Mocks and Stubs: Understanding the Difference and How to Use Them
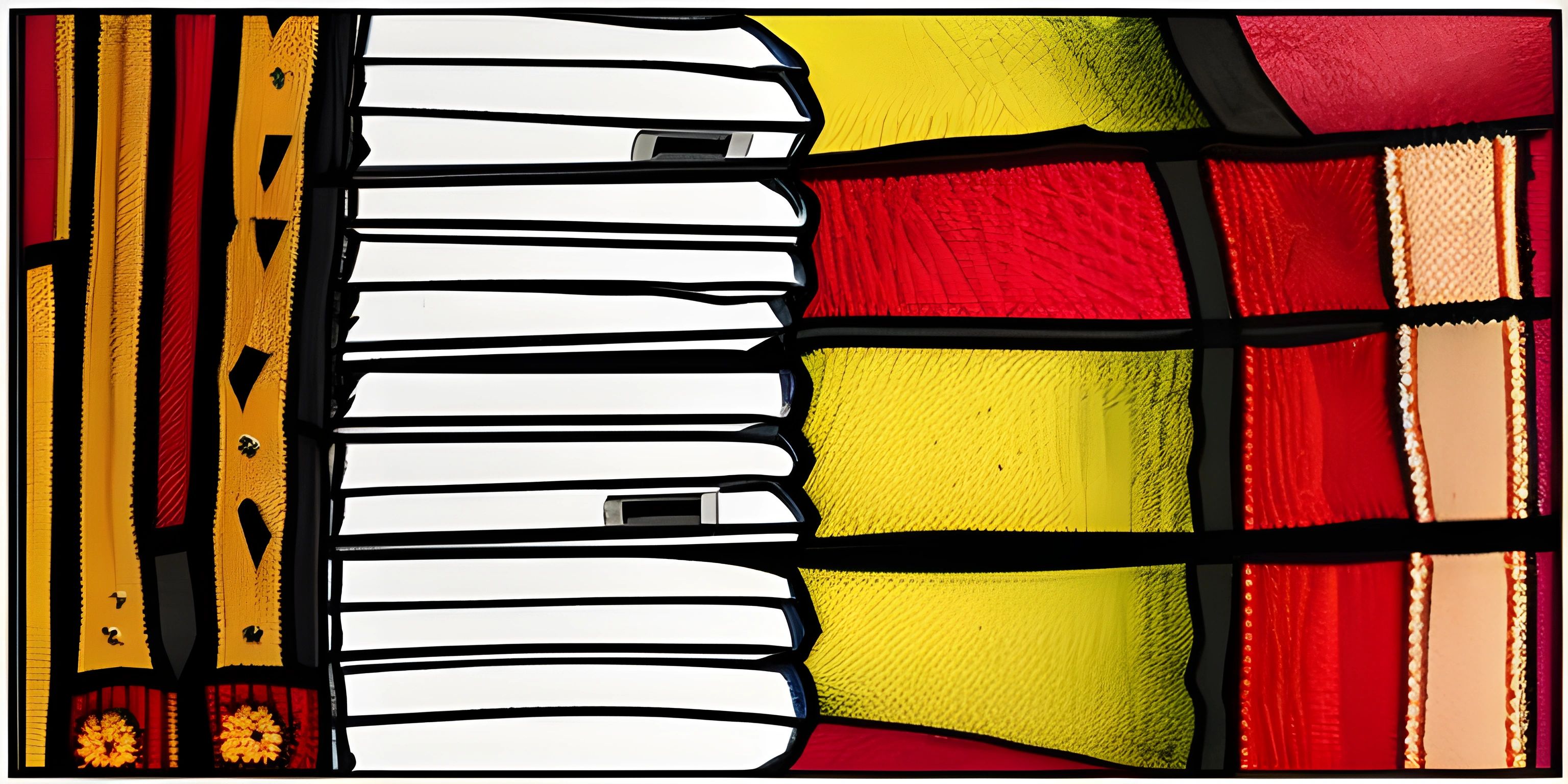
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
When it comes to testing our code, we often need to rely on external components or objects. These objects might not always be available, or we might not want to use the real objects to avoid side effects. In these cases, we can use mocks and stubs. But what exactly are they and how do they differ? Let's dive in!
Mocks
Mocks are objects that simulate the behavior of real objects in a controlled way. They are used for isolating the code under test and allow us to verify how the code interacts with external dependencies. In short, mocks help us ensure that our code is calling the right methods with the right arguments on external objects.
Here's an example using Python and the unittest.mock library:
from unittest.mock import Mock # Create a mock object email_service = Mock() # Use the mock object in our code email_service.send_email("[email protected]", "Welcome!") # Assert that the send_email method was called with the correct arguments email_service.send_email.assert_called_once_with("[email protected]", "Welcome!")
Stubs
Stubs are also used to replace real objects, but they are simpler than mocks. They provide canned responses to method calls without any validation or verification. Stubs are great for providing consistent and predictable results during testing.
Here's an example of a stub in Python:
class StubEmailService: def send_email(self, recipient, message): return "Email sent" # Use the stub object in our code email_service = StubEmailService() result = email_service.send_email("[email protected]", "Welcome!") # Assert that the stub returned the expected result assert result == "Email sent"
When to Use Mocks and Stubs
Generally, you should use mocks when you want to verify the interaction between your code and external dependencies, while stubs are useful for providing fixed, predictable responses.
Remember that mocks and stubs help you test your code in isolation, allowing you to focus on the functionality of the code under test without worrying about the actual behavior of external components.
Conclusion
Mocks and stubs play a crucial role in testing our code by allowing us to isolate it from external dependencies. Understanding the difference between them and knowing when to use each will improve the robustness of your tests and the overall quality of your software.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: What Programming Means (psst, it's free!).
FAQ
What are mocks?
Mocks are objects that simulate the behavior of real objects in a controlled way. They are used for isolating the code under test and allow us to verify how the code interacts with external dependencies.
What are stubs?
Stubs are simple objects that replace real objects and provide canned responses to method calls without any validation or verification. They are great for providing consistent and predictable results during testing.
When should I use mocks and when should I use stubs?
You should use mocks when you want to verify the interaction between your code and external dependencies, while stubs are useful for providing fixed, predictable responses.
Why are mocks and stubs important in testing?
Mocks and stubs help you test your code in isolation, allowing you to focus on the functionality of the code under test without worrying about the actual behavior of external components. This leads to more robust tests and better overall software quality.
How can I create mocks and stubs in Python?
In Python, you can create mocks using the unittest.mock
library and create stubs by defining custom classes with the desired canned responses.