Data Structures Overview
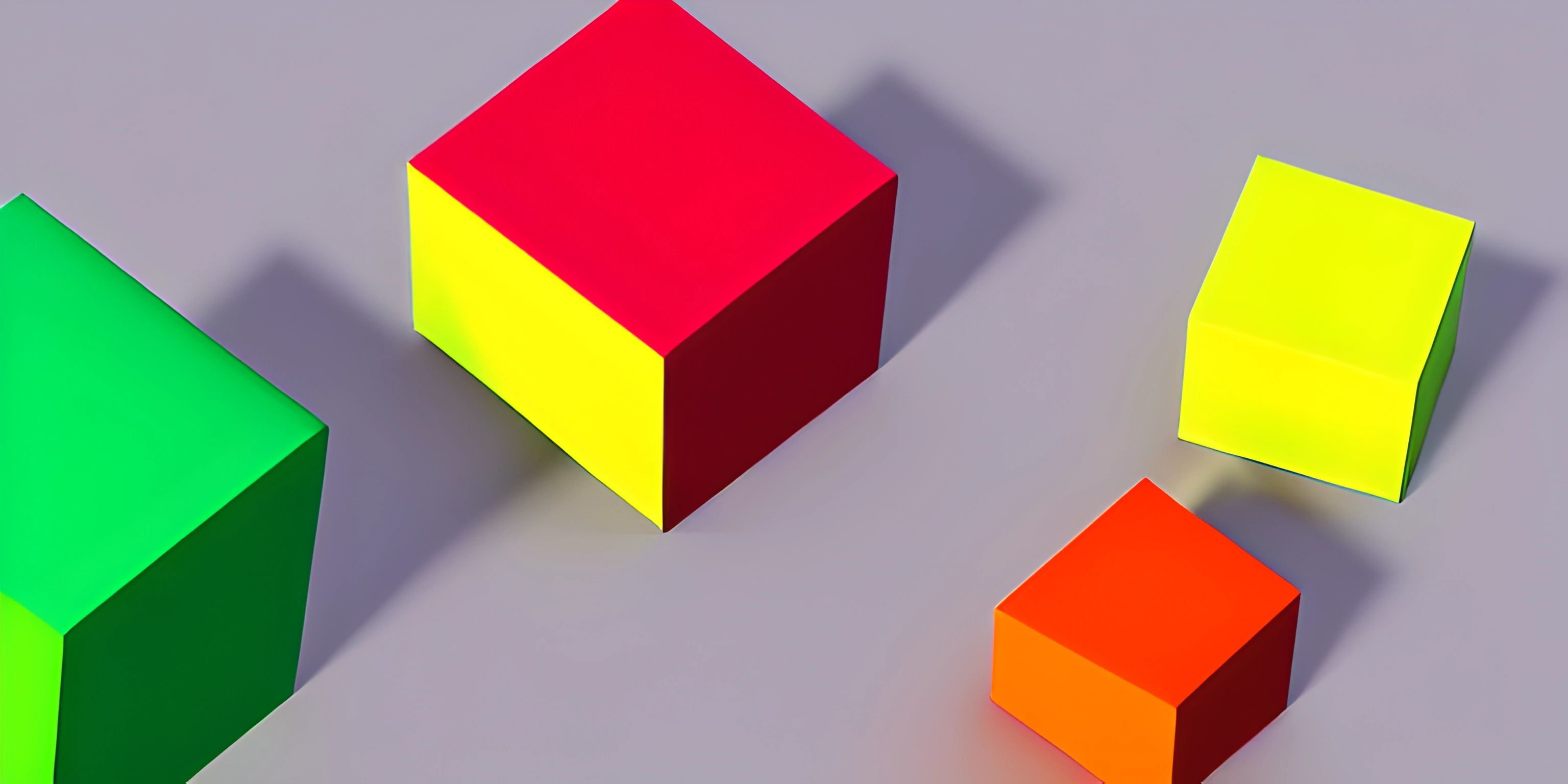
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Data structures are like the spice rack of programming. They're the essential ingredients that hold your program's data and keep it organized. We'll explore some common data structures and their uses, so you can pick the right one for your next programming endeavor.
Array
An array is a fixed-size collection of elements, all of the same data type. You can think of an array as a limited parking lot with numbered spaces, where you can park only specific types of vehicles. Here's an example of an array in Python:
my_array = [1, 2, 3, 4, 5]
Arrays provide fast access to elements using indices, but their fixed size can make them inflexible.
List
A list is a dynamic data structure that can grow or shrink in size. It's like a more versatile version of an array. Imagine a parking lot with the ability to create new spaces or remove unused ones. Here's an example of a list in Python:
my_list = [1, 2, 3, 4, 5]
Lists are great for storing a varying number of elements, but they can be slower than arrays when searching or modifying elements.
Stack
A stack is a data structure that works on a last-in, first-out (LIFO) principle. Think of it like a stack of pancakes: you can only add or remove pancakes from the top. Here's an example of a stack using Python's list
:
stack = [] stack.append(1) # Push 1 onto the stack stack.append(2) # Push 2 onto the stack stack.pop() # Pop the top element (2) off the stack
Stacks are used when you need to keep track of nested structures or when you want to reverse the order of elements.
Queue
A queue is a data structure that works on a first-in, first-out (FIFO) principle. Picture a line of people waiting for a bus: the first person in line gets on the bus first. Here's an example of a queue using Python's deque
:
from collections import deque queue = deque() queue.append(1) # Enqueue 1 queue.append(2) # Enqueue 2 queue.popleft() # Dequeue the first element (1)
Queues are useful for maintaining the order of elements and processing tasks in a sequential manner.
Dictionary
A dictionary (also known as a hash table or associative array) is a collection of key-value pairs. It's like a real dictionary, where you have a word (the key) and its definition (the value). Here's an example of a dictionary in Python:
my_dict = {"apple": "a tasty fruit", "banana": "a yellow fruit"}
Dictionaries provide fast access to values using keys, making them ideal for indexing or caching purposes.
Tree
A tree is a hierarchical data structure that consists of nodes and edges, where each node has a parent and zero or more children. Think of it like a family tree. Here's an example of a simple tree structure:
A / \ B C / \ / \ D E F G
Trees are used for organizing data hierarchically, managing dependencies, and implementing search algorithms.
Graph
A graph is a data structure consisting of nodes (or vertices) and edges (or connections) between them. Imagine a map of cities, where each city is a node and the roads connecting them are edges. Here's an example of a simple graph:
A -- B -- C \ | / \ | / D
Graphs are useful for representing complex relationships, routing and navigation, and solving network problems.
Now that you've got a taste of these essential data structures, get ready to whip up some delicious code to satisfy your programming cravings!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Recursion Intro (psst, it's free!).
FAQ
What are data structures and why are they important?
Data structures are specialized formats used to organize, store, and manipulate data in a computer's memory. They are important because they help us efficiently manage large amounts of data and perform complex tasks. Choosing the right data structure can greatly impact a program's performance and readability.
What are some common types of data structures?
Some common types of data structures include:
- Arrays: A fixed-size, ordered collection of elements, usually of the same data type.
- Linked Lists: A collection of elements, where each element points to the next element in the sequence.
- Stacks: A collection of elements with the Last-In-First-Out (LIFO) property, meaning the most recently added element is always removed first.
- Queues: A collection of elements with the First-In-First-Out (FIFO) property, meaning the oldest element is removed first.
- Trees: A hierarchical data structure consisting of nodes with a parent-child relationship, with one root node and multiple levels of branches and leaves.
- Graphs: A collection of nodes and edges, where nodes represent entities and edges represent relationships between entities.
- Hash Tables: A data structure that uses a hash function to map keys to their associated values, allowing for efficient data retrieval and insertion.
How do I choose the right data structure for my problem?
Choosing the right data structure depends on the specific requirements of your problem, such as the type of data you're working with, the operations you need to perform, and the desired time and space complexity. Analyze your problem carefully and consider how different data structures may impact your program's performance and readability. As you gain more experience working with data structures, you'll develop a better intuition for making these decisions.
Can I combine multiple data structures to solve a problem?
Absolutely! In fact, many complex problems require combining multiple data structures to achieve the desired functionality and performance. For example, you might use a combination of a hash table and a linked list to implement a Least Recently Used (LRU) cache. The key is to understand the strengths and weaknesses of each data structure and how they can complement each other to solve your problem efficiently.
Are data structures language-specific?
While the concept of data structures is universal, their implementation may vary across programming languages. Some languages have built-in support for certain data structures, while others require you to implement them from scratch. It's important to understand the data structures available in your chosen programming language and how to use them effectively.