Understanding Aliasing in Graphics
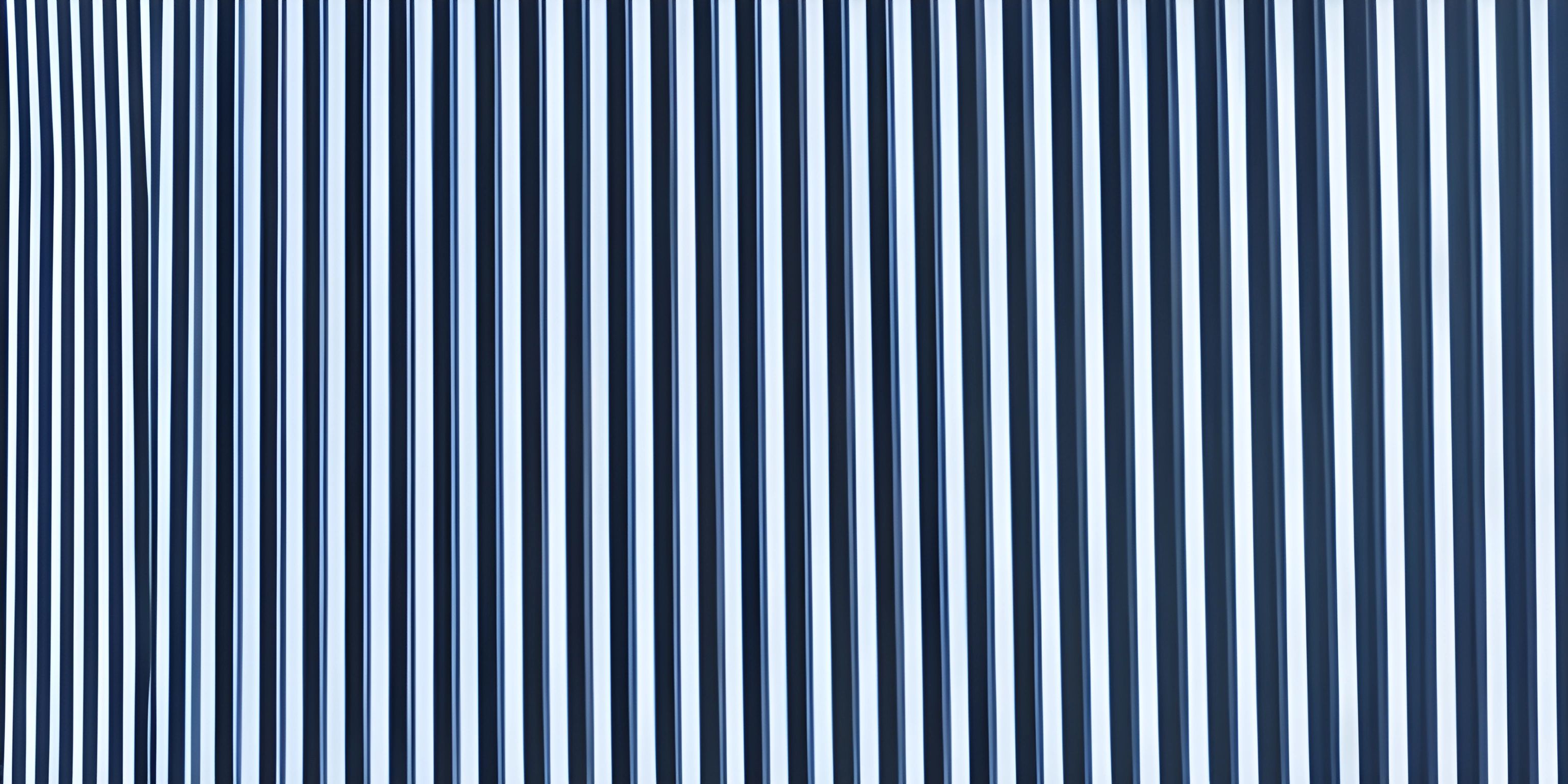
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Imagine looking at a beautiful digital image, but instead of smooth edges, you see jagged, pixelated lines. This unsightly phenomenon is known as aliasing, and it’s the bane of every graphic designer's existence. Fear not, for understanding aliasing and how to combat it will help you create cleaner, more professional visuals.
What is Aliasing?
Aliasing occurs when a high-resolution image or signal is sampled at a lower resolution, causing distortion or artifacts. This happens because the sampling rate is insufficient to capture the details of the original image. In simpler terms, it's like trying to recreate the Mona Lisa with a box of crayons—you’re bound to lose some detail!
The Staircase Effect
One of the most common manifestations of aliasing in graphics is the "staircase effect," where diagonal lines appear jagged, as if they were made of tiny steps. This visual glitch is particularly noticeable in computer-generated imagery (CGI), where lines and edges should ideally be smooth and continuous.
# Example of aliasing in a simple chart import matplotlib.pyplot as plt # Create some data with a smooth curve x = list(range(100)) y = [val**0.5 for val in x] # Plot the data with aliasing plt.plot(x, y) plt.title("Aliasing Example: Staircase Effect") plt.show()
In this example, plotting a smooth curve can result in a jagged appearance due to aliasing, especially if the resolution of the display is low.
Anti-Aliasing Techniques
To combat aliasing, various anti-aliasing techniques have been developed. These methods aim to smooth out jagged edges and improve the overall quality of digital images. Here are a few common techniques:
Supersampling
Supersampling involves rendering the image at a higher resolution and then downscaling it to the desired size. This process effectively smooths out the edges by averaging the colors of multiple pixels.
// Pseudocode for supersampling int targetWidth = 1920; int targetHeight = 1080; // Render at a higher resolution int superWidth = targetWidth * 2; int superHeight = targetHeight * 2; // Downscale to the target resolution renderImage(superWidth, superHeight); downscaleImage(targetWidth, targetHeight);
Supersampling is highly effective but computationally expensive, as it requires rendering more pixels than the final output.
Multisample Anti-Aliasing (MSAA)
MSAA is a more efficient alternative to supersampling. It samples multiple points within each pixel and averages the results, reducing jagged edges without the need for full-resolution rendering.
// Example of MSAA setup in OpenGL glEnable(GL_MULTISAMPLE); glHint(GL_MULTISAMPLE_FILTER_HINT_NV, GL_NICEST);
MSAA strikes a balance between performance and quality, making it a popular choice in real-time applications like video games.
Post-Processing Filters
Post-processing filters, such as FXAA (Fast Approximate Anti-Aliasing), are applied after the image is rendered. These filters analyze the rendered image and adjust pixel colors to smooth out edges.
// GLSL shader example for FXAA #version 330 core uniform sampler2D screenTexture; void main() { vec3 color = texture(screenTexture, gl_FragCoord.xy).rgb; // Apply FXAA algorithm to smooth edges color = applyFXAA(color); gl_FragColor = vec4(color, 1.0); }
Post-processing filters are generally faster than supersampling and MSAA but may not be as effective in preserving image detail.
Aliasing in Different Contexts
Aliasing isn't limited to graphics. It can occur in various areas, such as audio processing and signal transmission. However, the principles and techniques to address it remain largely similar.
Aliasing in Audio
In audio processing, aliasing occurs when sound waves are sampled at a rate that is too low, leading to distortion. The solution involves using a higher sampling rate and applying anti-aliasing filters to remove unwanted frequencies.
import numpy as np import matplotlib.pyplot as plt # Create a sine wave with a high frequency sampling_rate = 8000 t = np.linspace(0, 1, sampling_rate, endpoint=False) freq = 3000 # Frequency in Hz audio_signal = np.sin(2 * np.pi * freq * t) # Plot the signal plt.plot(t, audio_signal) plt.title("Aliasing in Audio Signal") plt.show()
This example demonstrates how a high-frequency sine wave can exhibit aliasing when sampled at a low rate.
Practical Tips to Reduce Aliasing
Choose the Right Resolution
Selecting an appropriate resolution for your images or rendering can significantly reduce aliasing. Higher resolutions capture more detail, minimizing the jagged edges.
Use Anti-Aliasing Techniques
Incorporate anti-aliasing techniques like supersampling, MSAA, or post-processing filters in your workflow to smooth out edges and improve visual quality.
Optimize Performance
Balancing quality and performance is crucial, especially in real-time applications like gaming. Choose anti-aliasing techniques that offer the best trade-off for your specific use case.
Conclusion
Aliasing can be a pesky problem, but understanding its causes and solutions will help you produce smoother, more professional graphics. Whether you’re working on digital art, video games, or any other visual medium, implementing the right anti-aliasing techniques can make a world of difference.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Full-stack Web Frameworks (Next.js) (psst, it's free!).
FAQ
What is aliasing in graphics?
Aliasing in graphics occurs when a high-resolution image is sampled at a lower resolution, causing jagged edges and visual artifacts. It's commonly seen as the "staircase effect" in diagonal lines.
What are some common anti-aliasing techniques?
Common anti-aliasing techniques include supersampling, multisample anti-aliasing (MSAA), and post-processing filters like FXAA. Each method has its strengths and weaknesses in terms of computational cost and image quality.
Is aliasing only a problem in graphics?
No, aliasing can occur in various fields, including audio processing and signal transmission. The underlying issue is the insufficient sampling rate to capture the original detail accurately.
How does supersampling work?
Supersampling works by rendering an image at a higher resolution than needed and then downscaling it to the target size. This process smooths out edges by averaging the colors of multiple pixels.
Why is anti-aliasing important in video games?
Anti-aliasing is crucial in video games to improve visual quality and provide a more immersive experience. Jagged edges and visual artifacts can be distracting, reducing the overall enjoyment of the game.