SQL Database Concepts
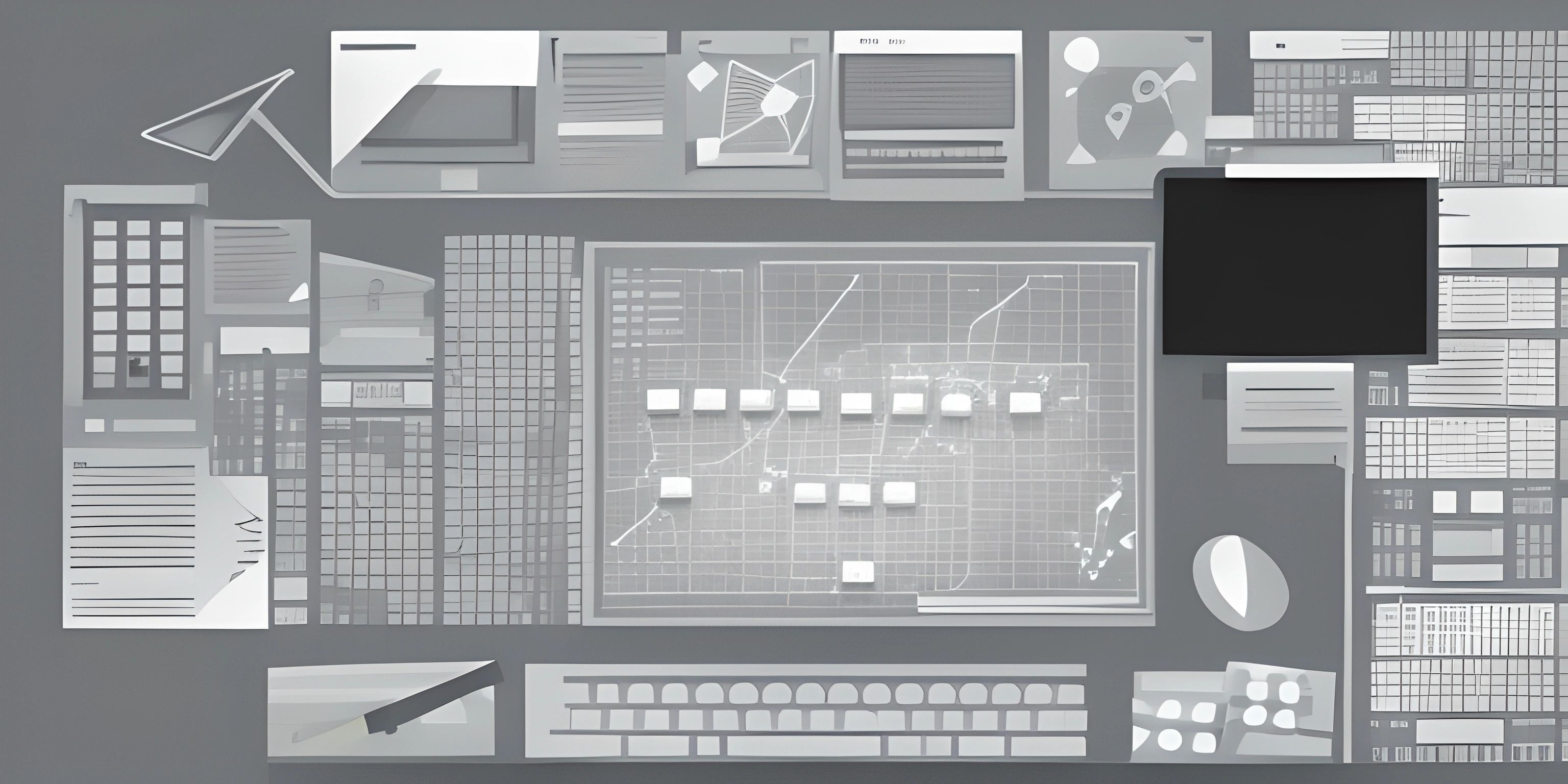
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Diving into the world of databases can be both exhilarating and intimidating, but fear not! We're here to guide you through the maze of SQL database concepts, and soon you'll be querying like a pro. Let's begin with the building blocks of SQL databases: tables, queries, and relationships.
Tables
Think of a table as the foundation of your SQL database. It's a grid-like structure that stores data in rows and columns. Each row represents a separate record, and each column represents a specific attribute of that record. For example, let's imagine we have a table called employees
:
| id | first_name | last_name | position | |----|------------|-----------|--------------| | 1 | Alice | Smith | Developer | | 2 | Bob | Johnson | Designer | | 3 | Carol | Williams | Project Lead |
Here, we have a simple employees
table with three columns (id
, first_name
, and last_name
) and three rows representing different employees.
Queries
In SQL, we use queries to interact with our database. The most common operations are selecting, inserting, updating, and deleting data. Let's explore each one briefly:
SELECT
The SELECT
statement retrieves data from one or more tables. For example, if we want to fetch all the data from the employees
table, we'd write:
SELECT * FROM employees;
*
represents all columns. If you want to select specific columns, you can list them like so:
SELECT first_name, last_name FROM employees;
INSERT
The INSERT
statement adds new records to a table. To add a new employee to the employees
table, we'd write:
INSERT INTO employees (id, first_name, last_name, position) VALUES (4, 'David', 'Brown', 'Tester');
UPDATE
The UPDATE
statement modifies existing records in a table. If we want to change David's position to 'QA Engineer', we'd write:
UPDATE employees SET position = 'QA Engineer' WHERE id = 4;
DELETE
The DELETE
statement removes records from a table. To remove David from the employees
table:
DELETE FROM employees WHERE id = 4;
Relationships
In an SQL database, tables often have relationships with one another. There are three main types of relationships: one-to-one, one-to-many, and many-to-many.
One-to-One
A one-to-one relationship signifies that each record in table A is linked to one and only one record in table B. For example, imagine an employee_details
table containing information that only pertains to a single employee.
One-to-Many
A one-to-many relationship signifies that each record in table A is linked to multiple records in table B. Consider a projects
table, where each project has multiple employees assigned to it, but an employee can only be assigned to one project at a time.
Many-to-Many
A many-to-many relationship signifies that each record in table A is linked to multiple records in table B, and vice versa. To represent this relationship, we use a junction table. For instance, an employee_skills
table might link employees to their various skills, allowing employees to have multiple skills and each skill to be possessed by multiple employees.
And there you have it! With a basic understanding of tables, queries, and relationships, you're well on your way to conquering the world of SQL databases. Keep practicing, and soon you'll be constructing complex queries and designing intricate databases with ease.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Basic Concepts (psst, it's free!).
FAQ
What are the basic SQL database concepts that beginners should know?
The basic SQL database concepts that beginners should know are:
- Tables: A table is the primary unit of data storage in an SQL database, consisting of rows and columns.
- Queries: Queries are commands used to retrieve, add, modify, or delete data within the database.
- Relationships: Relationships are the connections between tables to establish how data is related.
How do tables work in an SQL database?
Tables in an SQL database are structured in rows and columns, similar to a spreadsheet. Each row represents a record, and each column represents an attribute of that record. A table is defined by a schema, which specifies the columns and their data types, constraints, and other properties.
What is the purpose of SQL queries?
SQL queries are used to interact with the data stored in an SQL database. They serve various purposes such as:
- Retrieving data from one or more tables (SELECT)
- Inserting new rows (INSERT)
- Updating existing rows (UPDATE)
- Deleting rows (DELETE)
- Creating, modifying, or deleting tables and other database objects (CREATE, ALTER, DROP)
Can you give an example of a simple SQL query?
Here's an example of a simple SQL query to retrieve all columns and rows from a table called "employees":
SELECT * FROM employees;
What are the different types of relationships in SQL databases?
There are three main types of relationships in SQL databases:
- One-to-One: Each record in Table A is related to exactly one record in Table B, and vice versa.
- One-to-Many: Each record in Table A is related to one or more records in Table B, but each record in Table B is related to only one record in Table A.
- Many-to-Many: Each record in Table A is related to one or more records in Table B, and each record in Table B is related to one or more records in Table A. This is typically implemented using a junction table.