Understanding Variables and Data Types in JavaScript
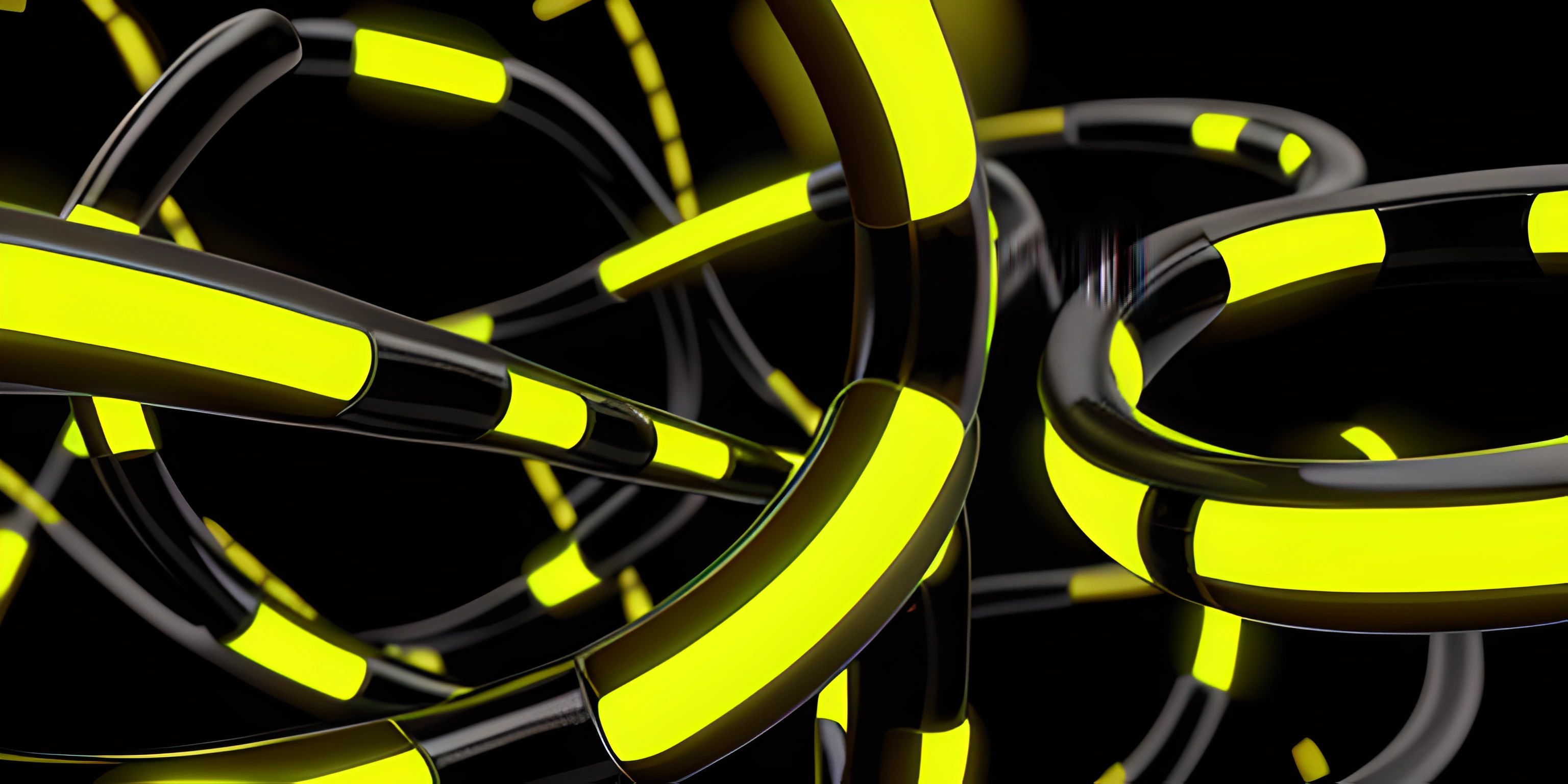
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
In JavaScript, variables are used to store data that can be used and manipulated throughout your code. Data types help define the kind of data stored in a variable. In this guide, we'll cover the basics of variables and the different data types available in JavaScript.
Variables
Variables are containers for storing data values. In JavaScript, you can declare a variable using the var
, let
, or const
keyword.
var
: This keyword is used to declare a variable in older JavaScript code. It has function scope, which means the variable is accessible only within the function it is declared in.let
: This keyword is used to declare a variable with block scope, which means the variable is only accessible within the block it is declared in. This is recommended for modern JavaScript code.const
: This keyword is used to declare a constant variable, which means its value cannot be changed after it is assigned.
var age = 30; let name = "John"; const pi = 3.14159;
Data Types
JavaScript has several different data types that can be stored in variables. These include:
Primitive Data Types
- Number: Represents both integers and floating-point numbers.
let num1 = 42; let num2 = 3.14;
- String: Represents a sequence of characters.
let greeting = "Hello, World!";
- Boolean: Represents a true or false value.
let isCoding = true;
- Null: Represents an intentionally empty value.
let emptyValue = null;
- Undefined: Represents a variable that has been declared but not assigned a value.
let notAssigned; console.log(notAssigned); // Output: undefined
- Symbol: Represents a unique, immutable value that can be used as an object property.
let symbol1 = Symbol("key"); let symbol2 = Symbol("key"); console.log(symbol1 === symbol2); // Output: false
Non-Primitive Data Types
- Object: Represents a collection of properties and methods.
let person = { name: "John", age: 30, greet: function() { console.log("Hello, my name is " + this.name); } };
- Array: Represents an ordered list of elements.
let fruits = ["apple", "banana", "orange"];
- Function: Represents a reusable block of code that can be called with arguments and return a value.
function add(x, y) { return x + y; }
Understanding variables and data types is essential for working with JavaScript. As you continue learning, you'll become more familiar with how to use and manipulate these data types in your code.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Syntax (psst, it's free!).
FAQ
What are the different data types in JavaScript?
JavaScript has several data types, including:
- String: A sequence of characters, like "Hello, world!"
- Number: Numeric values, such as 42 or 3.14
- Boolean: True or false values
- Object: A collection of key-value pairs
- Array: A list of values, like [1, 2, 3]
- Undefined: A variable that hasn't been assigned a value
- Null: Represents an intentional absence of any value
How do I declare and assign a value to a variable in JavaScript?
In JavaScript, you can declare and assign a value to a variable using the let
or const
keyword followed by the variable name, an equals sign, the value, and then a semicolon. For example:
let greeting = "Hello, world!"; const pi = 3.14159;
Use let
when you plan to change the value of the variable later in your code. Use const
when you want to make the value constant and unchangeable.
How can I find out the data type of a variable in JavaScript?
You can use the typeof
operator to find out the data type of a variable in JavaScript. For example:
let age = 25; console.log(typeof age); // Output: 'number'
The typeof
operator returns a string representing the data type.
Can I change the data type of a variable in JavaScript?
Yes, JavaScript is a dynamically-typed language, which means you can change the data type of a variable during runtime. For example:
let value = 42; console.log(typeof value); // Output: 'number' value = "Hello!"; console.log(typeof value); // Output: 'string'
In this example, the value
variable's data type changes from 'number' to 'string'.
How do I convert a string to a number in JavaScript?
There are a few ways to convert a string to a number in JavaScript, such as using parseInt()
, parseFloat()
, or the unary +
operator. Here are some examples:
let stringNumber = "42"; let intNumber = parseInt(stringNumber); console.log(intNumber); // Output: 42 let stringFloat = "3.14"; let floatNumber = parseFloat(stringFloat); console.log(floatNumber); // Output: 3.14 let stringNumber2 = "56"; let intNumber2 = +stringNumber2; console.log(intNumber2); // Output: 56