Understanding APIs and How They Are Used in Web Development
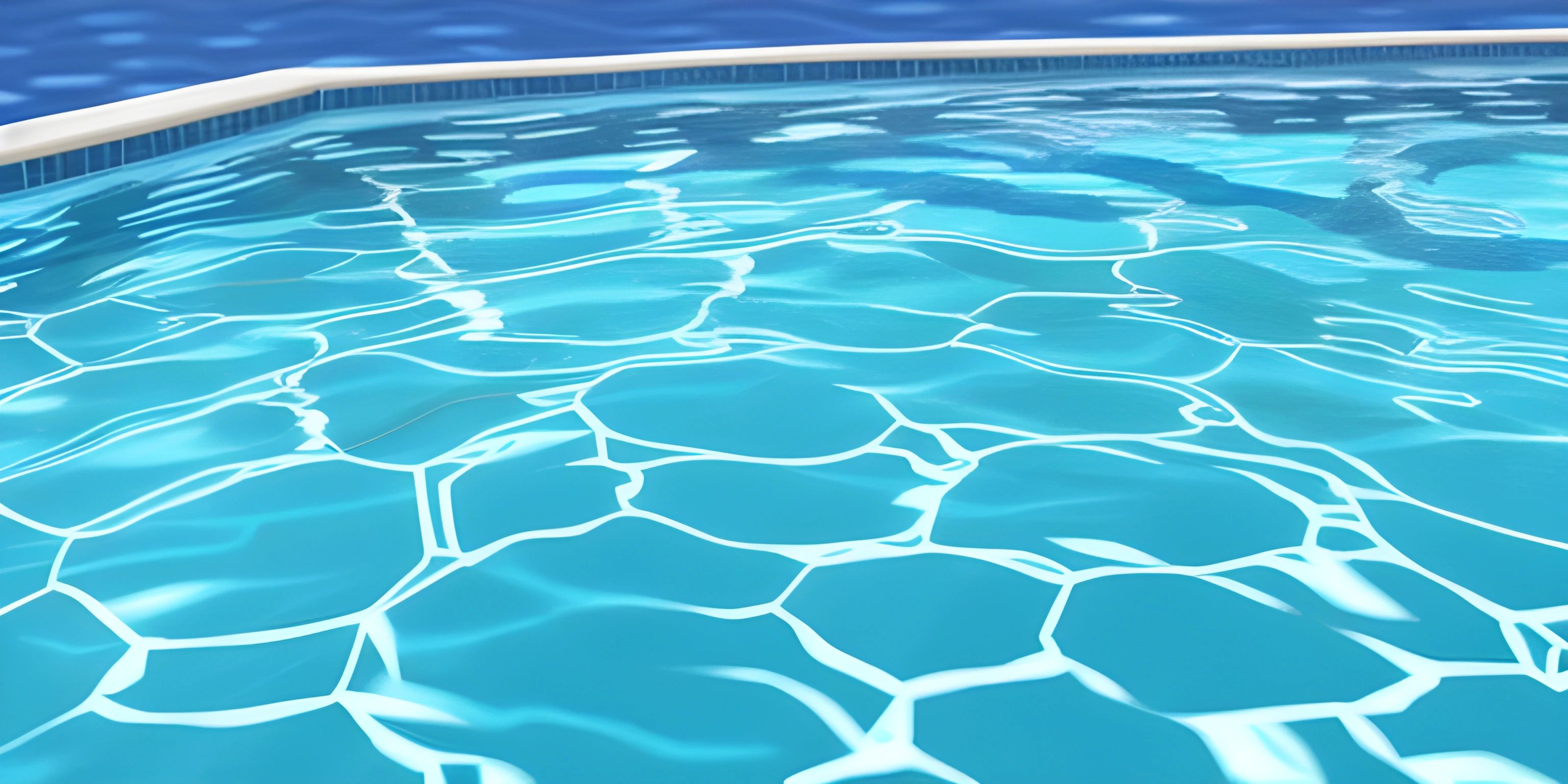
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Navigating the vast sea of web development can feel like steering a ship through uncharted waters. But fear not, brave coder! Today, we’ll uncover one of the essential treasures of the digital realm: APIs.
What is an API?
An API (Application Programming Interface) is like a magical middleman that allows different software applications to communicate with each other. Imagine you're at a restaurant. You, the hungry customer, are the application. The kitchen, which prepares your food, is another application. The waiter who takes your order and brings you your delicious meal? That's the API.
In essence, an API is a set of rules and protocols that enables different software entities to talk to each other. These rules define how requests and responses should be formatted, much like how a waiter must know the menu and properly relay orders to the kitchen.
The Anatomy of an API Request
To understand APIs better, let's break down the anatomy of an API request. Here’s a typical flow:
- Client: The application or user making the request.
- Endpoint: The URL path where the API can be accessed.
- Method: The type of request being made (GET, POST, PUT, DELETE).
- Headers: Additional information sent with the request, like authentication tokens.
- Body: The data sent with the request, often in JSON format.
Here’s an example of a simple API request using JavaScript:
// Define the API endpoint const url = "https://api.example.com/data"; // Use the Fetch API to make a GET request fetch(url, { method: "GET", // The method (GET request) headers: { "Content-Type": "application/json", // Header specifying that we expect JSON data } }) .then(response => response.json()) // Parse the JSON response .then(data => { console.log("Data received:", data); // Log the data received }) .catch(error => { console.error("Error fetching data:", error); // Handle any errors });
In this example, we’re making a GET request to the specified API endpoint. We include headers to indicate that we expect JSON data, and handle the response accordingly.
Types of APIs
APIs come in various flavors, but two of the most popular are REST and SOAP.
REST (Representational State Transfer)
RESTful APIs are like the Swiss Army knives of the web. They use standard HTTP methods (GET, POST, PUT, DELETE) and are designed to be stateless, meaning each request from a client contains all the information needed to process it. REST APIs often use JSON (JavaScript Object Notation) for data exchange, which is lightweight and easy to read.
Here’s an example of a RESTful API interaction:
// Define the data to be sent to the API const postData = { name: "Cratecode", type: "Educational" }; // Make a POST request to the API endpoint fetch("https://api.example.com/create", { method: "POST", // The method (POST request) headers: { "Content-Type": "application/json", // Header specifying that we are sending JSON data }, body: JSON.stringify(postData) // Convert our data to JSON and include it in the request body }) .then(response => response.json()) // Parse the JSON response .then(data => { console.log("Resource created:", data); // Log the data received }) .catch(error => { console.error("Error creating resource:", error); // Handle any errors });
SOAP (Simple Object Access Protocol)
SOAP is like the older, more formal cousin of REST. It relies on XML for message format and has more standardized rules for web services. SOAP is known for its robustness and strict standards, making it a preferred choice for enterprise-level applications that require high security and transactional reliability.
Understanding the difference between REST and SOAP is crucial for choosing the right type of API for your project.
Real World Applications of APIs
APIs are everywhere! They power many of the services we use daily. Here are a couple of real-world examples:
Social Media Integration
Ever noticed how you can log into various websites using your Google or Facebook account? That’s thanks to APIs. These platforms expose their authentication services via APIs, allowing third-party apps to leverage them for user login.
Payment Gateways
Services like PayPal and Stripe provide APIs that websites can use to process payments. This allows developers to integrate secure payment options without handling sensitive financial information directly.
Best Practices for Using APIs
When working with APIs, adhering to best practices ensures smooth and efficient communication between your application and the API. Here are some tips:
- Authentication & Authorization: Always use proper authentication methods (like OAuth) to secure your API requests.
- Rate Limiting: Be mindful of the API’s rate limits to avoid getting blocked.
- Error Handling: Implement robust error handling to gracefully manage API errors and provide informative feedback to users.
- Documentation: Refer to the API documentation for detailed information on endpoints, methods, and data formats.
Conclusion
APIs are the unsung heroes of modern web development, enabling seamless interaction between different software systems. Whether you're fetching data, integrating third-party services, or building complex applications, understanding how to work with APIs is a crucial skill for any developer.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Why Program? (psst, it's free!).
FAQ
What is an API?
An API (Application Programming Interface) is a set of rules and protocols that allows different software applications to communicate with each other. It acts as a middleman, much like a waiter in a restaurant, facilitating the exchange of data and functionality between systems.
What are the common types of APIs used in web development?
The two most common types of APIs in web development are REST (Representational State Transfer) and SOAP (Simple Object Access Protocol). REST uses standard HTTP methods and is often used with JSON data, while SOAP relies on XML and follows stricter standards for message format and communication.
Why are APIs important in web development?
APIs are crucial in web development because they enable different software systems to interact and share data seamlessly. They allow developers to integrate third-party services, fetch data from external sources, and build more dynamic and functional applications.
How do I make an API request using JavaScript?
You can make an API request in JavaScript using the Fetch API. For example, a simple GET request looks like this:
fetch("https://api.example.com/data", { method: "GET", headers: { "Content-Type": "application/json", } }) .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error(error));
What are some best practices for using APIs?
Some best practices for using APIs include using proper authentication methods, being mindful of rate limits, implementing robust error handling, and referring to the API documentation for detailed information on endpoints and data formats. Following these practices ensures secure and efficient communication with the API.