Unity C# Basics
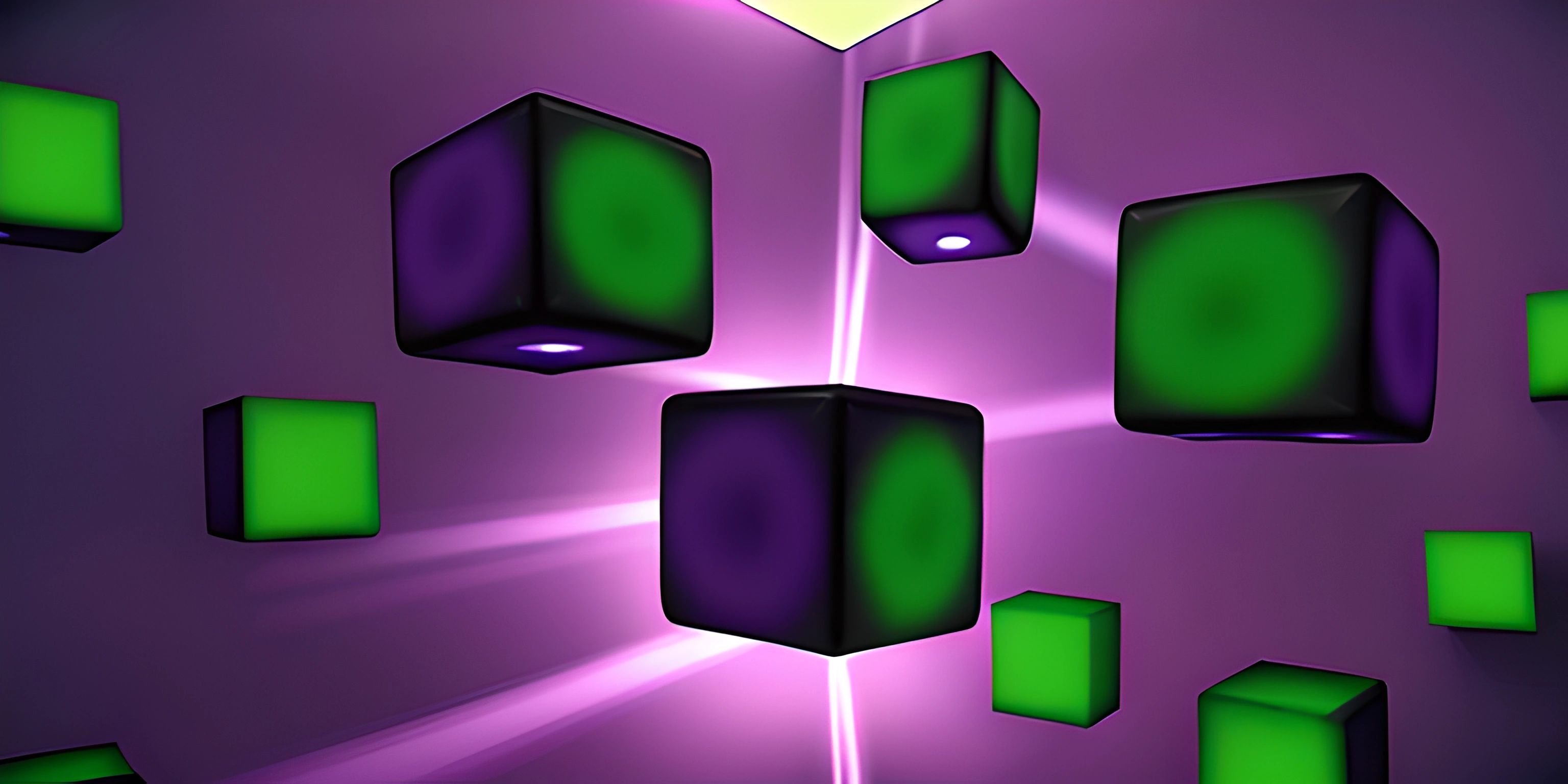
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Unity is a powerful game engine that allows you to create both 2D and 3D games across multiple platforms. But to bring your game to life, you'll need to understand the basics of programming with C#. C# is a versatile and popular programming language that Unity has chosen as its main scripting language. Let's dive into the essential concepts you'll need to know when working with C# in Unity.
Creating a Unity Script
In Unity, scripts are created as separate files, usually with the extension .cs
for C# scripts. To create a script in Unity, follow these steps:
- Right-click in your Project window.
- Select "Create" > "C# Script".
- Give your script a descriptive name, like "PlayerMovement".
After creating the script, double-click it to open it in your preferred code editor (Visual Studio or Visual Studio Code are common choices). You'll see a basic C# script template, which should look like this:
using UnityEngine; public class PlayerMovement : MonoBehaviour { void Start() { } void Update() { } }
This simple script is a great starting point for understanding the structure of a Unity C# script.
C# Script Structure
A typical Unity C# script includes the following components:
Namespaces
A namespace is a container that holds a group of related classes, interfaces, and other code elements. The using
directive at the top of the script allows you to access the contents of the specified namespace. In this case, using UnityEngine;
provides access to the Unity API and its classes, like MonoBehaviour
.
using UnityEngine;
Class Declaration
A class is a blueprint for creating objects in C#. In Unity, each script is a class derived from MonoBehaviour
, which is a base class provided by Unity for most game-related functionality. The class name should match the script file name.
public class PlayerMovement : MonoBehaviour
Methods
In C#, methods (also known as functions) are blocks of code that perform a specific task. Unity has some built-in methods that are automatically called at specific moments during your game's lifecycle. The Start()
method is called when the script is first enabled, while the Update()
method is called every frame, making it useful for handling real-time game logic.
void Start() { // Initialization code here } void Update() { // Per-frame logic here }
Adding Functionality
Now that you're familiar with the structure of a Unity C# script, let's add some functionality. For example, let's make a simple script that moves a game object when the player presses the spacebar.
void Update() { if (Input.GetKeyDown(KeyCode.Space)) { transform.position += new Vector3(1, 0, 0); } }
In the Update()
method, we check if the spacebar is pressed using Input.GetKeyDown(KeyCode.Space)
. If it is, we move the game object by adding a new Vector3
to its current position.
Attaching Scripts to Game Objects
To make your script work in your Unity scene, you'll need to attach it to a game object. Simply select the game object in the Unity hierarchy, then drag the script from the Project window onto the game object's Inspector panel. Alternatively, you can click "Add Component" in the Inspector panel and search for your script by its class name.
Now that you've learned the basics of C# programming in Unity, you're ready to start creating your own game scripts and bring your ideas to life. Happy coding!