Building a Voxel Engine in Python
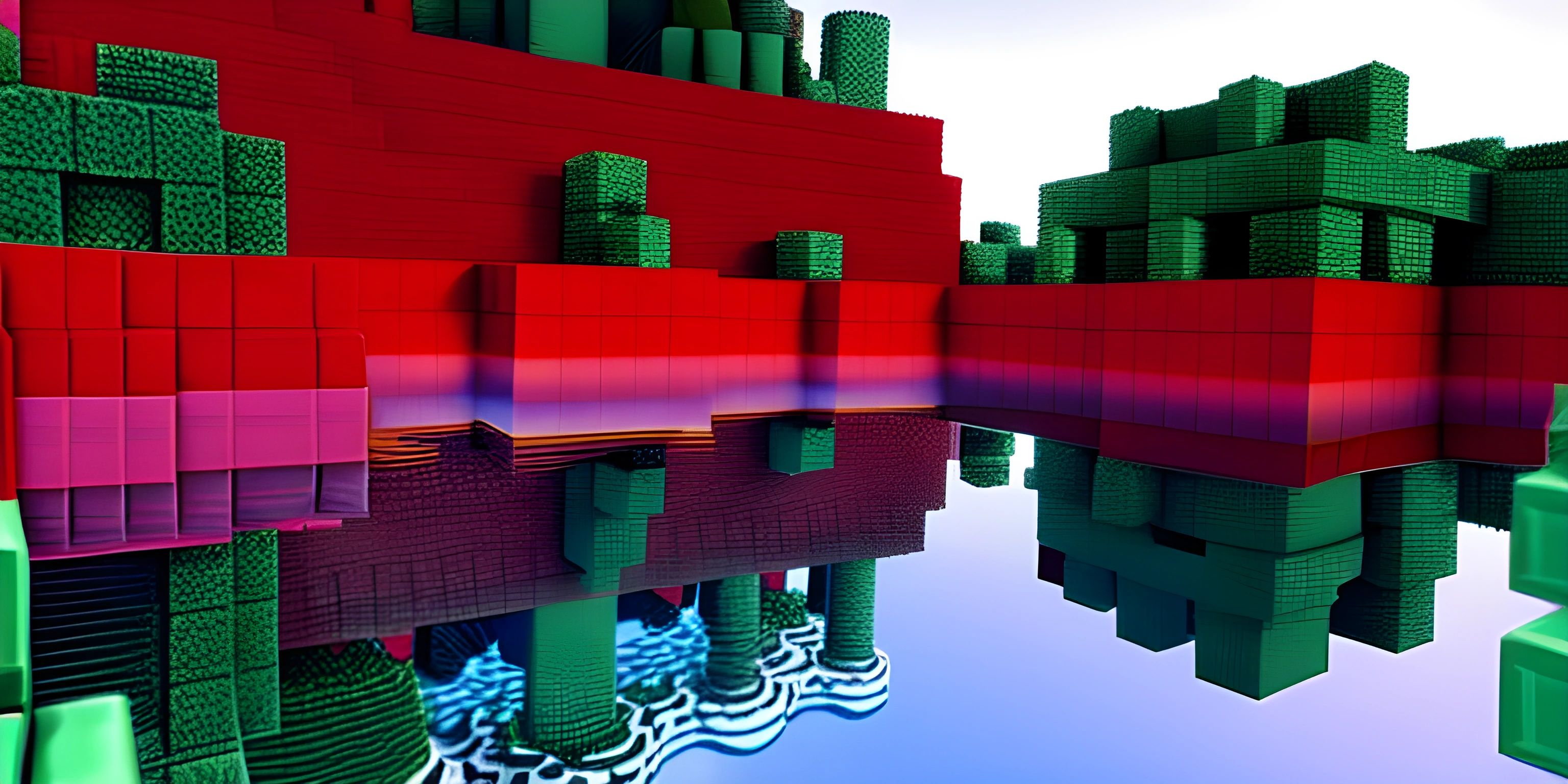
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Ever wondered how those blocky landscapes in games like Minecraft are created? Welcome to the magical world of voxel engines! In this article, we’ll explore how voxel engines work and walk through building one using Python. So, grab your pickaxe and let's dig in!
What Are Voxels?
First things first, let's define what a voxel is. A voxel, short for "volumetric pixel," is basically a 3D equivalent of a 2D pixel. Imagine a cube; that's a voxel. By stacking these tiny cubes together, we can create complex 3D landscapes.
The Basics of Voxel Engines
A voxel engine is the software that generates and manages these 3D cubes. It's responsible for rendering, updating, and even simulating interactions between voxels. Think of it as the brain behind the beautiful blocky worlds you see in voxel-based games.
Why Use Voxels?
Voxels offer several advantages:
- Simplicity: Easier to understand and implement compared to polygon-based graphics.
- Flexibility: Allows for easy terrain modification.
- Performance: Can be optimized efficiently for specific types of games.
Building Blocks of a Voxel Engine
Let's break down the core components of a voxel engine:
- Voxel Representation
- World Generation
- Rendering
- Interaction
Voxel Representation
Voxels can be represented using a 3D array. Each element in this array corresponds to a voxel in the world.
# Example: 3D array representing voxels world = [[[0 for z in range(10)] for y in range(10)] for x in range(10)] # 0 represents an empty voxel (air)
World Generation
The world generation logic creates the initial distribution of voxels. We can use procedural generation techniques like Perlin noise to generate realistic landscapes.
import noise def generate_world(size): scale = 100.0 world = [[[0 for z in range(size)] for y in range(size)] for x in range(size)] for x in range(size): for y in range(size): for z in range(size): height = int(noise.pnoise3(x/scale, y/scale, z/scale) * size/2 + size/2) if y < height: world[x][y][z] = 1 else: world[x][y][z] = 0 return world size = 50 world = generate_world(size)
Rendering
For rendering, we can use a simple library like Pygame or a more advanced one like OpenGL. Here’s a basic example using Pygame to render 2D projections of our 3D world:
import pygame # Initialize Pygame pygame.init() screen = pygame.display.set_mode((600, 600)) # Colors BLACK = (0, 0, 0) WHITE = (255, 255, 255) def draw_world(world): screen.fill(BLACK) size = len(world) for x in range(size): for y in range(size): for z in range(size): if world[x][y][z] == 1: pygame.draw.rect(screen, WHITE, (x * 10, y * 10, 10, 10)) pygame.display.flip() running = True while running: for event in pygame.event.get(): if event.type == pygame.QUIT: running = False draw_world(world) pygame.quit()
Interaction
Interaction involves detecting and responding to user inputs. For example, placing or removing voxels based on mouse clicks.
def handle_input(world): for event in pygame.event.get(): if event.type == pygame.QUIT: pygame.quit() exit() elif event.type == pygame.MOUSEBUTTONDOWN: x, y = pygame.mouse.get_pos() voxel_x, voxel_y = x // 10, y // 10 world[voxel_x][voxel_y][0] = 1 if world[voxel_x][voxel_y][0] == 0 else 0 running = True while running: handle_input(world) draw_world(world)
Advanced Topics
Here are some advanced topics you might want to explore:
- Optimizing Rendering: Use techniques like frustum culling and level of detail (LOD) to improve performance.
- Physics and Collision Detection: Implement basic physics to allow for more realistic interactions.
- Multiplayer: Sync voxel data between multiple clients for a shared experience.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Why Program? (psst, it's free!).
FAQ
What is a voxel?
A voxel is a volumetric pixel, essentially a tiny cube that represents a value in 3D space. Voxels are the building blocks for creating 3D environments in voxel engines.
Why are voxel engines simpler to understand?
Voxel engines are simpler because they use cubes (voxels) instead of complex polygons. This makes the math and code easier to manage, allowing for straightforward terrain manipulation and rendering.
How can I optimize a voxel engine?
Optimization can be achieved through techniques like frustum culling, level of detail (LOD), and efficient memory management. These methods help reduce the computational load and improve performance.
What libraries can I use for rendering voxels in Python?
For simpler projects, Pygame is a good choice. For more advanced rendering, you can use libraries like PyOpenGL, which provide more control and better performance.
Can voxel engines be used for games other than Minecraft-like worlds?
Absolutely! Voxel engines can be used for various types of games, including simulations, scientific visualizations, and even procedural content generation in non-blocky games.