SQL Introduction
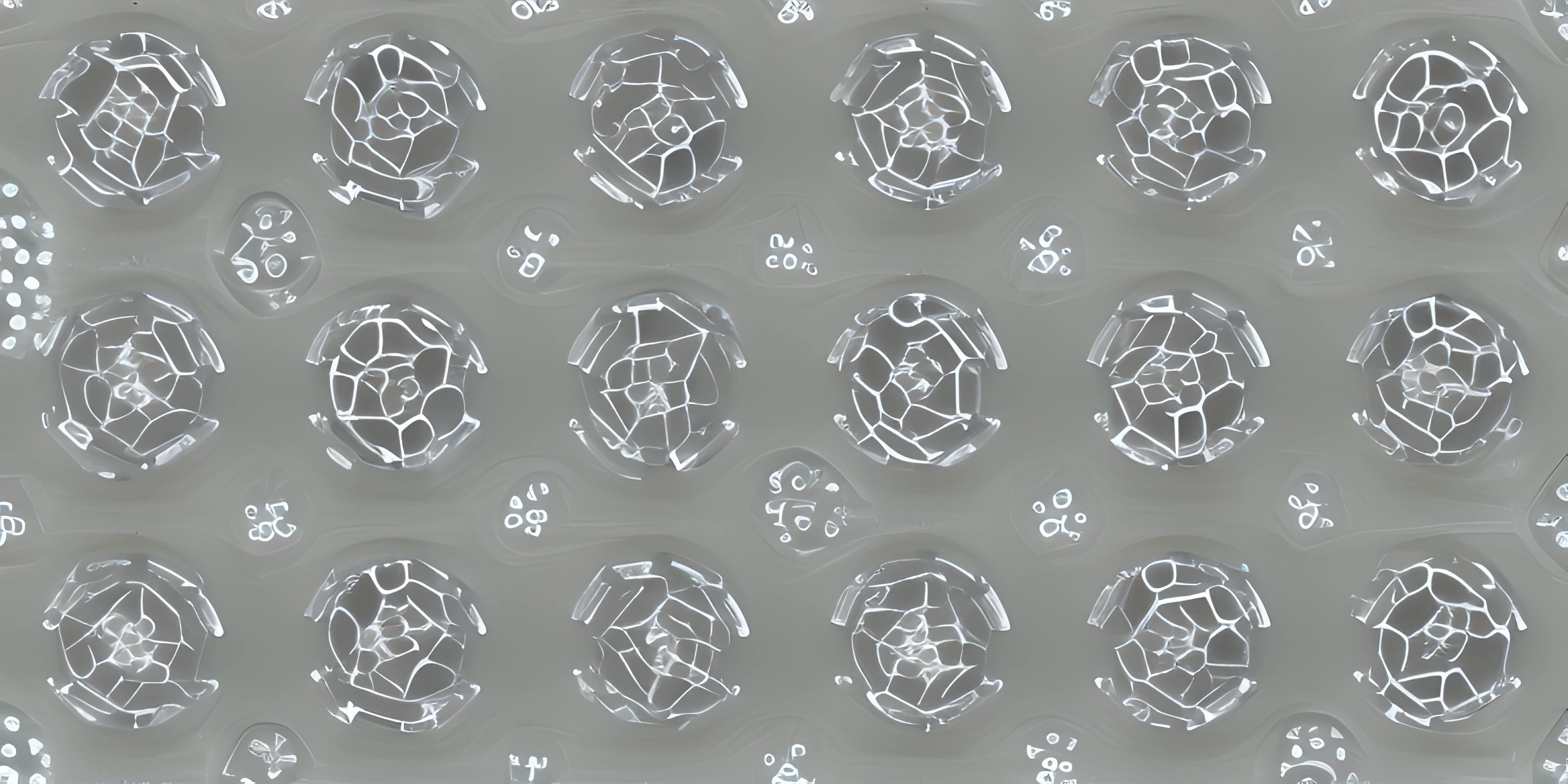
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Structured Query Language, or SQL for short, is the lingua franca for interacting with databases. It's a powerful, standardized tool that allows you to talk to your data, ask it questions, and make it dance like a well-trained ballerina. Let's dive in and get a taste of what SQL is all about.
A Peek into SQL
SQL is used to communicate with databases like MySQL, PostgreSQL, and Oracle. It's the go-to language for managing and manipulating data stored in relational databases. From simple data retrieval to complex data manipulation, SQL has got your back!
SQL Queries: The Key to the Kingdom
The heart of SQL lies in its queries. Queries are like a series of questions you ask your data to get the answers you need. Whether you want to fetch specific data, update it, or delete it, you'll find yourself writing SQL queries to accomplish the task.
Here's a simple example of an SQL query using the SELECT
statement to fetch data from a table called employees
:
SELECT first_name, last_name FROM employees;
This query retrieves the first name and last name of all employees in the employees
table.
CRUD Operations
SQL supports the four main CRUD operations: Create, Read, Update, and Delete. These operations are the bread and butter of managing your data. Let's take a quick look at each operation:
- Create: Use the
INSERT
statement to add new data to your database.
INSERT INTO employees (first_name, last_name) VALUES ("John", "Doe");
This statement inserts a new employee with the first name "John" and the last name "Doe" into the employees
table.
- Read: Use the
SELECT
statement to retrieve data from your database.
SELECT * FROM employees WHERE last_name = "Doe";
This statement fetches all records from the employees
table where the last name is "Doe".
- Update: Use the
UPDATE
statement to modify existing data in your database.
UPDATE employees SET first_name = "Jane" WHERE last_name = "Doe";
This statement updates the first name of all employees with the last name "Doe" to "Jane".
- Delete: Use the
DELETE
statement to remove data from your database.
DELETE FROM employees WHERE last_name = "Doe";
This statement deletes all records from the employees
table with the last name "Doe".
SQL: A Never-Ending Journey
So there you have it - a brief introduction to SQL and its capabilities. Of course, this is just the tip of the iceberg. SQL is a versatile and powerful language with a wide range of advanced features waiting for you to explore. As you dive deeper into the world of databases, you'll encounter exciting concepts like joins, subqueries, and stored procedures.
Now, it's time to roll up your sleeves, fire up your favorite SQL client, and start querying your data like a pro!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Async Rust (psst, it's free!).
FAQ
What is SQL and why is it important?
SQL (Structured Query Language) is a standardized programming language used to manage, manipulate, and query relational databases. It is important because it allows you to efficiently interact with large amounts of data, retrieve specific information, and perform various operations like inserting, updating, or deleting data.
Can you provide some examples of SQL statements?
Sure! Here are a few examples of common SQL statements:
-- SELECT: To retrieve data from a table SELECT * FROM employees; -- INSERT: To add new data to a table INSERT INTO employees (id, first_name, last_name, role) VALUES (1, "John", "Doe", "Manager"); -- UPDATE: To modify existing data in a table UPDATE employees SET role = "Supervisor" WHERE id = 1; -- DELETE: To remove data from a table DELETE FROM employees WHERE id = 1;
What are the different types of SQL commands?
SQL commands can be categorized into the following types:
- Data Definition Language (DDL): Used for defining the structure of a database, like creating or altering tables. Example commands: CREATE, ALTER, DROP.
- Data Manipulation Language (DML): Used for managing and manipulating data in a database. Example commands: SELECT, INSERT, UPDATE, DELETE.
- Data Control Language (DCL): Used for controlling access to a database. Example commands: GRANT, REVOKE.
- Transaction Control Language (TCL): Used for managing transactions within a database. Example commands: COMMIT, ROLLBACK, SAVEPOINT.
How can I filter and sort data using SQL?
To filter and sort data in SQL, you can use the WHERE
and ORDER BY
clauses, respectively. Here are some examples:
-- Filtering data using the WHERE clause SELECT * FROM employees WHERE role = "Manager"; -- Sorting data using the ORDER BY clause SELECT * FROM employees ORDER BY last_name ASC; -- Combining both filtering and sorting SELECT * FROM employees WHERE role = "Manager" ORDER BY last_name DESC;