Unity 2D Game Development
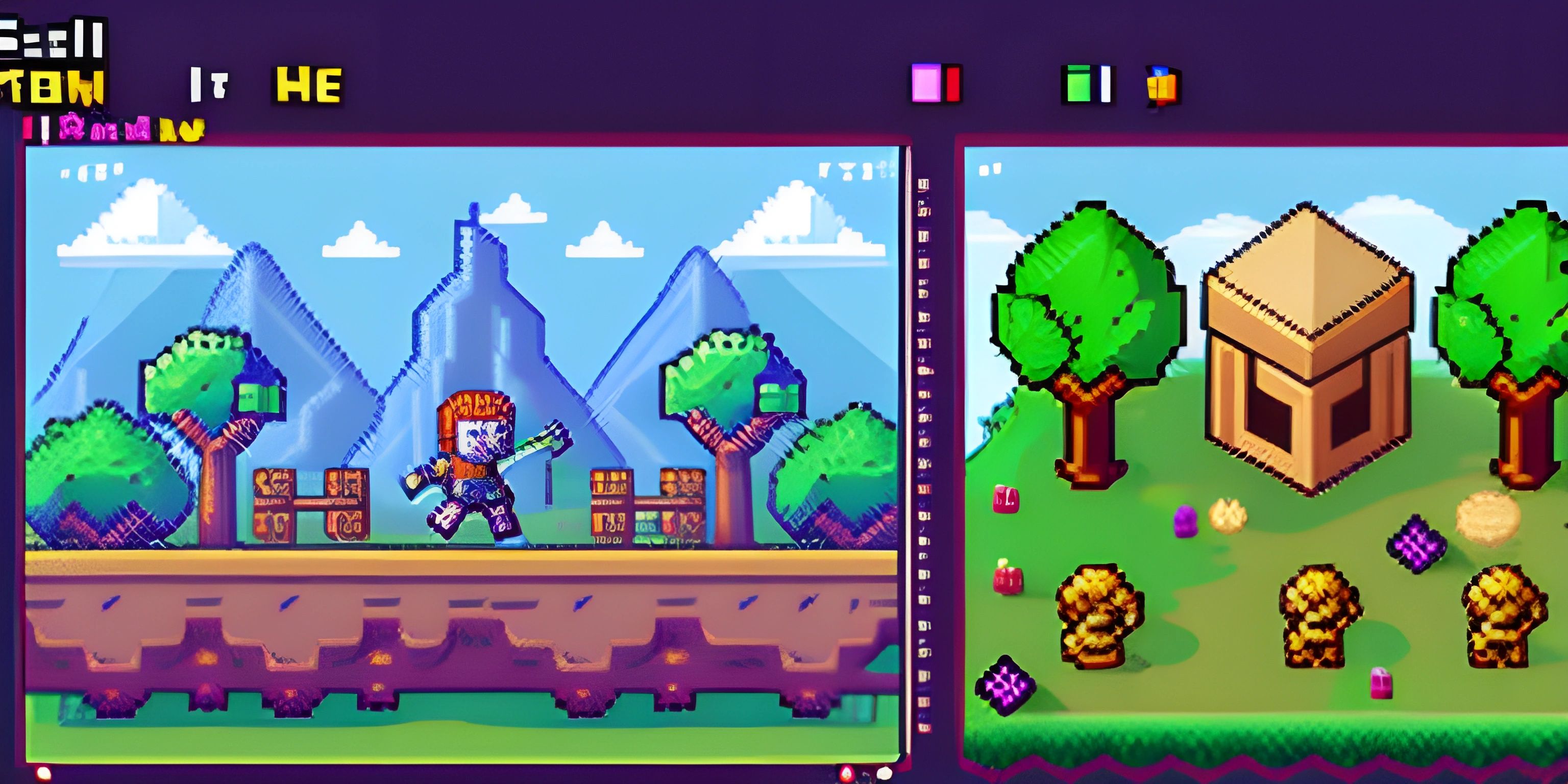
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Unity is a powerful game engine that allows developers to create both 2D and 3D games. In this article, we'll take a look at how to create a 2D game step by step.
Setting Up a Unity Project
-
Download and install Unity Hub: To begin, you need to download and install Unity Hub, a tool that helps manage your Unity projects and installations.
-
Create a new project: Open Unity Hub, click on the "New" button, and select "2D" as the template. Name your project and choose a location to save it.
-
Unity interface: Once the project is created, you'll be greeted by the Unity interface. Familiarize yourself with the main windows: Scene, Game, Hierarchy, Project, and Inspector.
Adding Game Elements
Sprites
Sprites are 2D images used to represent game objects. To create a sprite:
-
Import the image: Right-click in the Project window, select "Import New Asset," and choose the image file you want to use as a sprite.
-
Configure the sprite: Select the imported image in the Project window, and in the Inspector window, change the "Texture Type" to "Sprite (2D and UI)." Apply the changes.
-
Add the sprite to the scene: Drag the sprite from the Project window into the Scene or Hierarchy window. This creates a new GameObject with the sprite as its visual representation.
Physics
Unity has a built-in physics engine that makes it easy to create realistic movement and collisions. To enable physics for a GameObject:
-
Add a Rigidbody2D component: Select the GameObject in the Hierarchy, click "Add Component" in the Inspector, and search for "Rigidbody2D." This component allows the object to be affected by forces and gravity.
-
Add a Collider2D component: Similarly, add a Collider2D component (e.g., "Box Collider 2D" or "Circle Collider 2D") to the GameObject. This defines the object's physical shape for collisions.
Scripting
Scripts are used to control GameObjects and create game logic. Unity primarily uses C# for scripting.
Creating a Script
-
Add a new script: Right-click in the Project window, select "Create" > "C# Script," and name your script.
-
Edit the script: Double-click the script file in the Project window to open it in your preferred code editor (e.g., Visual Studio).
Writing Code
-
Accessing GameObjects: To access a GameObject in your script, you can use the
GameObject.Find
function or create a public variable and assign the GameObject in the Inspector. -
Using Components: To access a component on a GameObject, use the
GetComponent
function (e.g.,GetComponent<Rigidbody2D>()
). -
Handling Input: To detect player input, use the
Input
class, which has functions likeInput.GetKey
andInput.GetAxis
. -
Applying Forces: To move a Rigidbody2D using physics, apply forces to it using functions like
AddForce
andAddTorque
.
Here's an example of a simple player movement script:
using UnityEngine; public class PlayerMovement : MonoBehaviour { public float speed = 10f; private Rigidbody2D rb; void Start() { rb = GetComponent<Rigidbody2D>(); } void Update() { float horizontalInput = Input.GetAxis("Horizontal"); float verticalInput = Input.GetAxis("Vertical"); Vector2 movement = new Vector2(horizontalInput, verticalInput); rb.AddForce(movement * speed); } }
Attaching Scripts to GameObjects
To use your script, simply drag it onto the desired GameObject in the Hierarchy or click "Add Component" in the Inspector and search for your script's name.
With these basics in place, you're ready to dive deeper into Unity 2D game development. Explore more advanced topics, such as animation, UI, and level design. Happy game-making!