Variables in Programming
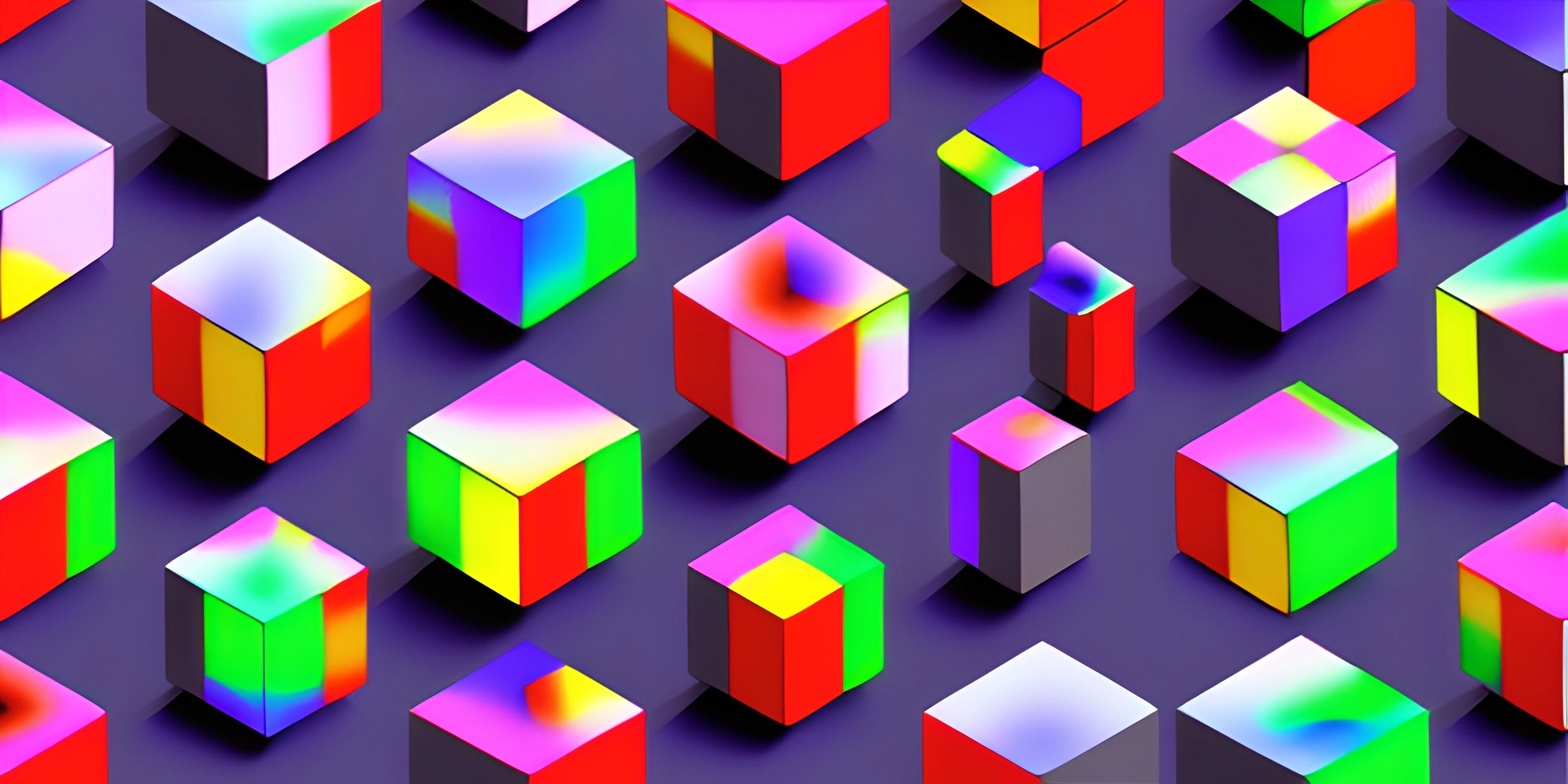
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
When learning how to program, one of the first things you'll encounter is the concept of variables. Think of them as little containers used to store and manage data throughout your program. Without them, coding would be like trying to cook a feast without any pots or pans!
What is a Variable?
A variable is a named storage location in a program that holds a value. You can think of it as a label on a box, where the box can contain different items (values) at different times.
Variables allow us to store, change, and retrieve data throughout a program's execution. They are essential because they provide a way to represent and manipulate data in a meaningful and efficient manner.
Declaring a Variable
To create a variable, you must first declare it. Declaring a variable means telling the program that you want to reserve memory space to store a value. This is usually done by specifying the variable's name and data type. The syntax for declaring a variable varies depending on the programming language you're using. For example, in JavaScript, you can declare a variable like this:
let age;
In this case, we've declared a variable named age
. The let
keyword is used to declare a variable in JavaScript.
Assigning a Value to a Variable
Once you've declared a variable, you can assign a value to it. This is like placing an item inside the box we mentioned earlier. Here's how we can assign a value to the age
variable in JavaScript:
age = 25;
Now, the variable age
holds the value 25
. In many programming languages, you can declare and assign a variable in a single line, like this:
let age = 25;
Accessing a Variable's Value
To use the value stored in a variable, you simply write the variable's name in your code. When the program encounters the name, it will replace it with the value it holds. Here's an example:
let age = 25; console.log("My age is " + age);
This code will output My age is 25
. The console.log()
function prints the message to the console, and the +
operator concatenates the string with the value of age
.
Variable Types and Scope
Variables can store different types of data, such as numbers, strings, or even more complex data structures like arrays and objects. The specific data types available depend on the programming language you are using.
Another important concept related to variables is scope. Scope determines where a variable can be accessed and modified in your program. There are generally two types of scope: global scope and local scope. Global variables can be accessed from anywhere in the program, while local variables are only accessible within the function or block of code they are declared in.
Understanding variables, their types, and scope is crucial for effective programming. As you gain more experience, you'll learn how to use variables efficiently to create powerful and maintainable code. Remember, variables are like the pots and pans of programming – the more you learn to use them effectively, the better your code will be!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).