SOLID Principles in Software Design
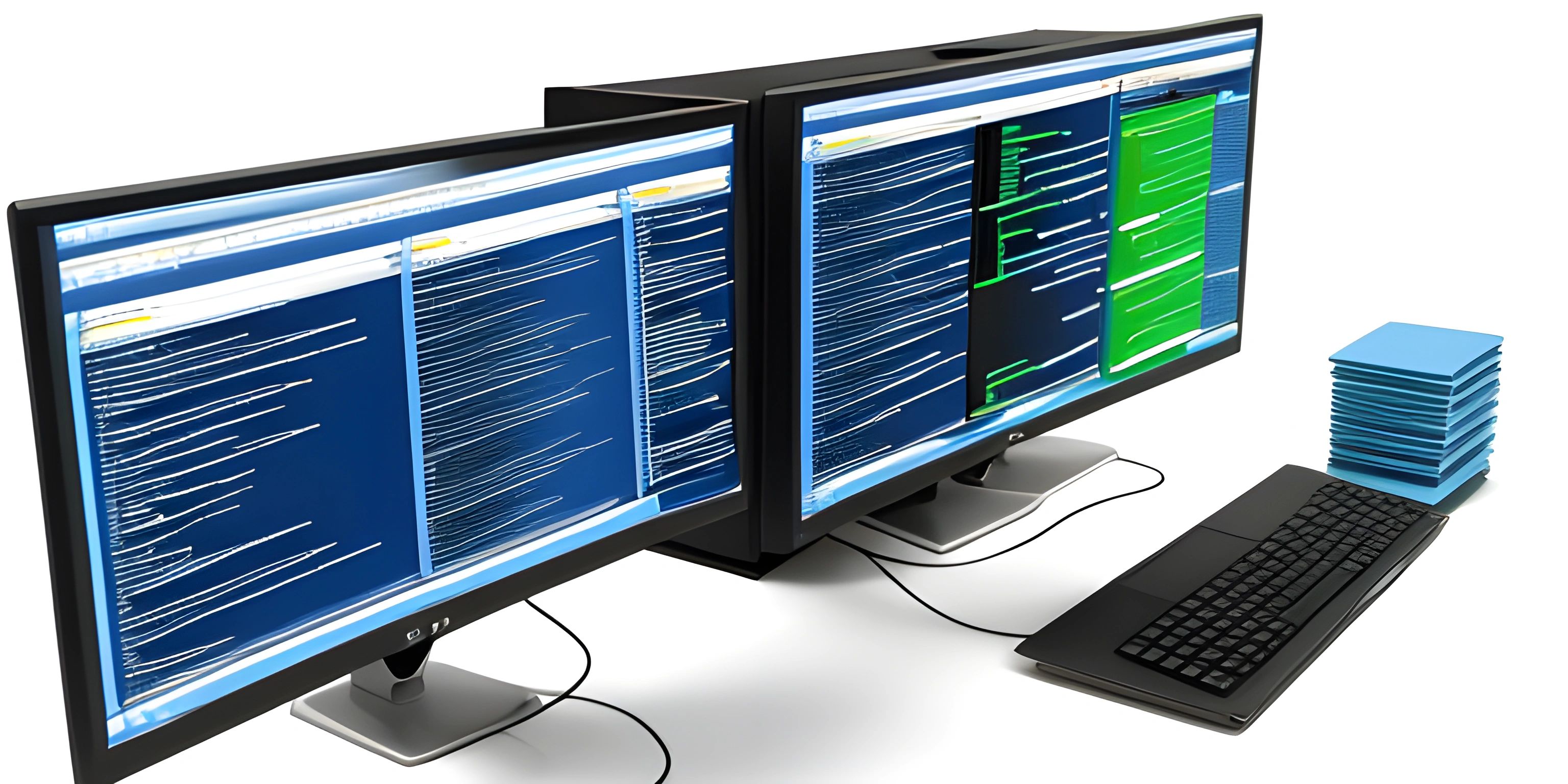
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
SOLID principles are a set of five design guidelines that can help you create more modular, maintainable, and scalable software applications. They are an essential part of any developer's toolkit, especially for those working with object-oriented programming (OOP). Let's dive into each of the SOLID principles and explore their importance.
Single Responsibility Principle (SRP)
The Single Responsibility Principle states that a class should have only one reason to change. In other words, a class should be responsible for a single piece of functionality. This makes the code easier to manage, test, and understand.
For example, consider an application that processes orders. Instead of having one massive class that handles everything related to orders, you would break it down into smaller, more focused classes, like Order
, Payment
, Shipping
, and so on.
Open/Closed Principle (OCP)
The Open/Closed Principle states that software entities (classes, modules, functions, etc.) should be open for extension but closed for modification. This means that you should be able to add new functionality without modifying existing code, reducing the risk of introducing bugs.
To achieve this, you can use abstractions, such as interfaces or abstract classes, and rely on inheritance and polymorphism. For example, if you have a Shape
interface and various classes implementing it (Circle
, Square
, Triangle
), you can add a new shape class without changing the code that uses the Shape
interface.
Liskov Substitution Principle (LSP)
The Liskov Substitution Principle states that objects of a derived class should be able to replace objects of the base class without affecting the correctness of the program. In other words, if a class B
is a subclass of class A
, you should be able to use an instance of B
wherever you use an instance of A
.
This principle encourages creating subclasses that don't break the behavior defined by the base class, ensuring that your code remains consistent and predictable. It helps in creating a robust class hierarchy that can be easily extended and maintained.
Interface Segregation Principle (ISP)
The Interface Segregation Principle states that clients should not be forced to depend on interfaces they do not use. In other words, it's better to have multiple small, focused interfaces rather than a single large one, which may contain methods that a client doesn't need.
This principle promotes the separation of concerns, allowing you to create more modular and flexible code. For example, instead of having a single Vehicle
interface, you could create separate interfaces for Drivable
, Flyable
, and Sailable
, allowing you to mix and match functionalities as needed.
Dependency Inversion Principle (DIP)
The Dependency Inversion Principle states that high-level modules should not depend on low-level modules, but both should depend on abstractions. It also suggests that abstractions should not depend on details, but details should depend on abstractions.
This principle encourages you to write code that depends on abstractions rather than concrete implementations. This can be achieved through techniques such as dependency injection, interface-based programming, and the use of inversion of control containers. Applying DIP helps you create more modular, testable, and maintainable code.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What are the SOLID principles?
SOLID principles are a set of five design guidelines for creating maintainable and scalable software applications. They include Single Responsibility Principle (SRP), Open/Closed Principle (OCP), Liskov Substitution Principle (LSP), Interface Segregation Principle (ISP), and Dependency Inversion Principle (DIP).
Why are SOLID principles important?
SOLID principles are important because they help developers create more modular, maintainable, and scalable software applications. By adhering to these principles, you can reduce the likelihood of introducing bugs, improve code readability, and make it easier to extend and modify your applications.
How can I apply the Open/Closed Principle in my code?
To apply the Open/Closed Principle, you can use abstractions such as interfaces or abstract classes and rely on inheritance and polymorphism. This allows you to extend existing functionality without modifying the existing code, reducing the risk of introducing bugs.