Using Constants in Javascript
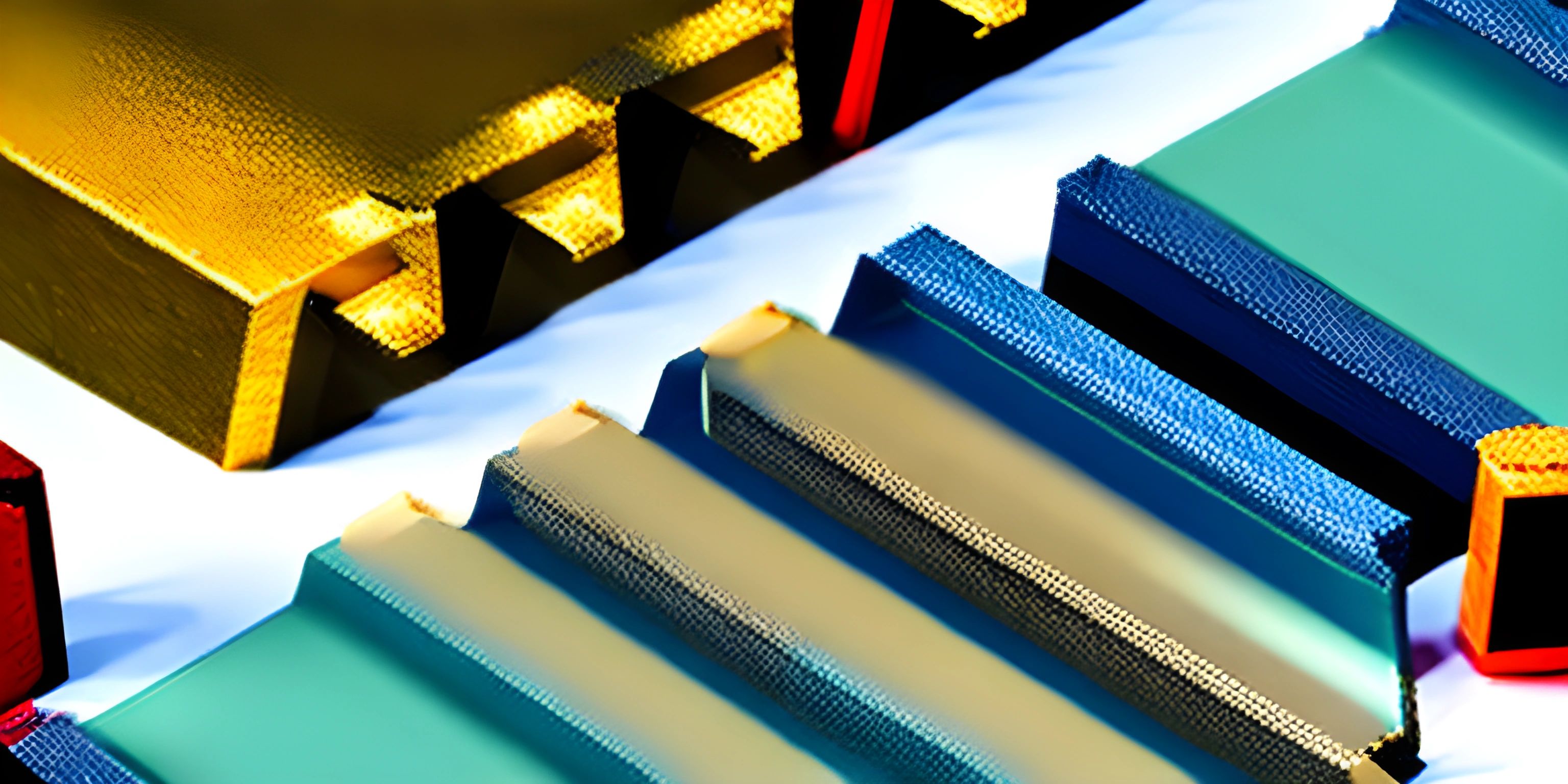
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
The world of programming is full of mutable things. Variables change, functions can be redefined, and data can be manipulated. But amidst all this, there is something immutable, something that remains unchanging under all circumstances. It's a rock in the tempest, a lighthouse amidst stormy seas, a... well, you get the idea. I'm talking about constants.
In Javascript, constants (declared with the const
keyword) are fixed values that cannot be changed after they are initialized. They're like the North Star: always there, always the same, and great for navigation.
Constants: Like a Promise You Can't Break
Unlike their mutable counterparts (variables), constants are unwavering. Once you declare a constant and assign it a value, that value is set in stone. Here's an example:
const name = "Ada"; name = "Grace"; // This line will throw an error
In this example, name
is a constant. We've promised Javascript that name
will always be "Ada", and Javascript takes our word seriously. When we try to change name
to "Grace", Javascript throws an error, because we're breaking our promise.
Why Use Constants?
You might be wondering, "Why would I use a constant when I could use a variable?" Well, constants serve a few key purposes.
Firstly, they improve readability. If you see a constant in the code, you can trust that its value won't change. This makes the code easier to understand, as you don't have to keep track of the constant's value.
Secondly, they prevent accidental reassignment. If you've ever spent hours debugging your program only to find out you accidentally changed a variable's value, then you'll appreciate constants. With constants, such mishaps are prevented.
Lastly, they can make your code more maintainable. Constants signal to others (and future you) that a value should not be changed. This can simplify the process of updating or refactoring your code.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Basic Concepts (psst, it's free!).
FAQ
What is a constant in Javascript?
In Javascript, a constant is a fixed value that cannot be changed after it is initialized. They are declared using the const
keyword.
Why should I use constants instead of variables?
Constants improve readability, prevent accidental reassignment, and make your code more maintainable. They give you the assurance that the value won't change throughout the code, simplifying the process of reading and debugging your code.
Can constants be used for objects and arrays?
Yes, constants can be used for objects and arrays. But remember, while the reference to the object or array cannot be changed, the contents of the object or array can be modified.