Exploring Java Data Types and How to Use Them
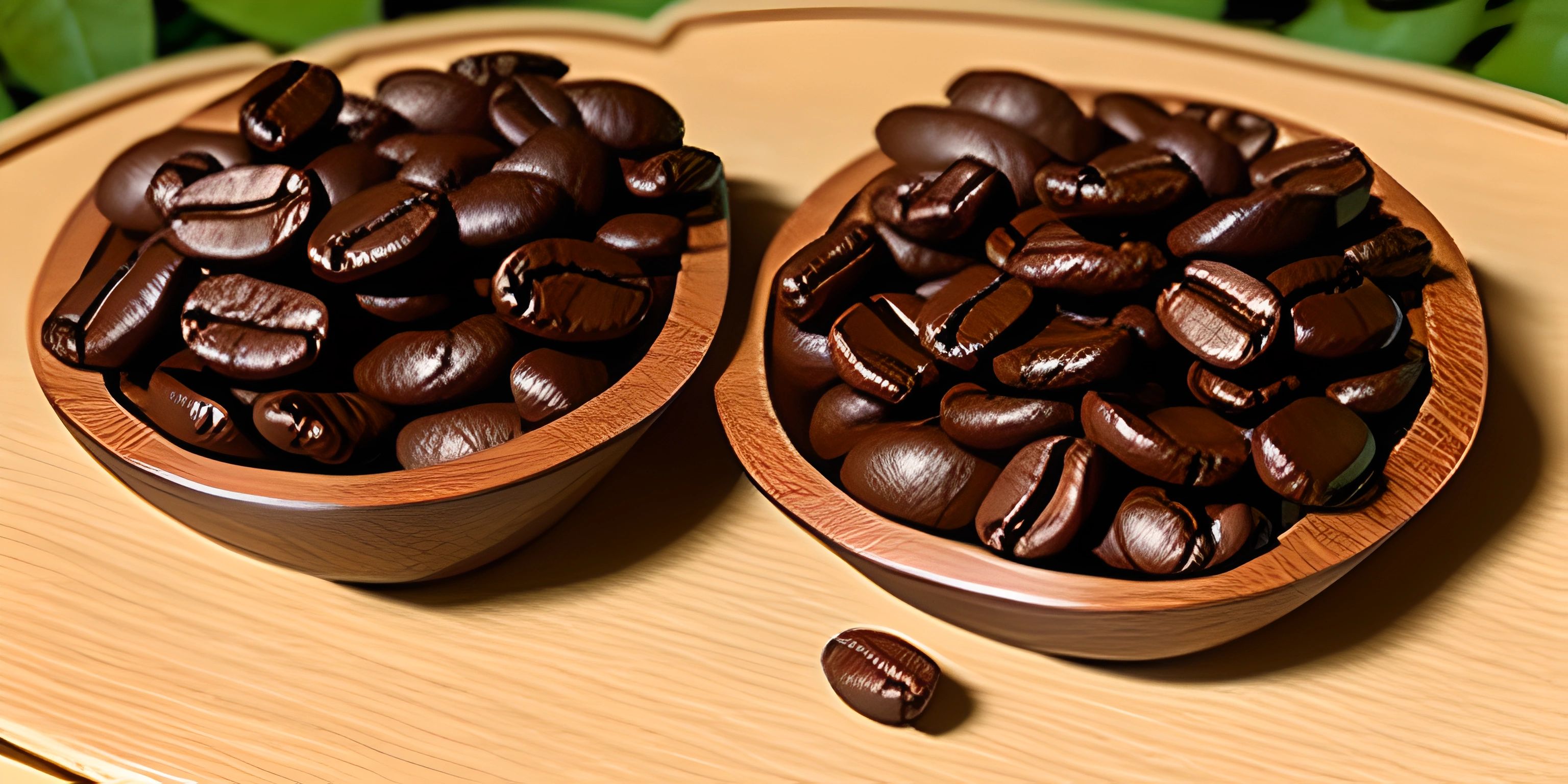
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Java is a versatile and powerful programming language, and one of the things that make it so powerful is its rich data type system. In Java, we have several built-in data types to store and manipulate different types of values. These data types are essential for defining variables, creating arrays, and designing functions, among many other things.
Primitive Data Types
Java has eight primitive data types. They are called primitive because they are the most basic data types, and all other data types are built on top of these. Let's dive into each one of them:
int
An int
represents a whole number, like 42 or -7. It can store values in the range of -2,147,483,648 to 2,147,483,647. Here's an example of declaring an int
variable:
int myNumber = 42;
double
A double
represents a decimal number (a number with a decimal point), like 3.14 or -0.5. Here's an example of declaring a double
variable:
double pi = 3.14;
boolean
A boolean
represents a true or false value. It is useful for controlling the flow of your program, like in conditional statements and loops. Here's an example:
boolean isHappy = true;
char
A char
represents a single character, like 'a' or 'Z'. It stores the Unicode value of the character. Here's an example:
char myInitial = 'J';
byte
, short
, and long
These data types are similar to int
, but they have different ranges of values they can store. They are used for optimizing memory usage when you know the range of values your variable will take. Here are the ranges and examples:
byte
: -128 to 127
byte smallNumber = 100;
short
: -32,768 to 32,767
short mediumNumber = 30000;
long
: -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807
long bigNumber = 10000000000L;
float
A float
is similar to a double
but with less precision. It is used when you need to save memory but still require decimal values. Here's an example:
float smallerPi = 3.14f;
Reference Data Types
In addition to primitive data types, Java also has reference data types. These are more complex data types, such as classes, interfaces, and arrays. The most common reference data type you'll encounter is String
, which represents a sequence of characters.
String myName = "John Doe";
Reference data types are a whole new world on their own, and we'll explore more about them in another article, so stay tuned!
In conclusion, understanding Java's data types and their usage is fundamental to writing efficient and effective programs. Keep these data types in mind and practice using them in your projects to create fully functional and well-optimized applications.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Data Types (psst, it's free!).
FAQ
What are the different data types available in Java?
Java has two main categories of data types: primitive data types and reference data types. The primitive data types in Java include:
byte
: 8-bit integershort
: 16-bit integerint
: 32-bit integerlong
: 64-bit integerfloat
: 32-bit floating-point numberdouble
: 64-bit floating-point numberchar
: 16-bit Unicode characterboolean
: true or false value Reference data types, on the other hand, include classes, interfaces, and arrays. These are created using thenew
keyword and are stored as references (memory addresses) rather than actual values.
How do I declare and initialize a variable using a specific data type in Java?
To declare and initialize a variable in Java, you need to specify the data type, followed by the variable name, an equals sign, the value, and finally a semicolon. Here's an example of declaring and initializing an integer variable:
int myNumber = 42;
What is type casting in Java and how do I use it?
Type casting in Java is the process of converting a value from one data type to another. There are two types of casting: implicit casting (automatic) and explicit casting (manual). Implicit casting occurs when you assign a value of a smaller data type to a larger one, for example:
int intValue = 42; double doubleValue = intValue; // Implicit casting from int to double
Explicit casting is necessary when you want to convert a value of a larger data type to a smaller one, and it's done by specifying the target data type in parentheses before the value:
double doubleValue = 42.5; int intValue = (int) doubleValue; // Explicit casting from double to int
How do I work with character data types in Java?
The char
data type is used to store a single Unicode character in Java. You can declare and initialize a character variable by enclosing the character in single quotes:
char myChar = 'A';
To work with multiple characters or strings, you can use the String
reference data type:
String myString = "Hello, World!";
What's the difference between `float` and `double` data types in Java?
Both float
and double
are used to represent floating-point numbers in Java, but they have different levels of precision and storage sizes. The float
data type has a 32-bit storage size and a precision of about 7 decimal digits, while the double
data type has a 64-bit storage size and a precision of about 15 decimal digits. By default, Java treats decimal numbers as double
. To declare a float
variable, you need to append an F
or f
after the number:
float myFloat = 3.14F; double myDouble = 3.14;