Understanding RESTful APIs
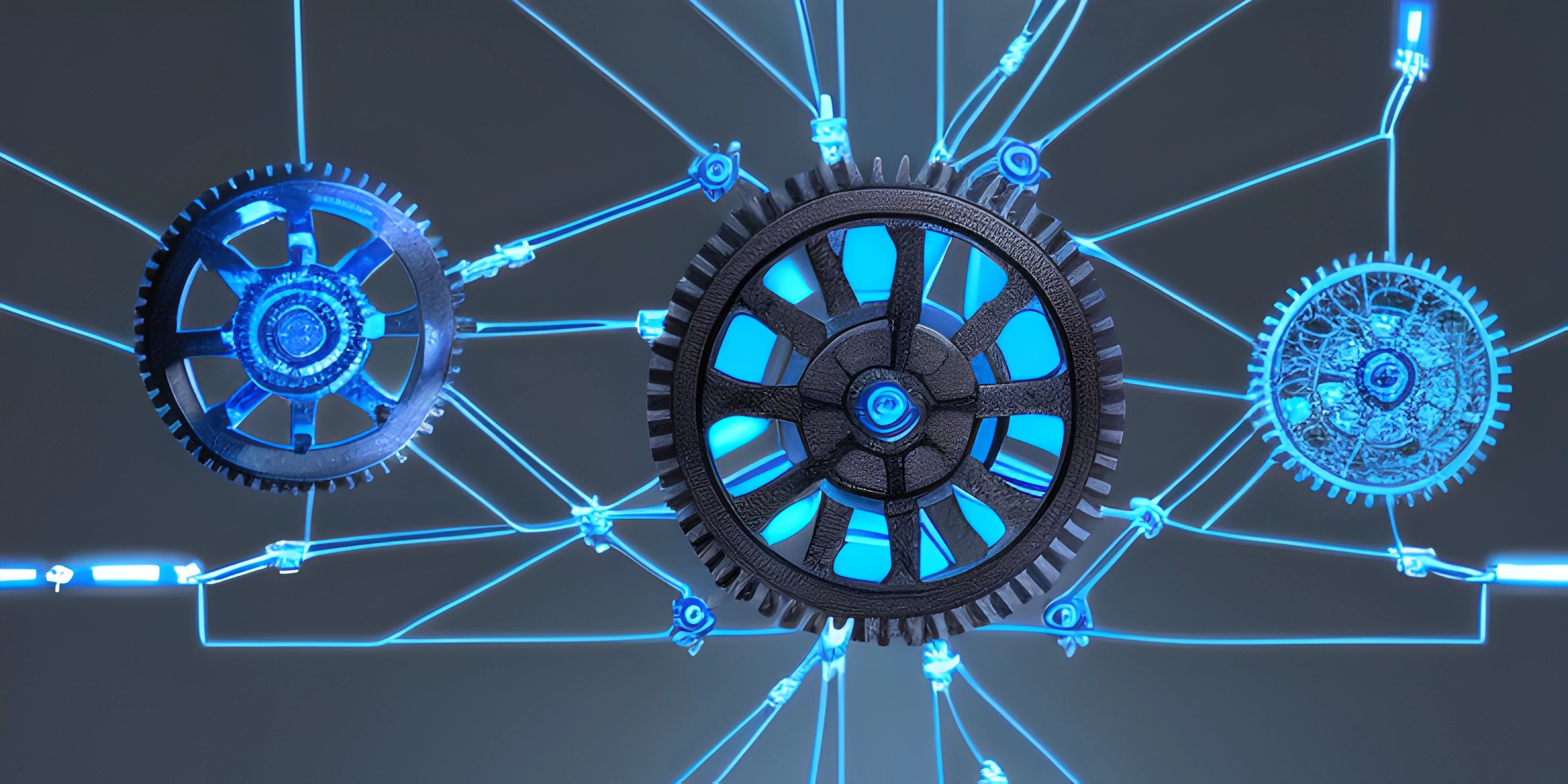
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
RESTful APIs play a critical role in the modern world of web development. They allow different software applications to communicate with each other, making it possible to build complex and feature-rich web applications. But what exactly are RESTful APIs, and how do they work? Let's find out!
What is a RESTful API?
REST (Representational State Transfer) is an architectural style for designing networked applications. A RESTful API is an application programming interface (API) that adheres to REST principles, enabling different software systems to exchange data over HTTP.
RESTful APIs provide a standardized way for various applications to request and manipulate resources, such as data objects, through a set of predefined HTTP methods like GET, POST, PUT, DELETE, and others.
Key Concepts
Before diving into RESTful API implementation, let's discuss some essential concepts that help to understand how RESTful APIs work.
Resources
In RESTful APIs, resources are the core entities that the API deals with. A resource can represent anything, such as a user, a product, or an order. Each resource is identified by a unique identifier, typically a Uniform Resource Identifier (URI).
HTTP Methods
HTTP methods define the actions that can be performed on resources. The most commonly used methods in RESTful APIs are:
- GET: Retrieve a resource or a collection of resources.
- POST: Create a new resource.
- PUT: Update an existing resource.
- DELETE: Delete a resource.
Endpoint
An endpoint is a specific URL where the API can be accessed to perform a particular operation. In RESTful APIs, endpoints are usually designed as resource-based URLs, such as /users
or /orders/123
.
Implementing a RESTful API
Now that we understand the basic concepts, let's explore the steps to implement a simple RESTful API.
1. Designing the API
Before writing any code, it's essential to plan and design the API by identifying the resources, defining the HTTP methods, and structuring the endpoints. For example, if we are building an API for managing users, we might create the following endpoints:
GET /users
: Retrieve a list of users.GET /users/{id}
: Retrieve a specific user by ID.POST /users
: Create a new user.PUT /users/{id}
: Update a user by ID.DELETE /users/{id}
: Delete a user by ID.
2. Choosing a Framework or Library
Several web development frameworks and libraries make it easier to implement RESTful APIs. Some popular choices include Express.js for Node.js, Flask for Python, and Ruby on Rails for Ruby. Select a framework or library that suits your technology stack and requirements.
3. Implementing the API
Using the chosen framework or library, implement the API by writing code that handles incoming HTTP requests, performs the necessary operations, and returns the appropriate HTTP response. This may involve interacting with databases, performing validation, and handling errors.
4. Testing and Documentation
During and after the implementation phase, it's crucial to test the API to ensure that it works as expected. You can use tools like Postman or Curl to send HTTP requests and validate the API's responses.
Additionally, create comprehensive documentation that describes the API's resources, endpoints, request parameters, and response formats. This documentation will help other developers understand and use your API effectively.
Best Practices
When building RESTful APIs, keep the following best practices in mind to create scalable and maintainable APIs:
- Use standard HTTP methods and status codes.
- Keep endpoint URLs resource-based and consistent.
- Use proper authentication and authorization mechanisms.
- Implement pagination and filtering for large data sets.
- Validate input data and provide informative error messages.
- Version your API to handle changes without breaking existing clients.
With a solid grasp of RESTful API concepts and best practices, you're now ready to design, implement, and maintain effective APIs that power modern web applications. Happy coding!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Async Rust (psst, it's free!).
FAQ
What is a RESTful API?
A RESTful API (Representational State Transfer) is a web service that follows a set of architectural constraints, allowing clients to interact with and manipulate web resources. These APIs enable communication between different software applications over the internet, using standard HTTP methods like GET, POST, PUT, PATCH, and DELETE.
How do RESTful APIs work?
RESTful APIs work by exposing web resources (data objects) through unique URLs. Clients send HTTP requests to these URLs, specifying the desired action (GET, POST, PUT, PATCH, or DELETE) and any necessary data. The server processes the request, performs the action on the resource, and sends an HTTP response back to the client, often with a status code and relevant data.
What are some best practices for implementing RESTful APIs?
Here are a few best practices for implementing RESTful APIs:
- Use consistent and meaningful naming conventions for your URLs and resources.
- Leverage standard HTTP methods (GET, POST, PUT, PATCH, DELETE) to perform actions on resources.
- Make use of proper status codes to indicate the result of an API request.
- Provide clear and concise documentation for developers who will interact with your API.
- Implement proper authentication and authorization to secure your API.
Can you give an example of a RESTful API request?
Sure! Let's say we have an API that manages a collection of books. To fetch the details of a book with the ID 123
, a client would send a GET request to the URL https://api.example.com/books/123
. The server would then process the request, retrieve the book details, and send them back as an HTTP response in a format like JSON or XML.
What are some common alternatives to RESTful APIs?
Some common alternatives to RESTful APIs include:
- GraphQL: A query language for APIs that allows clients to request only the data they need, reducing the amount of data transferred over the network.
- SOAP (Simple Object Access Protocol): A protocol that uses XML to exchange structured information between web services, often seen as more complex and less flexible compared to REST.
- gRPC: A high-performance, open-source RPC (Remote Procedure Call) framework that uses Protocol Buffers for efficient serialization, suitable for low-latency and high-throughput communication.