Tree Data Structure Intro
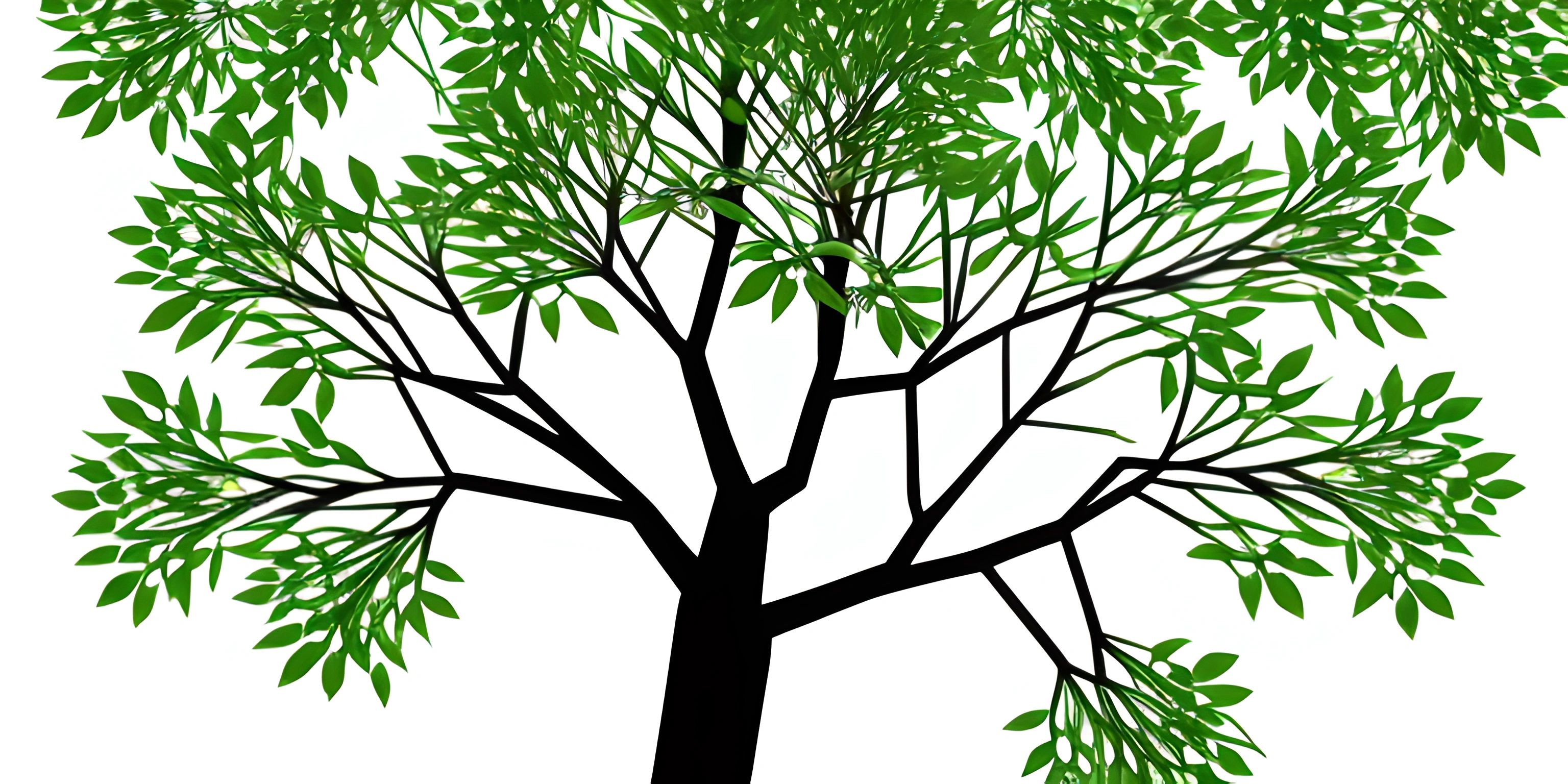
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Trees are one of the most versatile and widely used data structures in programming. Imagine a family tree, but instead of people, we have pieces of data connected by branches, forming a hierarchy. That's a tree data structure in a nutshell!
What is a Tree?
A tree is a hierarchical data structure that consists of nodes connected by edges. The top node is called the root, and each node can have zero or more child nodes. The nodes with no child nodes are called leaves.
Here's an example of a tree:
A / \ B C / \ \ D E F
In this tree, A is the root, B and C are child nodes of A, and D, E, and F are leaves.
Why Use a Tree?
Trees are excellent for representing hierarchical relationships and for efficiently searching, inserting, and deleting elements. Some common uses of trees include:
- Representing file systems in operating systems
- Storing data in databases
- Efficiently searching through large datasets, like in search engines
- Parsing programming languages and generating abstract syntax trees (AST)
Types of Trees
There are numerous types of trees, each catering to different needs and use cases. Here are a few popular ones:
- Binary Trees: Each node has at most two child nodes. This type of tree is useful for searching, sorting, and arithmetic operations.
- Balanced Trees: A binary tree where the height difference between the left and right subtree of any node is at most one. Examples include AVL trees and red-black trees, which are used in databases and filesystems.
- Tries: A tree where nodes store characters of a string, and strings can be searched by traversing nodes from the root. Tries are used in text processing and search engines.
- Heap Trees: A binary tree with specific properties, like the parent node is always greater (or smaller) than its children. Heaps are used in priority queues and sorting algorithms.
Basic Tree Operations
Here are some common operations in tree data structures:
- Insert: Add a new node to the tree, maintaining the tree's properties.
- Delete: Remove a node from the tree, maintaining the tree's properties.
- Search: Find a node in the tree based on a specific value or property.
- Traversals: Visit each node in a specific order, such as in-order, pre-order, or post-order traversal.
There's so much more to learn about tree data structures, but this introduction should give you a solid foundation to build upon. So go ahead and explore the wonderful world of trees, where branching out into new heights of programming knowledge awaits you!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Recursion Intro (psst, it's free!).
FAQ
What is a tree data structure and why is it important in programming?
A tree data structure is a non-linear, hierarchical data organization model where data is represented in nodes connected by edges. It is important in programming as it allows for efficient storage, retrieval, and manipulation of data. Tree structures are widely used in various algorithms, searching and sorting operations, and managing hierarchical relationships.
How is a tree data structure different from other data structures like arrays or linked lists?
Unlike arrays and linked lists, which are linear data structures, tree data structures are hierarchical, with each element (called node) having a parent-child relationship with other nodes. This structure enables quicker search, insert, and delete operations in many cases, as well as better representation of complex data relationships.
What are some common types of tree data structures?
There are several types of tree data structures, including:
- Binary Trees: Each node has at most two children.
- Binary Search Trees: A binary tree where each node's left subtree contains only nodes with values less than the parent node, and the right subtree contains nodes with values greater than the parent node.
- AVL Trees: A self-balancing binary search tree that maintains a height-balanced tree for efficient search operations.
- Trie (Prefix Tree): A tree where each node represents a character in a string and is often used for efficient searching of words in a dictionary.
- Heap: A binary tree with specific properties, such as a min-heap (where parent nodes are smaller than their children nodes) or a max-heap (where parent nodes are larger than their children nodes).
How do I traverse a tree data structure?
There are several ways to traverse a tree data structure, and the choice depends on the specific use case. The most common traversal methods are:
- Depth-First Search (DFS): Explores as far as possible along each branch before backtracking. It includes in-order, pre-order, and post-order traversal techniques.
- Breadth-First Search (BFS): Visits all the nodes at the same level before moving down to the next level. Also known as level-order traversal.
Can tree data structures be used to represent real-world scenarios?
Yes, tree data structures are often used to represent real-world scenarios. For example, they can represent organizational structures, file systems, document structures (like XML or JSON), and game decision trees. Their hierarchical nature allows for efficient representation and manipulation of complex data relationships.