Exploring the Unity API and Its Features
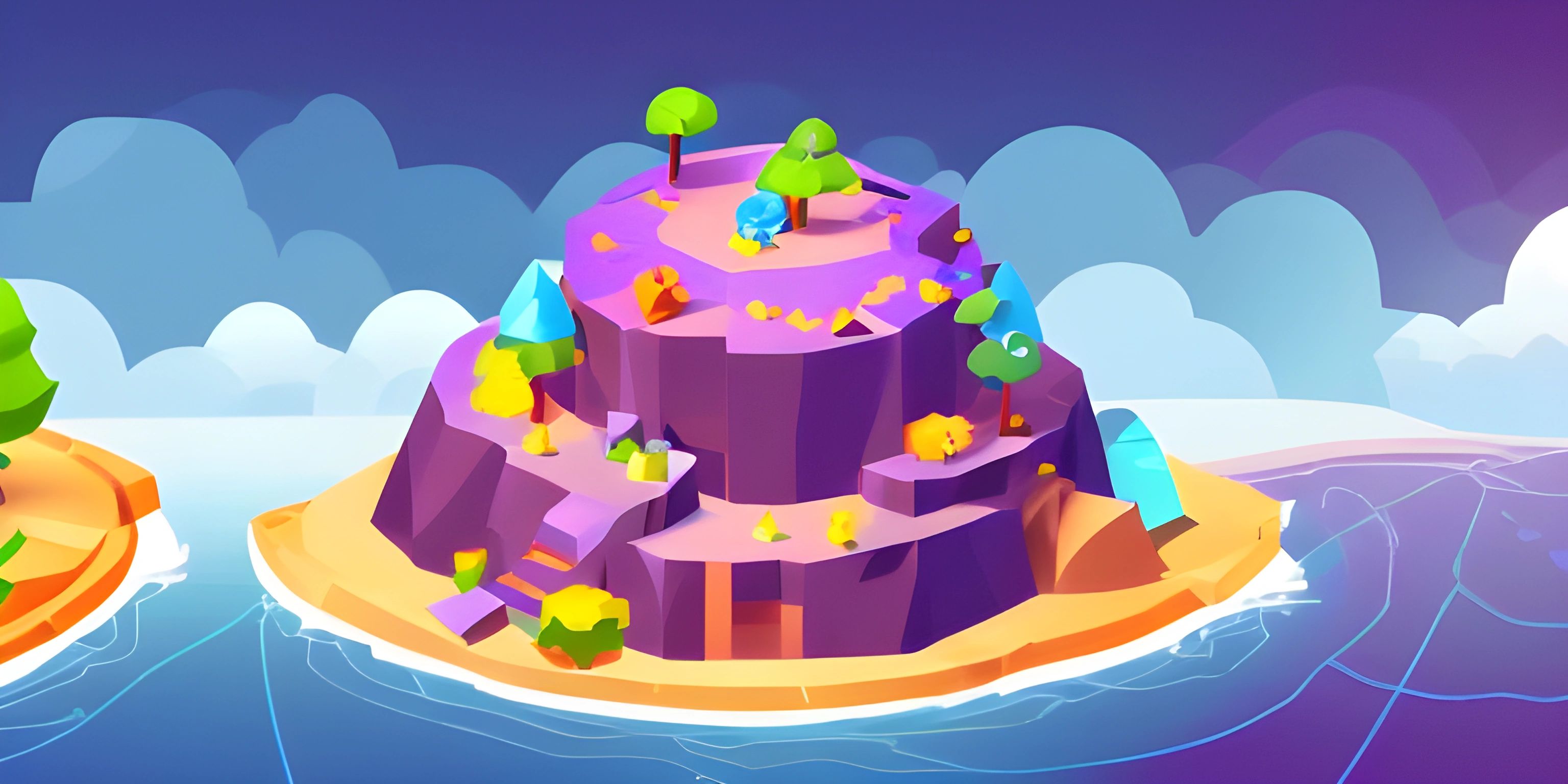
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
If you've ever wanted to create your own video game, chances are you've stumbled upon Unity. Unity is like the Swiss Army knife of game engines—it's powerful, versatile, and has a tool for just about everything. But what makes it really shine is its Application Programming Interface (API). The Unity API acts as a bridge between your code and the Unity engine, allowing you to bring your wildest game ideas to life.
What is an API?
Before we delve into Unity's specific API, let's break down what an API actually is. API stands for Application Programming Interface. Think of it like a menu at a restaurant. The menu provides a list of dishes you can order along with a description of each dish. When you specify what you want, the kitchen (i.e., the backend) takes your order and delivers the dish to your table. In this analogy, the Unity API is the menu, your code is the order, and the Unity engine is the kitchen that prepares and serves the dish.
Getting Started with Unity
To use the Unity API, you first need to set up the Unity environment. You'll also need a working knowledge of C#, as that's the primary language used for scripting in Unity.
- Download and Install Unity: Head over to the Unity website and download the latest version. Installation steps are straightforward.
- Open a New Project: Once Unity is installed, open it and create a new project. Choose the 3D or 2D template based on your game idea.
- Set Up Your Scene: Unity comes with a default scene that includes a Camera and Light. You can add more objects to the scene to create your game world.
Key Components of the Unity API
GameObjects and Components
In Unity, everything you see in your game is a GameObject. A GameObject can represent characters, props, scenery—basically anything that exists in your game world.
Components are the building blocks of GameObjects. Think of them like the different parts of a car: the engine, the wheels, the seats, etc. Each component adds functionality to the GameObject. For example, a Rigidbody component would add physics properties to a GameObject, making it respond to gravity and collisions.
Here's a simple example of creating a GameObject and adding a component to it:
using UnityEngine; public class ExampleScript : MonoBehaviour { void Start() { // Create a new GameObject GameObject cube = GameObject.CreatePrimitive(PrimitiveType.Cube); // Add a Rigidbody component to the cube cube.AddComponent<Rigidbody>(); // Set the position of the cube cube.transform.position = new Vector3(0, 5, 0); } }
MonoBehaviour
MonoBehaviour is the base class from which every Unity script derives. It provides numerous methods and properties that you can use to control the behavior of GameObjects. Some of the most commonly used methods include:
Start()
: Called before the first frame update.Update()
: Called once per frame.FixedUpdate()
: Called at a fixed interval, useful for physics calculations.OnCollisionEnter()
: Called when a collision occurs.
Here's a quick example of a script that moves a GameObject when the arrow keys are pressed:
using UnityEngine; public class MoveObject : MonoBehaviour { public float speed = 10f; void Update() { float moveHorizontal = Input.GetAxis("Horizontal"); float moveVertical = Input.GetAxis("Vertical"); Vector3 movement = new Vector3(moveHorizontal, 0.0f, moveVertical); transform.Translate(movement * speed * Time.deltaTime); } }
Prefabs
Prefabs are like cookie cutters for GameObjects. They allow you to create, configure, and store a GameObject complete with all its components and properties, and then reuse it throughout your game. Think of it as a template that you can instantiate multiple times.
To create a prefab:
- Create a GameObject in the scene.
- Configure it with all the necessary components and settings.
- Drag the GameObject from the Hierarchy into the Project window.
You can then instantiate the prefab in your scripts:
using UnityEngine; public class SpawnObject : MonoBehaviour { public GameObject prefab; public int numberOfObjects = 10; void Start() { for (int i = 0; i < numberOfObjects; i++) { Instantiate(prefab, new Vector3(i * 2.0F, 0, 0), Quaternion.identity); } } }
Events and Delegates
Unity uses events and delegates to handle input, collision, and other actions that require a response. For instance, you might want to trigger an event when a player collects a coin or when an enemy is defeated.
Here's a simple example of using events and delegates:
using UnityEngine; public class Coin : MonoBehaviour { public delegate void CoinCollected(); public static event CoinCollected OnCoinCollected; void OnTriggerEnter(Collider other) { if (other.CompareTag("Player")) { OnCoinCollected?.Invoke(); Destroy(gameObject); } } } public class Player : MonoBehaviour { void OnEnable() { Coin.OnCoinCollected += CoinCollected; } void OnDisable() { Coin.OnCoinCollected -= CoinCollected; } void CoinCollected() { Debug.Log("Coin collected!"); } }
Debugging and Troubleshooting
Unity provides several tools for debugging your game. The Console window is invaluable for catching errors and logging messages. You can use Debug.Log
, Debug.Warning
, and Debug.Error
to output messages to the console:
using UnityEngine; public class DebugExample : MonoBehaviour { void Start() { Debug.Log("This is a log message."); Debug.LogWarning("This is a warning message."); Debug.LogError("This is an error message."); } }
Conclusion
The Unity API is a powerhouse that enables you to create complex and engaging games. From GameObjects and components to prefabs and events, the API provides all the tools you need to bring your game to life. By understanding and utilizing these features, you can turn your game ideas into reality.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Full-stack Web Frameworks (Next.js) (psst, it's free!).
FAQ
What is a GameObject in Unity?
A GameObject in Unity is the fundamental building block for creating objects in your game world. It can represent anything from characters to scenery. Components can be added to GameObjects to give them functionality.
How do you create a GameObject in Unity?
You can create a GameObject in Unity using the GameObject.CreatePrimitive
method. For example, GameObject cube = GameObject.CreatePrimitive(PrimitiveType.Cube);
creates a cube GameObject.
What is a MonoBehaviour in Unity?
MonoBehaviour is the base class from which all scripts in Unity derive. It provides essential methods like Start()
, Update()
, and FixedUpdate()
to control the behavior of GameObjects.
What is a Prefab in Unity?
A Prefab is a pre-configured GameObject that can be reused throughout your game. You can create a prefab by dragging a GameObject from the Hierarchy into the Project window and then instantiate it in your scripts.
How do you handle events in Unity?
Unity handles events using delegates and events. You can define a delegate and an event, and then invoke the event when a specific action occurs. Other scripts can subscribe to the event and execute their methods when it is triggered.