Understanding Tree Data Structures
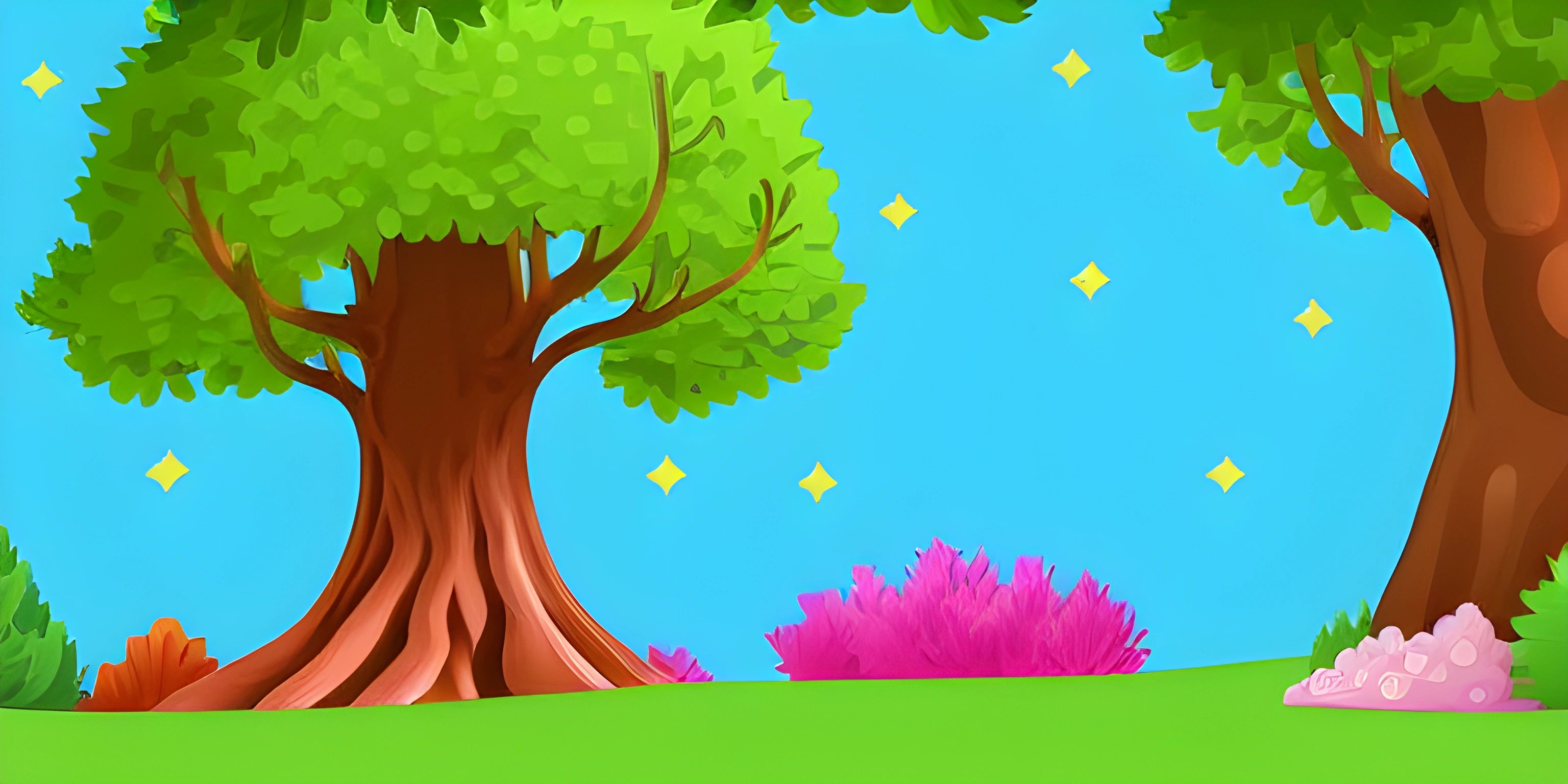
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Ever glanced at a family tree and marveled at how it represents relationships between individuals? In programming, we have a similar concept called a tree data structure, which helps us store data in a hierarchical pattern.
Roots, Nodes, and Leaves
The tree data structure is composed of smaller units called nodes. There's a special node known as the root node. It's like the granddaddy of all nodes, from which every other node descends. Nodes linked directly to a parent node are called its children. Nodes with no children are often referred to as leaf nodes, similar to the leaves on a tree that have no further branches.
Let's visualize this with pseudocode:
Node { value, leftChild, rightChild }
Suppose the root node has a value of 5. The left child could be 3, and the right child could be 8. Each child node could further have left and right children, forming a tree-like structure.
Types of Trees
One common type of tree is a binary tree, where each parent node has at most two children nodes. These are often used in search algorithms due to their efficiency.
Why Trees?
You might be wondering, why the fuss about trees? Can't we just use arrays or linked lists? Well, trees are incredibly helpful for storing data that naturally form hierarchies like website domains or file systems. Moreover, operations like searching, inserting, and deleting data are faster in tree structures.
Trees are also the backbone of many key algorithms in computer science, such as the Depth-First Search and Breadth-First Search. So, understanding trees is crucial to becoming a proficient programmer!
FAQ
What is a tree data structure?
A tree data structure is a type of data structure that stores data in a hierarchical pattern. It consists of nodes connected by edges. The topmost node is called the root, and the nodes with no children are called leaves.
What is a binary tree?
A binary tree is a type of tree where each parent node can have at most two children nodes. This makes binary trees particularly useful in various search and sorting algorithms due to their structure.
Why are tree data structures used in programming?
Tree data structures are used in programming because they are efficient in performing operations like searching, insertion, and deletion. They are also useful for storing data that naturally forms a hierarchy, such as a file system or a website domain. Furthermore, tree structures are foundational to many important algorithms in computer science.