Unity 3D Game Development
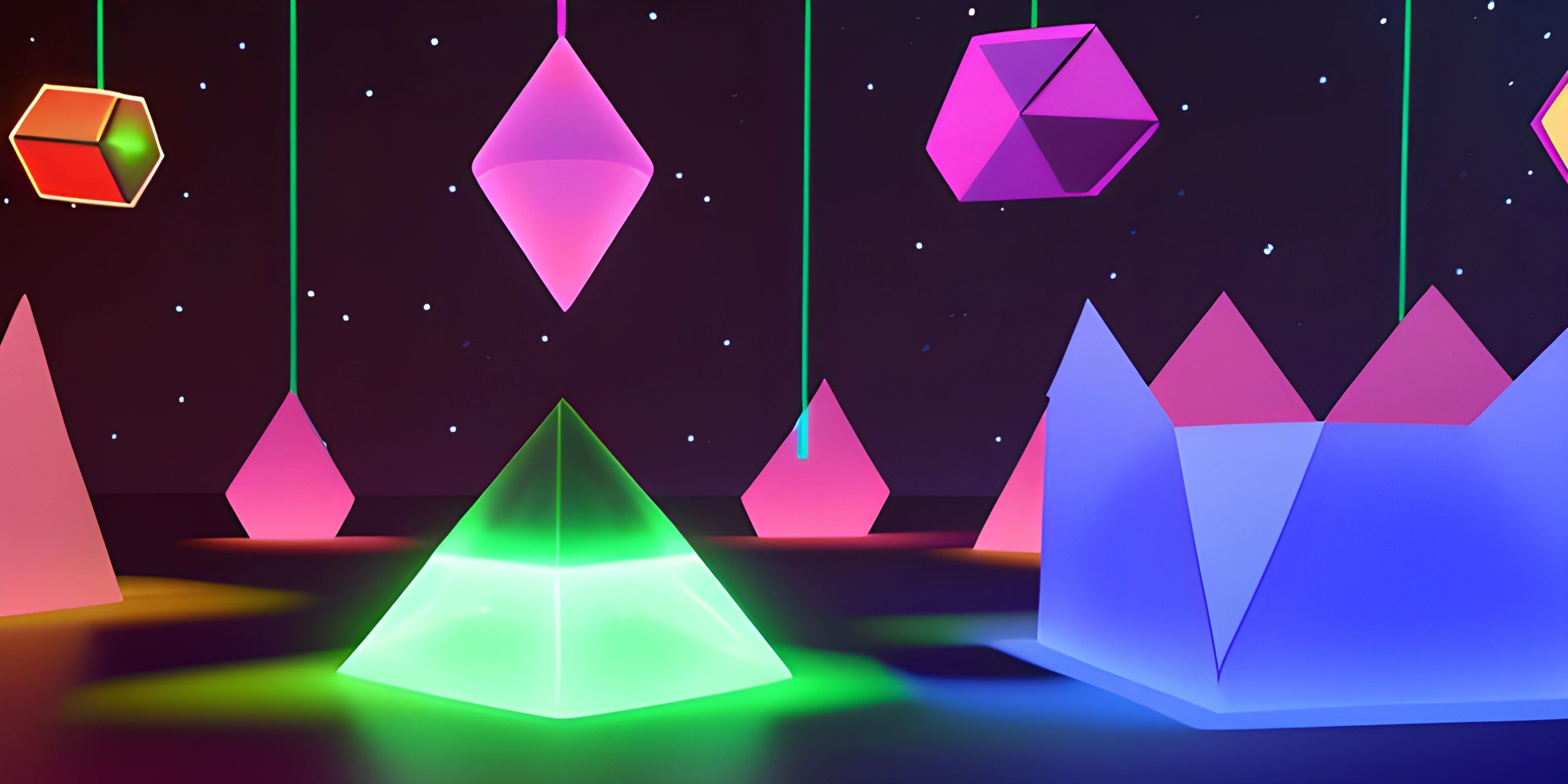
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Building 3D games in Unity from scratch can feel like a daunting task, but fear not! With a little know-how, you'll be creating fantastic virtual worlds in no time. In this article, we'll go through the basics of Unity, its interface, and the essential elements: scenes, assets, and scripting.
Unity Interface
Unity's interface is where you'll spend most of your time while creating your game. It's divided into several sections, such as the Scene View, the Game View, the Hierarchy, the Inspector, and the Project Window. Familiarize yourself with these panels because they are your primary tools for game development.
Scene Creation
A Unity scene is like a container that holds all the game objects, such as characters, terrain, and lighting. Creating a new scene is as simple as navigating to File > New Scene
. Unity's default scene comes with a camera and a light source, but you can add more objects as needed.
Adding Game Objects
Game objects are the building blocks of your 3D world. To add a new game object, go to GameObject > 3D Object
and choose from the available options like cube, sphere, or plane. Once you've added an object, you can use the transform tools to move, rotate, or scale it in the Scene View.
Assets
Assets are the resources used in your game, such as models, textures, sounds, and scripts. You can create assets from scratch or import them from the Unity Asset Store. Once you have assets in your project, you can use the Project Window to organize them into folders and drag them into your scene.
Importing Assets
To import an existing asset, either drag it into the Project Window or go to Assets > Import New Asset
. Unity supports a wide range of file formats, so make sure you're using a compatible file type. When you import an asset, Unity may create additional files such as materials, textures, or animations, depending on the asset type.
Scripting
Scripting is the magic that brings your game to life. In Unity, you can write scripts using C#. These scripts control the behavior of game objects and handle events like user input or collisions.
Creating a Script
To create a new script, right-click in your Project Window and select Create > C# Script
. Give your script a descriptive name, and double-click it to open it in your preferred code editor.
Attaching Scripts to Game Objects
Once you've written your script, you need to attach it to a game object to make it work. You can do this by selecting the game object in the Hierarchy or Scene View and dragging the script from the Project Window to the Inspector.
Writing Your First Script
When you open a new script, Unity generates a basic template with the necessary using
statements and class definition. Inside the class, you'll find two methods: Start
and Update
. The Start
method runs once when the game object is initialized, while the Update
method runs every frame.
Here's a simple example of a script that moves a game object to the right over time:
using UnityEngine; public class MoveObject : MonoBehaviour { public float speed = 1.0f; void Update() { transform.Translate(Vector3.right * speed * Time.deltaTime); } }
In this example, we define a public speed
variable, which can be adjusted in the Inspector. Then, in the Update
method, we use the Translate
function to move the object along the X-axis, multiplied by the speed and Time.deltaTime
to ensure smooth movement across different frame rates.
Now that you've got a grasp on Unity's interface, scene creation, assets, and scripting, you're ready to start building your own 3D games! Remember, practice makes perfect - so keep experimenting and learning as you go. Good luck, and happy game developing!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Why Program? (psst, it's free!).
FAQ
What are the basics of creating a 3D game scene in Unity?
To create a 3D game scene in Unity, follow these steps:
- Open Unity and create a new 3D project.
- In the Hierarchy window, right-click and add various game objects like 3D models, lights, and cameras.
- Use the Scene view to position and scale game objects to create the desired environment.
- Create a Prefab by dragging a game object from the Hierarchy to the Project window, allowing you to reuse game objects with the same properties.
- Use the Inspector window to change properties and add components to game objects, such as physics, materials, and scripts.
How do I import and use assets in Unity 3D?
Importing and using assets in Unity is quite simple:
- Download or create assets like 3D models, textures, and audio files.
- Drag and drop the assets into the Project window, or use the import option from the 'Assets' menu.
- To use an asset in your scene, simply drag it from the Project window to the Hierarchy or the Scene view.
- Use the Inspector window to adjust the properties of the asset, such as scale, rotation, and materials.
How do I start scripting in Unity 3D?
To begin scripting in Unity, follow these steps:
- Create a new C# script from the 'Assets' menu or by right-clicking in the Project window.
- Name your script and open it in your preferred code editor (Unity supports Visual Studio and Visual Studio Code).
- Inside the script, you'll find basic functions like
Start()
andUpdate()
. These functions are called automatically by Unity during gameplay. - Write your custom code within these functions or create new functions to perform game logic.
- Save the script and attach it to a game object in the scene by dragging it from the Project window to the desired game object in the Hierarchy.
How can I add physics to my Unity 3D game?
To add physics to your game, do the following:
- Select the game object you want to apply physics to in the Hierarchy or Scene view.
- In the Inspector window, click 'Add Component' and search for Rigidbody or Rigidbody2D (for 2D games).
- Add a Collider component (e.g., BoxCollider, SphereCollider, or CapsuleCollider) to define the object's physical shape.
- Adjust the properties of the Rigidbody and Collider components as needed, such as mass, drag, and collision detection mode.
- Use scripting to apply forces, detect collisions, or control other aspects of the physics simulation.
What are some tips for optimizing performance in Unity 3D games?
To optimize performance in your Unity 3D game, consider the following tips:
- Use lower-poly models and efficient textures to reduce rendering complexity.
- Use Occlusion Culling to avoid rendering objects that are not visible to the camera.
- Optimize physics by using simpler colliders, reducing the number of physics interactions, and using layers to limit collision checks.
- Use Level of Detail (LOD) to display lower-quality models when objects are further away from the camera.
- Optimize your scripts by avoiding expensive operations in the
Update()
function and using object pooling to reduce instantiation overhead.