Return -1 if the target element is not found } const array = [10, 20, 30, 40, 50]; const targetElement = 30; const result = linearSearch(array, targetElement); console.log(result); // Output: 2
Linear search is straightforward but can be inefficient for large datasets because it might have to check every single element.
Binary Search
Binary search is a more efficient algorithm but requires that the data be sorted. This algorithm repeatedly divides the search interval in half. If the value of the target element is less than the value in the middle of the interval, the algorithm narrows the interval to the lower half. Otherwise, it narrows it to the upper half. This process continues until the target element is found or the interval is empty.
Here's how binary search works in Python:
def binary_search(arr, target): left, right = 0, len(arr) - 1 while left <= right: mid = (left + right) // 2 # Find the middle index if arr[mid] == target: return mid # Target element found elif arr[mid] < target: left = mid + 1 # Narrow to the upper half else: right = mid - 1 # Narrow to the lower half return -1 # Target element not found array = [10, 20, 30, 40, 50] target_element = 30 result = binary_search(array, target_element) print(result) # Output: 2
Binary search is much faster than linear search for large datasets, with a time complexity of O(log n) compared to O(n) for linear search.
Hashing
Hashing is an algorithm that transforms a given input into a fixed-size string of characters, which is usually a hash code. The main advantage of hashing is its efficiency in search operations, usually averaging O(1) time complexity. Think of it as a magical spell that directly points to the item you're looking for.
Here's a simple hashing example in Java:
import java.util.HashMap; public class HashingSearch { public static void main(String[] args) { HashMap<Integer, String> dataMap = new HashMap<>(); dataMap.put(1, "Apple"); dataMap.put(2, "Banana"); dataMap.put(3, "Cherry"); int key = 2; if (dataMap.containsKey(key)) { System.out.println("Found: " + dataMap.get(key)); // Output: Found: Banana } else { System.out.println("Not Found"); } } }
Hashing is particularly powerful when dealing with large datasets, like dictionaries and databases, making retrieval operations lightning-fast.
Depth-First Search (DFS)
DFS is a graph traversal algorithm that starts at the root node and explores as far as possible along each branch before backtracking. It's like exploring a labyrinth by always going as far as you can before hitting a dead end and then backtracking.
Here's how DFS can be implemented in C++:
#include <iostream> #include <vector> using namespace std; void DFSUtil(int v, vector<bool>& visited, const vector<vector<int>>& adj) { visited[v] = true; cout << v << " "; // Print the visited node // Recur for all the vertices adjacent to this vertex for (int i : adj[v]) { if (!visited[i]) { DFSUtil(i, visited, adj); } } } void DFS(const vector<vector<int>>& adj, int V) { vector<bool> visited(V, false); // Mark all vertices as not visited for (int v = 0; v < V; v++) { if (!visited[v]) { DFSUtil(v, visited, adj); } } } int main() { vector<vector<int>> adj = { {1, 2}, {0, 3, 4}, {0, 4}, {1, 5}, {1, 2, 5}, {3, 4} }; int V = adj.size(); // Number of vertices cout << "Depth-First Search starting from node 0:\n"; DFS(adj, V); // Perform DFS starting from node 0 return 0; }
DFS is useful for tasks that involve exploring all possible paths, such as solving puzzles and finding connected components in a graph.
Breadth-First Search (BFS)
BFS is another graph traversal algorithm that starts at the root node and explores all its neighbors before moving to the next level. Imagine standing at the center of a maze and exploring all possible moves at each step.
Here's how BFS can be implemented in Python:
from collections import deque def bfs(graph, start): visited = set() queue = deque([start]) visited.add(start) while queue: vertex = queue.popleft() print(vertex, end=" ") # Print the visited node for neighbor in graph[vertex]: if neighbor not in visited: visited.add(neighbor) queue.append(neighbor) graph = { 0: [1, 2], 1: [0, 3, 4], 2: [0, 4], 3: [1, 5], 4: [1, 2, 5], 5: [3, 4] } print("Breadth-First Search starting from node 0:") bfs(graph, 0) # Output: 0 1 2 3 4 5
BFS is ideal for finding the shortest path in unweighted graphs and exploring nodes layer by layer.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Data Types (psst, it's free!).
FAQ
What is the main difference between linear search and binary search?
Linear search checks each element one by one and is simpler but less efficient for large datasets. Binary search, on the other hand, requires the dataset to be sorted and repeatedly divides the interval in half, making it much faster with a time complexity of O(log n).
When should I use hashing for searching?
Hashing is best used when you need fast retrieval times and can afford the overhead of creating a hash table. It's particularly effective for large datasets where quick lookups are essential, such as dictionaries and databases.
What are some applications of Depth-First Search (DFS)?
DFS is used in various applications such as solving puzzles, finding connected components in a graph, performing topological sorting, and detecting cycles in a graph.
How does Breadth-First Search (BFS) differ from Depth-First Search (DFS)?
BFS explores all nodes at the present depth level before moving on to nodes at the next depth level, making it ideal for finding the shortest path in unweighted graphs. DFS, in contrast, explores as far as possible along each branch before backtracking, making it suitable for tasks like maze solving and pathfinding.
Can binary search be used on unsorted data?
No, binary search requires the data to be sorted in order to function correctly. If the data is not sorted, you would need to sort it first or use a different searching algorithm like linear search.