Basic Programming Concepts
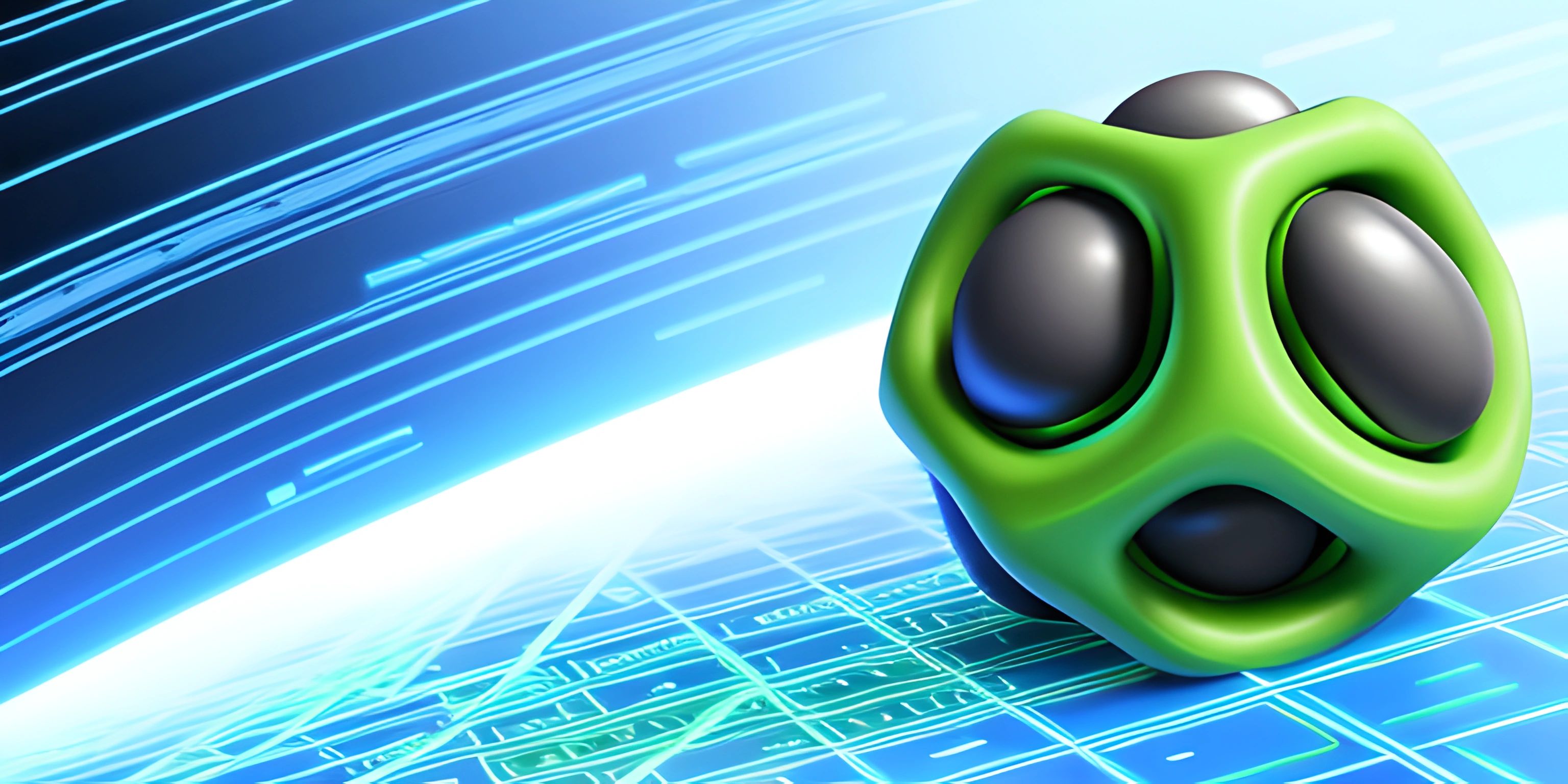
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Embarking on a programming journey requires a solid grasp of the foundational concepts that serve as the building blocks of any programming language. Let's dive into some of these essential ideas and get you ready to code like a pro!
Loops
In programming, loops are used to repeatedly execute a block of code until a specified condition is met. This is incredibly useful when you want to perform a certain task multiple times without duplicating code. There are two main types of loops: for loops and while loops.
For Loops
A for loop executes a block of code for a predetermined number of iterations. It usually consists of a counter, a condition, and an incrementation step. Here's an example in pseudocode:
for i = 1 to 5 print i
This code will print the numbers 1 through 5, one at a time.
While Loops
A while loop continues to execute a block of code as long as a specific condition remains true. Here's an example in pseudocode:
counter = 1 while counter <= 5 print counter counter = counter + 1
This code will also print the numbers 1 through 5, but it does so using a while loop instead of a for loop.
Conditions
Conditions are at the heart of decision-making in programming. They allow your code to execute different paths depending on whether specific criteria are met. The most common way to use conditions is with if statements.
If Statements
An if statement checks if a condition is true, and if so, executes a block of code. You can also use else if to check additional conditions, and else to execute code when none of the specified conditions are met. Here's a pseudocode example:
age = 18 if age >= 21 print "You can drink alcohol" else if age >= 18 print "You can vote, but not drink" else print "You are too young to vote or drink"
This code checks the age and prints an appropriate message based on the value.
Data Types
In programming, there are various data types to represent different kinds of information. Some of the most common data types include:
- Numbers: integers (whole numbers) and floating-point numbers (decimals)
- Strings: sequences of characters, like "Hello, world!"
- Booleans: true or false values, used for conditions
- Arrays: collections of elements, often of the same data type
- Objects: complex data structures that have properties and methods
Understanding data types and their respective uses is crucial for efficient and accurate programming.
With a firm understanding of loops, conditions, and data types, you're well on your way to mastering the basics of programming. Keep exploring, and happy coding!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Basic Concepts (psst, it's free!).
FAQ
What are the fundamental programming concepts every beginner should know?
The basic programming concepts every beginner should understand are:
- Variables: Storage units for data in a program, which can be assigned values and manipulated as needed.
- Data types: The classification of data into different categories, such as integers, floats, strings, and booleans.
- Loops: Structures that allow code to be executed repeatedly based on a condition, such as
for
andwhile
loops. - Conditional statements: Statements that execute code based on specific conditions being met, like
if
,elif
, andelse
. - Functions: Reusable blocks of code that perform a specific task and can be called with input parameters.
How do loops work in programming?
Loops in programming are used to execute a block of code repeatedly based on a condition. There are two primary types of loops:
- For loops: These loops iterate over a sequence (like a list, tuple, or string) and execute the code block for each item in the sequence. For example:
for i in range(5): print(i)
This code prints numbers 0 to 4.
- While loops: These loops execute the code block as long as a specified condition remains true. For example:
count = 0 while count < 5: print(count) count += 1
This code also prints numbers 0 to 4.
What are the different data types in programming?
Data types are classifications of data based on their characteristics. Common data types include:
- Integers: These are whole numbers without decimals, such as 42, -7, and 0.
- Floats: These are numbers with decimals, such as 3.14, -0.5, and 7.0.
- Strings: These are sequences of characters, like "hello," "world," and "123".
- Booleans: These represent true/false values and are often used in conditional statements. Some languages also have more complex data types, like lists, tuples, and dictionaries, which allow you to work with collections of data.
How do conditional statements work in programming?
Conditional statements are used to execute different code blocks based on specific conditions being met. The most common types of conditional statements are if
, elif
, and else
. Here's an example:
num = 5 if num > 0: print("The number is positive.") elif num < 0: print("The number is negative.") else: print("The number is zero.")
In this example, since num
is 5, the output will be "The number is positive."
What is the purpose of functions in programming?
Functions are reusable blocks of code that serve a specific purpose and can be called multiple times with different input parameters. Functions make code more organized, modular, and easy to maintain. Here's an example of a simple function:
def add_numbers(a, b): return a + b result = add_numbers(3, 4) print(result) # Output: 7
In this example, the add_numbers
function takes two parameters (a
and b
) and returns their sum. The function can be called with different values to perform the same task multiple times.