Symbol Table Management
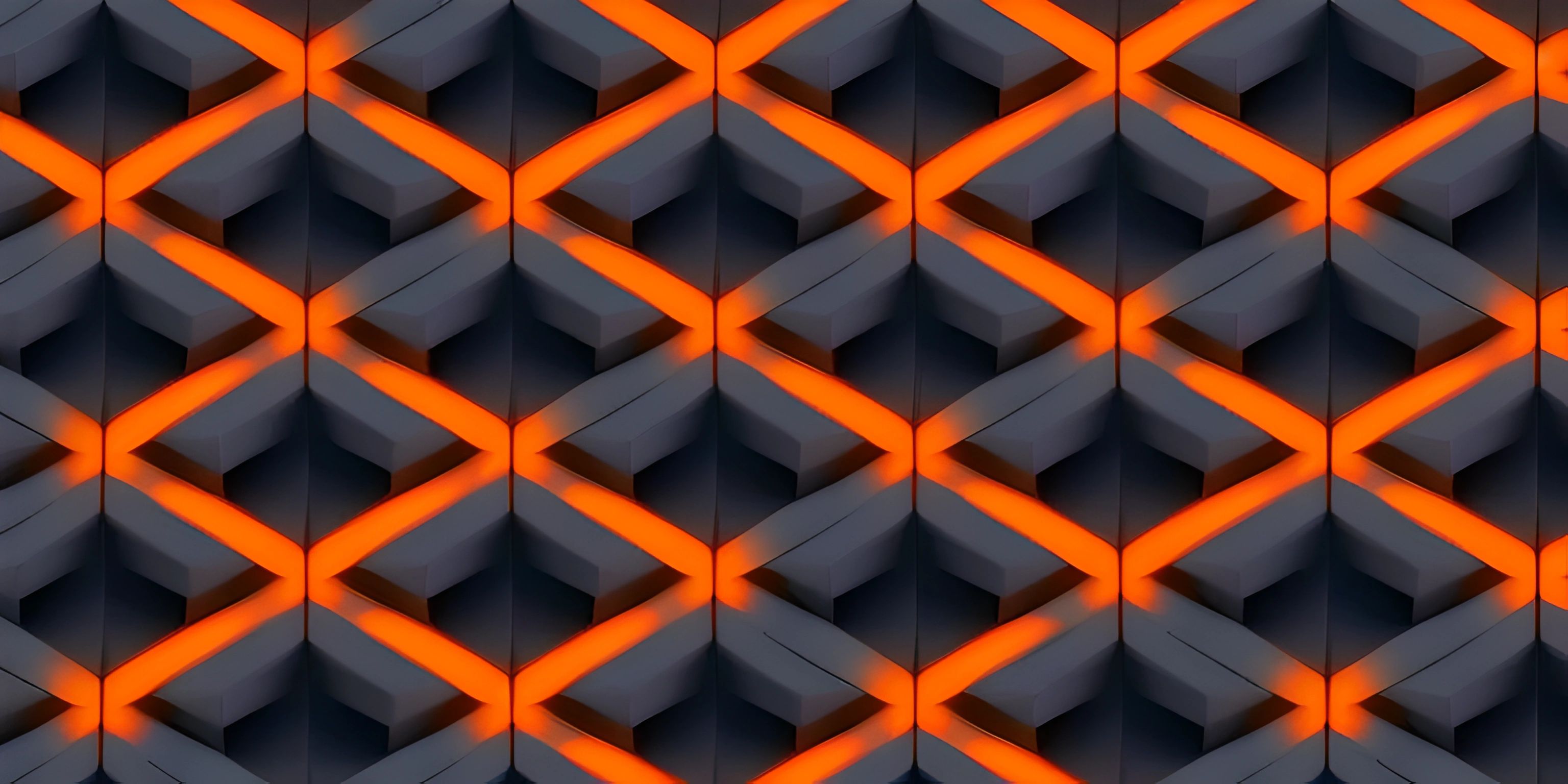
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Symbol tables are the unsung heroes of the compiler world. They help manage all the identifiers (e.g., variable names, function names) and their associated metadata in a program. In this article, we'll dive into the world of symbol table management, why it's essential, and how it's done.
What is a Symbol Table?
A symbol table is a data structure that holds information about the identifiers used in a program. It maps the name of the identifier to its respective properties, such as its type, scope, and memory address. When writing code, you don't want to wade through a sea of variables and functions, trying to remember which is which. Instead, let the symbol table keep track of everything for you.
How does it work?
Imagine you're working on a compiler, and you come across a variable declaration, like int x;
. The compiler needs to remember that x
is an integer, and it'll need some space in memory to store its value. The symbol table steps in, acting like a librarian, keeping track of x
, its type, and eventually, its memory location.
Later, when the compiler encounters a statement like x = 42;
, it can refer to the symbol table, verify that x
exists and is an integer, and then generate the appropriate code to store 42
at x
's memory location.
Symbol Table Management
Managing a symbol table involves inserting, searching, and deleting entries. Let's dive into each of these operations and their importance.
Inserting Entries
When the compiler encounters a new identifier, it needs to be added to the symbol table. This process involves creating a new entry with the identifier's name, type, scope, and any other relevant information. The insertion process may also involve checking for duplicate identifiers within the same scope and throwing an error if one is found.
Searching Entries
When the compiler encounters an identifier in an expression or statement, it must look it up in the symbol table. Searching involves finding the relevant entry for the identifier based on its name and scope. For example, when processing a statement like y = x + 1;
, the compiler searches for x
in the symbol table to determine its type and memory location.
Deleting Entries
As the compiler processes the program, it may enter and exit various scopes. When exiting a scope, the entries associated with that scope should be removed from the symbol table. This cleanup process prevents memory leaks and ensures that identifiers from different scopes don't conflict.
Data Structures for Symbol Tables
Several data structures can be used to implement symbol tables, such as hash tables, binary search trees, and tries. Each has its pros and cons, and the choice depends on factors like the size of the symbol table, the average number of entries per scope, and the frequency of lookups.
-
Hash Tables: These offer constant-time insertion, searching, and deletion, making them an efficient choice. However, they may require more memory than other data structures.
-
Binary Search Trees: These provide logarithmic-time operations but can become imbalanced, leading to degraded performance. Self-balancing trees, like AVL or Red-Black trees, are better options.
-
Tries: These are particularly useful when dealing with symbol tables with a high number of entries sharing common prefixes (e.g., long variable names). Tries offer efficient insertion and searching, but their memory usage can be high.
In conclusion, symbol tables play a crucial role in compiler design, keeping track of identifiers and their attributes. Managing a symbol table involves inserting, searching, and deleting entries as the compiler processes the code. Selecting the appropriate data structure for implementing symbol tables is essential for achieving optimal performance.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What is a symbol table in compiler design?
A symbol table is a data structure used in compiler design to store and manage information about various identifiers (variables, functions, classes, etc.) used in a program's source code. It helps the compiler to quickly look up information about these identifiers during the compilation process, such as their types, scope, and memory locations.
Why is symbol table management important in compiler design?
Symbol table management is vital in compiler design for several reasons:
- It allows the compiler to efficiently store and retrieve information about the identifiers used in the source code.
- It helps in ensuring that identifiers are used correctly, with proper scoping rules and type checking.
- It enables the compiler to generate accurate and efficient machine code by providing information about the memory locations of variables and other identifiers.
- It aids in producing meaningful error messages and debugging information for developers when issues arise in the code.
How are symbol tables organized?
Symbol tables can be organized in various ways, depending on the compiler design and the requirements of the programming language. Common organization methods include:
- Linear lists: A simple list of identifier entries, which can be searched sequentially.
- Hash tables: Using a hash function to map identifier names to specific entries, resulting in faster search times.
- Trees: Organizing entries in a tree structure, where each node represents a scope level, allowing for efficient handling of nested scopes and inheritance.
- Stacks: Utilizing a stack data structure to manage the current scope and handle nested scopes.
Can you give an example of how to create a simple symbol table using a hash table?
Sure! Here's a basic example in Python:
class SymbolTable: def __init__(self): self.table = {} def insert(self, identifier, data): self.table[identifier] = data def lookup(self, identifier): return self.table.get(identifier) # Usage example symbol_table = SymbolTable() symbol_table.insert("variable_name", {"type": "int", "scope": "global"}) # Lookup an identifier print(symbol_table.lookup("variable_name")) # Output: {'type': 'int', 'scope': 'global'}
This creates a simple symbol table using Python's built-in dictionary (hash table) to store and manage the identifiers and their associated data.
How are scopes managed in a symbol table?
Scopes are managed in a symbol table by keeping track of the nesting levels of code blocks, functions, or classes. When a new scope is entered, the symbol table may create a new sub-table, node, or stack frame to handle the new identifiers declared within that scope. Upon exiting the scope, the symbol table returns to the previous level, effectively removing the identifiers declared in the exited scope. This helps in preventing name clashes and maintaining proper visibility of identifiers in their respective scopes.