3D Graphics with Py5
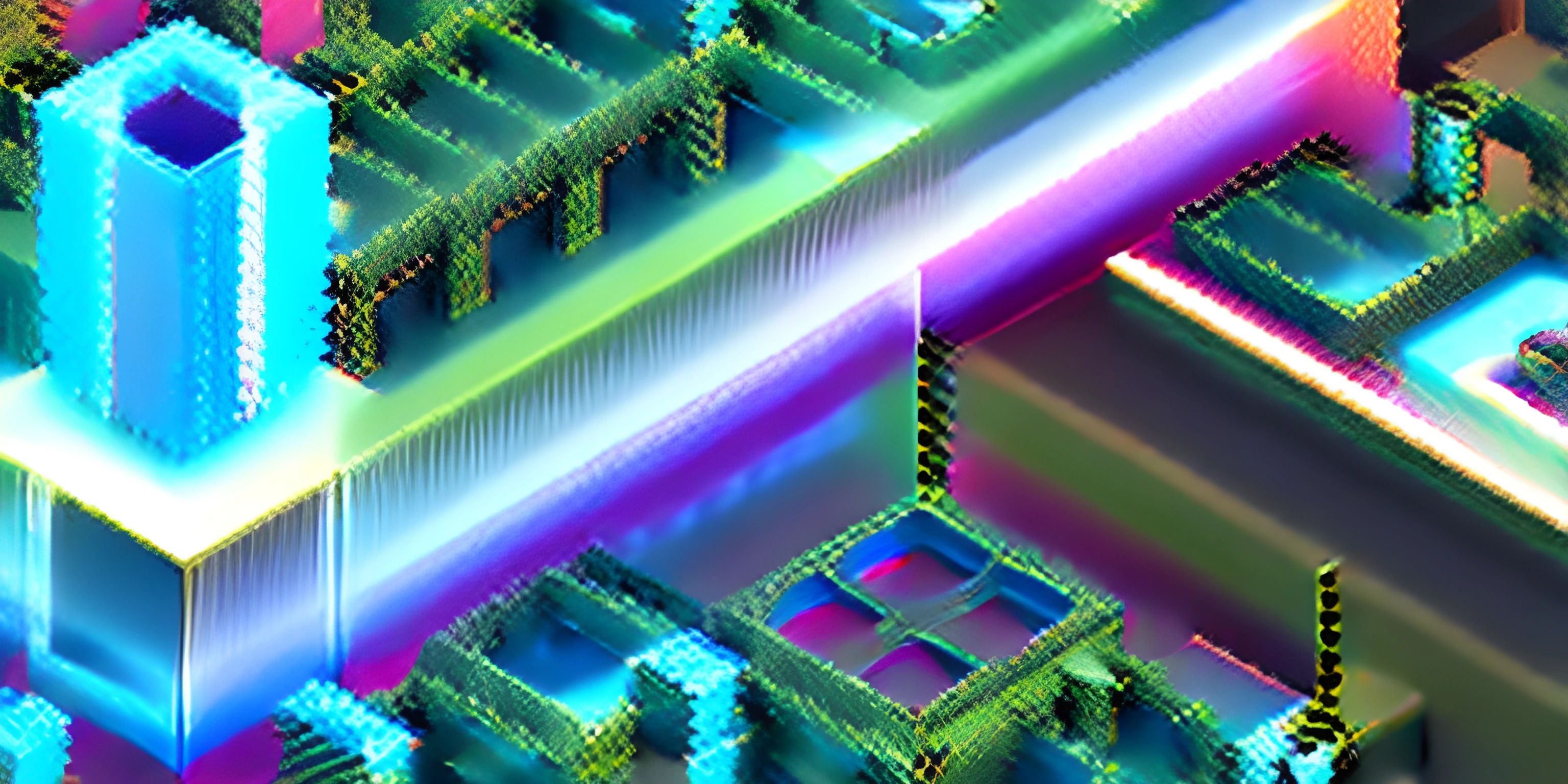
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Imagine taking a flat, boring piece of paper and transforming it into a vibrant 3D world where shapes jump out at you, rotate, and even dance to your commands. This isn't magic—it's the power of 3D graphics! Today, we're diving into the fascinating realm of 3D graphics using the Py5 library, a friendly interface to the Processing graphics library for Python enthusiasts.
Let's get started by setting the stage for our 3D masterpiece.
Setting Up Your Environment
Before we create mind-blowing 3D visuals, we need to set up our environment. To do this, you'll need to install Py5. Open your terminal and run:
pip install py5
Once you have Py5 installed, you're ready to start coding!
The Basics of a Py5 Sketch
In Py5, every sketch starts with two essential functions: setup()
and draw()
. These functions define the initial setup and the continuous drawing loop, respectively.
Let's create a basic Py5 sketch to get the ball rolling:
import py5 def setup(): py5.size(800, 600, py5.P3D) # Set up a window with 3D rendering py5.background(200) # Set a light gray background def draw(): py5.background(200) # Refresh the background each frame py5.run_sketch()
In this example, we're setting up a window with an 800x600 resolution and enabling 3D rendering with py5.P3D
. The draw()
function continuously refreshes the background to ensure smooth animations.
Drawing 3D Shapes
Now that we have a basic setup, let's move on to drawing some 3D shapes. Py5 allows you to create various 3D primitives such as boxes, spheres, and cylinders.
Drawing a Box
Here's how to draw a rotating 3D box:
import py5 def setup(): py5.size(800, 600, py5.P3D) py5.background(200) def draw(): py5.background(200) py5.translate(py5.width / 2, py5.height / 2, -200) # Move to the center py5.rotate_x(py5.frame_count * 0.01) # Rotate around the X-axis py5.rotate_y(py5.frame_count * 0.01) # Rotate around the Y-axis py5.box(150) # Draw a box with a width, height, and depth of 150 py5.run_sketch()
In this code, py5.translate()
moves the origin to the center of the window, and py5.rotate_x()
and py5.rotate_y()
apply rotations around the X and Y axes. As a result, the box appears to rotate continuously.
Drawing a Sphere
Next, let's draw a sphere:
import py5 def setup(): py5.size(800, 600, py5.P3D) py5.background(200) def draw(): py5.background(200) py5.translate(py5.width / 2, py5.height / 2, -200) py5.rotate_x(py5.frame_count * 0.01) py5.rotate_y(py5.frame_count * 0.01) py5.sphere(100) # Draw a sphere with a radius of 100 py5.run_sketch()
Similar to the box, the sphere rotates around the X and Y axes.
Adding Lighting
To make our 3D shapes look more realistic, we need to add some lighting. Py5 provides several functions to create different types of lights, such as ambient_light()
, directional_light()
, and point_light()
.
Here's how to add a simple directional light to our rotating box:
import py5 def setup(): py5.size(800, 600, py5.P3D) py5.background(200) py5.no_stroke() # Remove outlines for a smooth appearance def draw(): py5.background(200) py5.directional_light(255, 255, 255, 1, 1, -1) # White light from the top-right py5.translate(py5.width / 2, py5.height / 2, -200) py5.rotate_x(py5.frame_count * 0.01) py5.rotate_y(py5.frame_count * 0.01) py5.box(150) py5.run_sketch()
The py5.directional_light()
function adds a white light that shines from the top-right direction, enhancing the 3D effect of our box.
Animating 3D Objects
Animating 3D objects in Py5 is straightforward. By manipulating the py5.frame_count
, we can create smooth animations.
Let's create an example where multiple spheres move in a circular pattern:
import py5 import math def setup(): py5.size(800, 600, py5.P3D) py5.background(0) py5.no_stroke() def draw(): py5.background(0) py5.directional_light(255, 255, 255, 1, 1, -1) py5.translate(py5.width / 2, py5.height / 2, -200) for i in range(10): angle = py5.frame_count * 0.02 + i * (2 * math.pi / 10) x = 200 * math.cos(angle) y = 200 * math.sin(angle) py5.push_matrix() py5.translate(x, y, 0) py5.sphere(30) py5.pop_matrix() py5.run_sketch()
In this code, we use trigonometric functions (math.cos
and math.sin
) to calculate the positions of the spheres, creating a circular animation.
Combining Shapes and Animations
For the grand finale, let's combine multiple shapes and animations to create a mesmerizing 3D scene. We'll draw a rotating box surrounded by orbiting spheres:
import py5 import math def setup(): py5.size(800, 600, py5.P3D) py5.background(0) py5.no_stroke() def draw(): py5.background(0) py5.directional_light(255, 255, 255, 1, 1, -1) py5.translate(py5.width / 2, py5.height / 2, -200) # Draw rotating box py5.push_matrix() py5.rotate_x(py5.frame_count * 0.01) py5.rotate_y(py5.frame_count * 0.01) py5.box(150) py5.pop_matrix() # Draw orbiting spheres for i in range(10): angle = py5.frame_count * 0.02 + i * (2 * math.pi / 10) x = 200 * math.cos(angle) y = 200 * math.sin(angle) py5.push_matrix() py5.translate(x, y, 0) py5.sphere(30) py5.pop_matrix() py5.run_sketch()
This code showcases the power of combining different shapes and animations, creating an engaging and dynamic 3D scene.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Color Mandelbrot Set (psst, it's free!).
FAQ
How do I install Py5?
You can install Py5 using pip. Open your terminal and run the command pip install py5
.
Can I draw custom 3D shapes in Py5?
Yes, you can draw custom 3D shapes using the begin_shape()
, vertex()
, and end_shape()
functions. This allows you to create complex 3D models by defining their vertices.
How can I improve the performance of my Py5 sketches?
To improve performance, you can reduce the number of shapes, lower the resolution, or optimize your code by minimizing complex calculations within the draw()
function. Profiling tools can also help identify bottlenecks.
Can I export my 3D animations to a video file?
Yes, you can export your 3D animations by saving each frame as an image using save_frame()
. Then, you can use external tools like FFmpeg to combine these frames into a video file.
What other libraries can I use for 3D graphics in Python?
In addition to Py5, you can use libraries like Pygame, OpenGL, and PyOpenGL for 3D graphics in Python. Each library has its strengths and use cases.