Standard Library Basics
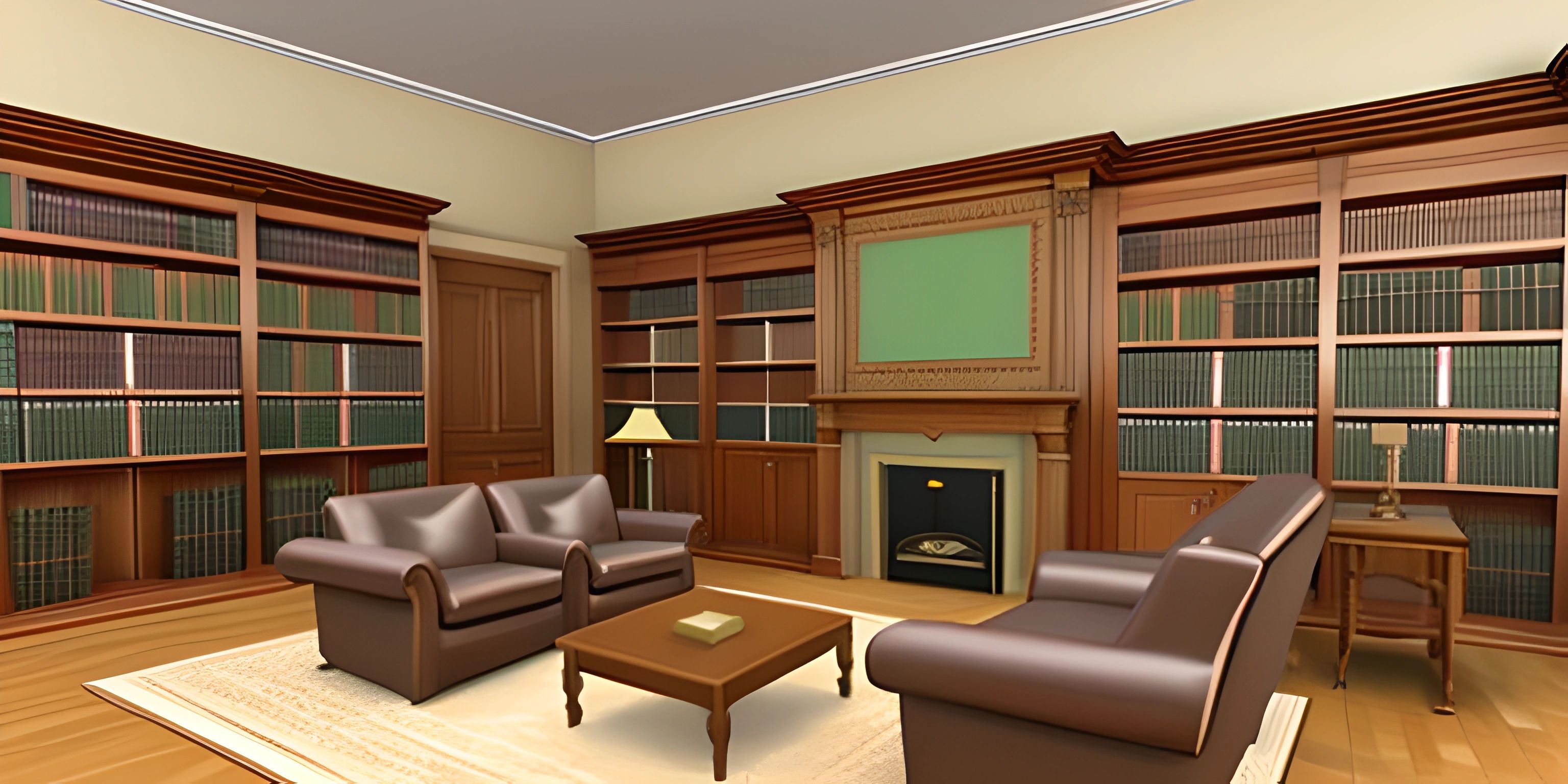
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Diving into programming can feel like trying to make a delicious homemade meal from scratch, without any ingredients in your pantry. Thankfully, every programming language comes stocked with a standard library that provides you with a set of pre-written code ingredients, ready for use in your programs.
What is a Standard Library?
A standard library is a collection of functions, classes, and modules that come bundled with a programming language. These pre-written pieces of code help you accomplish common tasks without having to reinvent the wheel. It's like a toolset that comes with your shiny new programming language, saving you time and effort.
For example, if you need to sort a list of numbers, you don't have to write your own sorting algorithm. You can use a function from your language's standard library that does the job for you. Most standard libraries provide functionality for things like file I/O, string manipulation, and mathematical operations.
Why is a Standard Library Important?
Picture yourself stranded on a deserted island. You'd need to build a shelter, find food, and create a signal for help. Imagine how much easier your life would be if you had a toolbox with tools and instructions to help you survive! Standard libraries are like that toolbox for programmers.
Here are a few reasons why standard libraries are important:
-
Efficiency: A standard library offers pre-written code, allowing you to focus on the unique aspects of your project. You'll spend less time writing and debugging basic functionality.
-
Consistency: Using a standard library promotes consistency across different projects and developers. It encourages everyone to use the same set of tools, making it easier to read, understand, and maintain code.
-
Reliability: Standard libraries are usually well-tested and optimized for performance. Instead of rolling your custom solution, you can rely on a battle-tested implementation provided by the language creators.
-
Portability: When you use functions from a standard library, your code becomes more portable across different platforms. The library takes care of platform-specific details, so you don't have to.
A Glimpse into Different Standard Libraries
Let's take a quick tour of some standard libraries from popular programming languages:
-
Python: Python's standard library, also known as "batteries included," is extensive and covers a wide range of functionality. Modules like
os
,sys
,re
, andmath
are commonly used by developers. You can check the Python documentation for more information. -
JavaScript: JavaScript's standard library is relatively small compared to Python, but it still covers the essentials. You'll find built-in objects like
Array
,Math
,Date
, andString
that offer functions and methods for various tasks. You can find more details in the MDN Web Docs. -
Java: Java's standard library, also known as the Java API, is vast and covers everything from basic data structures to network communication. Packages like
java.util
,java.io
, andjava.math
are frequently used. Check the Java API documentation for more information.
Conclusion
A standard library is your programming language's best friend, providing you with pre-written code to accomplish common tasks. It's essential for efficiency, consistency, reliability, and portability in your projects. So, the next time you start a new project, remember to explore your language's standard library - it's like opening up a treasure chest of ready-to-use tools!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Upgrading our Calculator (psst, it's free!).
FAQ
What is a standard library in programming?
A standard library is a collection of pre-written code that comes with a programming language. It provides commonly used functions, data structures, and utility modules that developers can use to build their applications more efficiently. By using the standard library, programmers can save time and effort, as well as maintain consistency and readability in their code.
Why are standard libraries important?
Standard libraries are important for several reasons:
- They provide reusable code for common programming tasks, which can save time and effort for developers.
- Using the standard library ensures consistency and readability in your code, making it easier to understand and maintain.
- Standard libraries are typically well-tested and optimized, which means using them can lead to better performance in your applications.
- They can help reduce the chances of introducing bugs, as the standard library functions are more likely to be thoroughly tested and reviewed than custom implementations.
How do I use the standard library in my code?
To use the standard library in your code, you'll first need to import the specific modules or functions that you want to use. The exact syntax for importing will vary depending on the programming language you're working with. For example, in Python, you can import a module from the standard library using the import
statement:
import math
After importing the module, you can access its functions and classes by referring to them using the module name:
result = math.sqrt(25)
Can I use multiple standard libraries in my project?
Yes, you can use multiple standard libraries in your project. In fact, it's quite common to use several standard libraries together to handle different aspects of your application. For example, you might use the os
module in Python for file and directory operations, the re
module for regular expressions, and the json
module for working with JSON data. Just make sure to import each module separately before using its functions and classes.
Are there differences in standard libraries among programming languages?
Yes, there are differences in standard libraries among programming languages. Each language has its own unique set of standard libraries with different modules, functions, and classes. Some functionalities might be available in the standard library of one language but not in another. Additionally, the syntax and usage of standard library functions can also vary between languages. It's essential to consult the documentation for the specific language you're working with to understand the available standard library features and how to use them.