Understanding Unit Testing
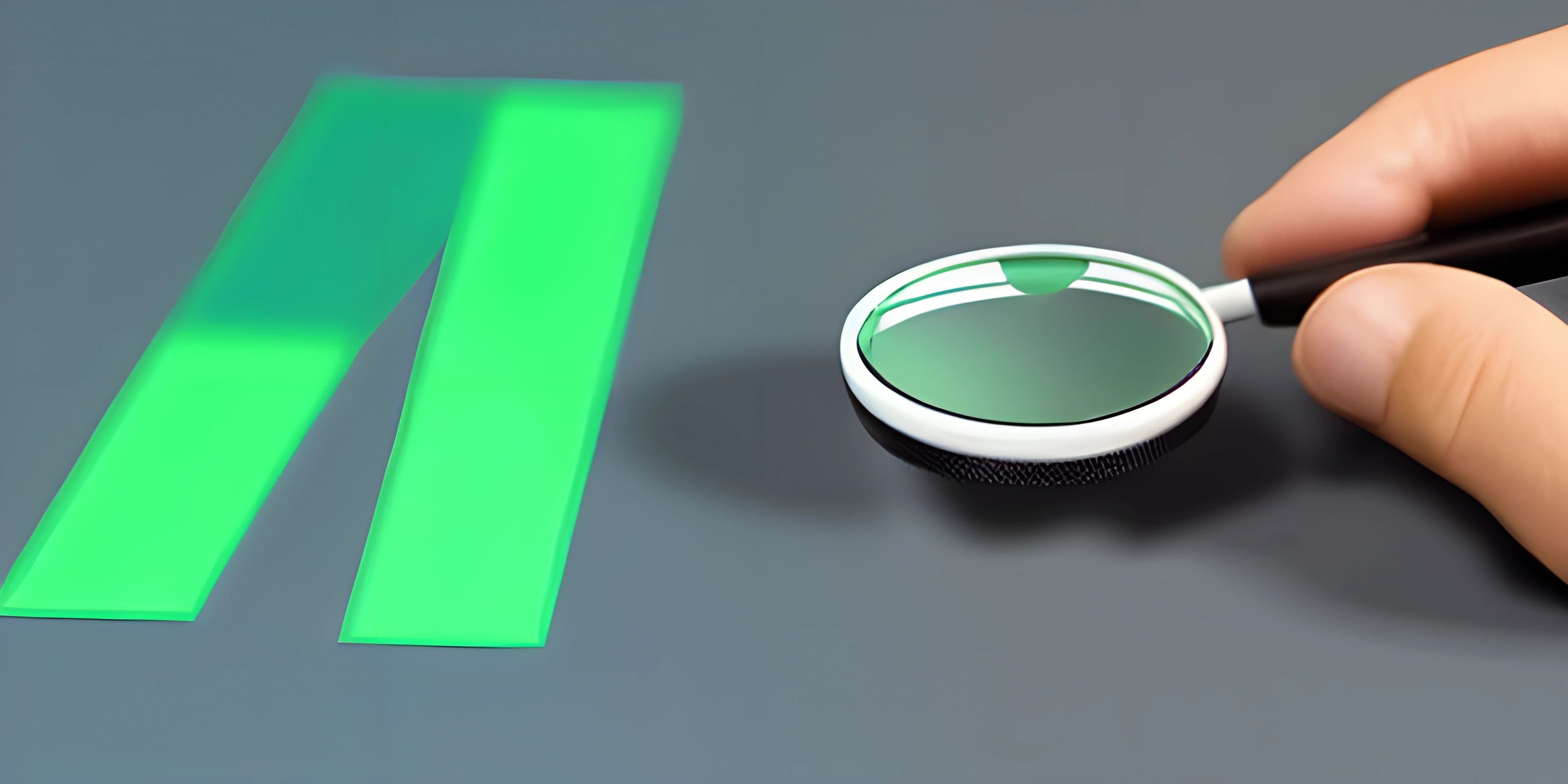
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Unit testing is the process of testing individual components or pieces of your code to ensure that they function correctly. Think of it as a quality assurance step in software development, where each part of your codebase is checked and validated, like a well-oiled machine.
Why is Unit Testing Important?
Unit testing is an essential practice for several reasons:
- Ensures code correctness: By testing individual components of your code, you can catch errors early and ensure that your code behaves as expected.
- Makes debugging easier: If a bug is discovered, unit tests can help you quickly identify the problematic component and fix it.
- Simplifies code refactoring: When you need to modify or improve your code, unit tests provide a safety net to catch any functional regressions that may be introduced.
- Improves code quality: Writing unit tests forces you to think about your code structure and design, leading to cleaner and more maintainable code.
How Do Unit Tests Work?
In unit testing, you write test cases or test functions that target specific components of your code, such as functions or classes. These test functions typically follow a pattern:
- Arrange: Set up the necessary data and objects for the test.
- Act: Execute the code you're testing with the arranged data.
- Assert: Check if the result matches the expected outcome.
Here's a simple example of a unit test in Python:
def add(a, b): return a + b def test_add(): # Arrange a = 2 b = 3 # Act result = add(a, b) # Assert assert result == 5
In this example, we're testing the add
function. The test_add
function sets up the data, calls the add
function, and checks if the result is as expected.
Unit Testing in Different Languages
Unit testing is language-agnostic, and most programming languages have testing frameworks to help you write and run tests. Here are some popular ones:
Explore these frameworks and start incorporating unit testing into your software development process to build more robust and reliable applications.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Making a Basic Discord Bot in JavaScript (discord.js) (psst, it's free!).
FAQ
What is unit testing?
Unit testing is the process of testing individual components or pieces of your code to ensure that they function correctly. It helps ensure code correctness, makes debugging easier, simplifies code refactoring, and improves code quality.
Why is unit testing important?
Unit testing is important because it ensures code correctness, makes debugging easier, simplifies code refactoring, and improves code quality. It is a crucial step in the software development process to maintain the reliability and robustness of an application.
How do unit tests work?
Unit tests work by writing test cases or test functions targeting specific components of your code, such as functions or classes. Test functions typically follow a pattern of arranging the necessary data and objects, executing the code being tested, and asserting that the result matches the expected outcome.
What are some popular unit testing frameworks for different programming languages?
Some popular unit testing frameworks include pytest for Python, Jest for JavaScript, JUnit for Java, NUnit for C#, and RSpec for Ruby. Each framework provides tools and features to help you write and run tests in their respective languages.