Exploring Trees and Their Uses in Computer Science
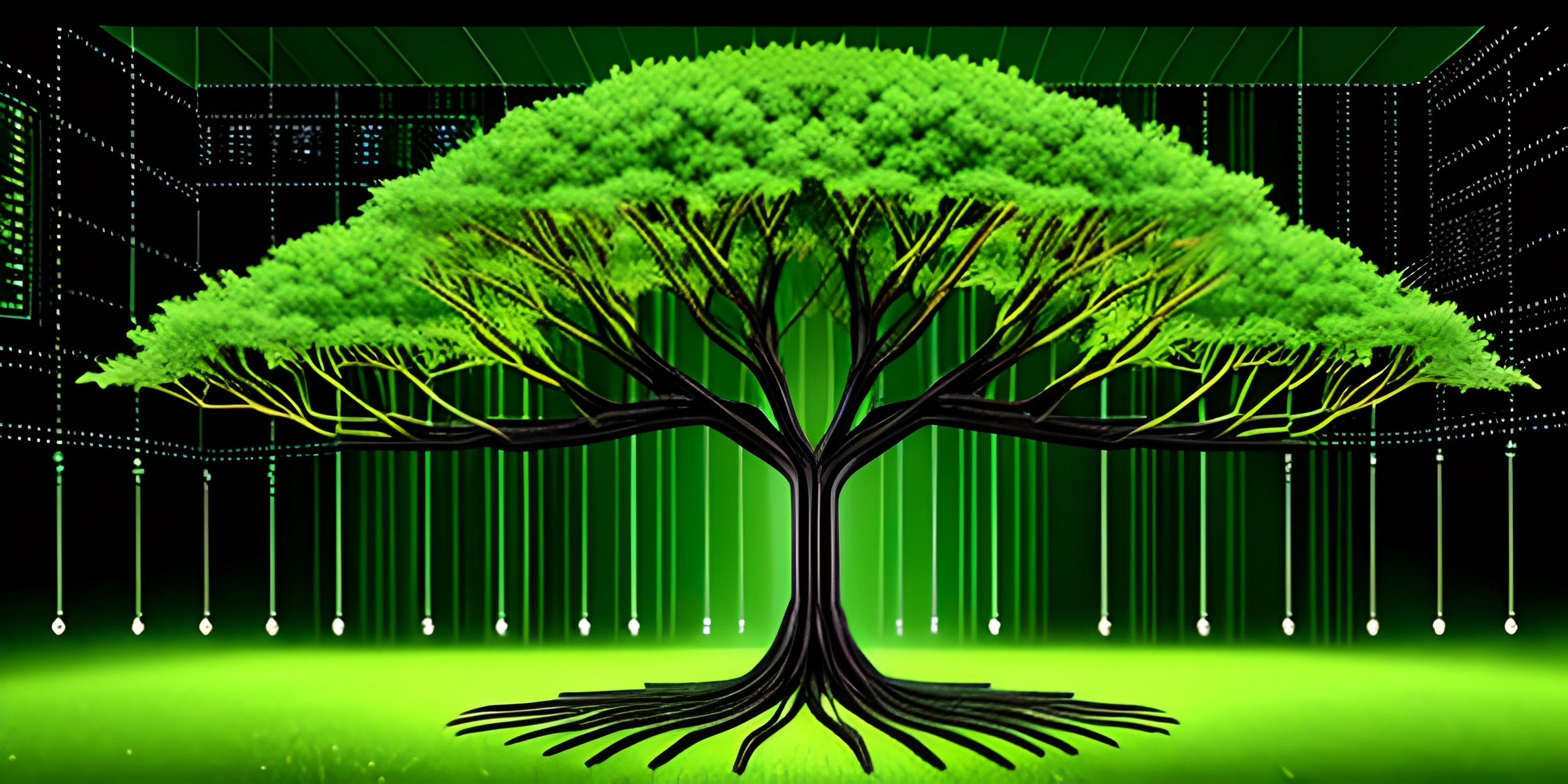
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Trees are everywhere in computer science. They're so prevalent that they've embedded themselves in the very roots of our code. But what is a tree, and why is it such a popular data structure? Let's branch out and explore these leafy wonders.
What is a Tree?
A tree is a hierarchical data structure made up of nodes, where each node can have zero or more child nodes. Each node contains some data, and the connections between nodes represent relationships between the data points. In a tree, there is a special node called the root which has no parent node, and all other nodes can be reached from the root by following a unique sequence of connections.
Trees are a non-linear data structure, which means they don't follow a straight path like arrays or linked lists. Instead, they branch out and organize data in a hierarchical manner. This allows for more efficient organization and searching of data in certain scenarios.
Binary Trees
One of the most popular types of trees is the binary tree. In a binary tree, each node can have at most two children, commonly referred to as the left and the right child. Binary trees are often used for searching and sorting operations, as they can help reduce the time complexity of these tasks.
Tree Traversal
Traversing a tree means visiting all the nodes in the tree in a specific order. There are several common ways to traverse a tree, including:
- Preorder traversal: Visit the root node first, then traverse the left subtree, followed by the right subtree.
- Inorder traversal: Traverse the left subtree first, then visit the root node, and finally traverse the right subtree.
- Postorder traversal: Traverse the left subtree first, then the right subtree, and finally visit the root node.
These traversal methods are used in various algorithms and applications, depending on the problem being solved.
Trees in Computer Science
Trees are incredibly versatile and are used in a wide range of computer science applications. Here are a few examples:
File Systems
Your computer's file system is organized as a tree, with directories acting as nodes and files as leaves. This structure allows for efficient organization and retrieval of files within the system.
Databases
Many databases use tree structures, such as B-trees and AVL trees, to organize and store data efficiently. These trees help optimize search, insertion, and deletion operations, ensuring quick database access even as the amount of data grows.
Artificial Intelligence
In AI, trees are used in various algorithms, such as decision trees and game trees, to represent decision-making processes and search for optimal solutions. By systematically exploring the tree, an AI can evaluate different choices and outcomes to make intelligent decisions.
Compiler Design
In compiler design, trees are used to represent the structure of a program's source code. These trees, called abstract syntax trees (AST), are created during the parsing stage of compilation and help the compiler understand and optimize the code being compiled.
The Forest of Algorithms
As you venture deeper into the world of computer science, you'll encounter many more trees and their uses. From trie structures used for efficient string manipulation to Huffman trees used in data compression, trees are branching out and taking root in every corner of the field.
So remember, next time you're lost in a forest of code, take a moment to bask in the shade of the trees that make it all possible.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Recursion Intro (psst, it's free!).
FAQ
What are tree data structures and why are they important in computer science?
Tree data structures are a type of hierarchical data structure that is organized into connected nodes, where each node has a parent node and zero or more child nodes. Trees are important in computer science because they provide an efficient way to organize and search through data, making them useful in various applications, such as file systems, databases, compilers, and artificial intelligence.
How is a binary tree different from other types of trees?
A binary tree is a specific type of tree data structure where each node can have at most two child nodes, usually referred to as the left and right children. This restriction allows for efficient algorithms and operations, such as searching, insertion, and deletion, making binary trees particularly useful for tasks like sorting and searching.
What are some common tree traversal techniques?
There are several common tree traversal techniques, including:
- Pre-order traversal: Visit the root node first, then traverse the left subtree and the right subtree.
- In-order traversal: Traverse the left subtree, visit the root node, and then traverse the right subtree. This is often used in binary search trees, as it produces a sorted sequence of nodes.
- Post-order traversal: Traverse the left subtree, then the right subtree, and finally visit the root node.
- Level-order traversal: Visit the nodes level by level, starting with the root node, and moving from left to right.
Can you provide an example of a tree data structure in a real-world application?
One common real-world application of tree data structures is file systems. In a file system, directories can be seen as tree nodes, with each directory containing subdirectories and files as child nodes. This tree-like structure allows for efficient organization and searching of files and directories within the system.
What is a balanced tree and why is it important?
A balanced tree is a tree in which the heights of the left and right subtrees of every node differ by at most one. Balanced trees are important because they ensure that operations, such as searching, insertion, and deletion, take optimal time. In the case of a balanced binary search tree, these operations have a time complexity of O(log n), where n is the number of nodes in the tree. Unbalanced trees, on the other hand, can lead to inefficient operations and degraded performance.