Asyncio in Python
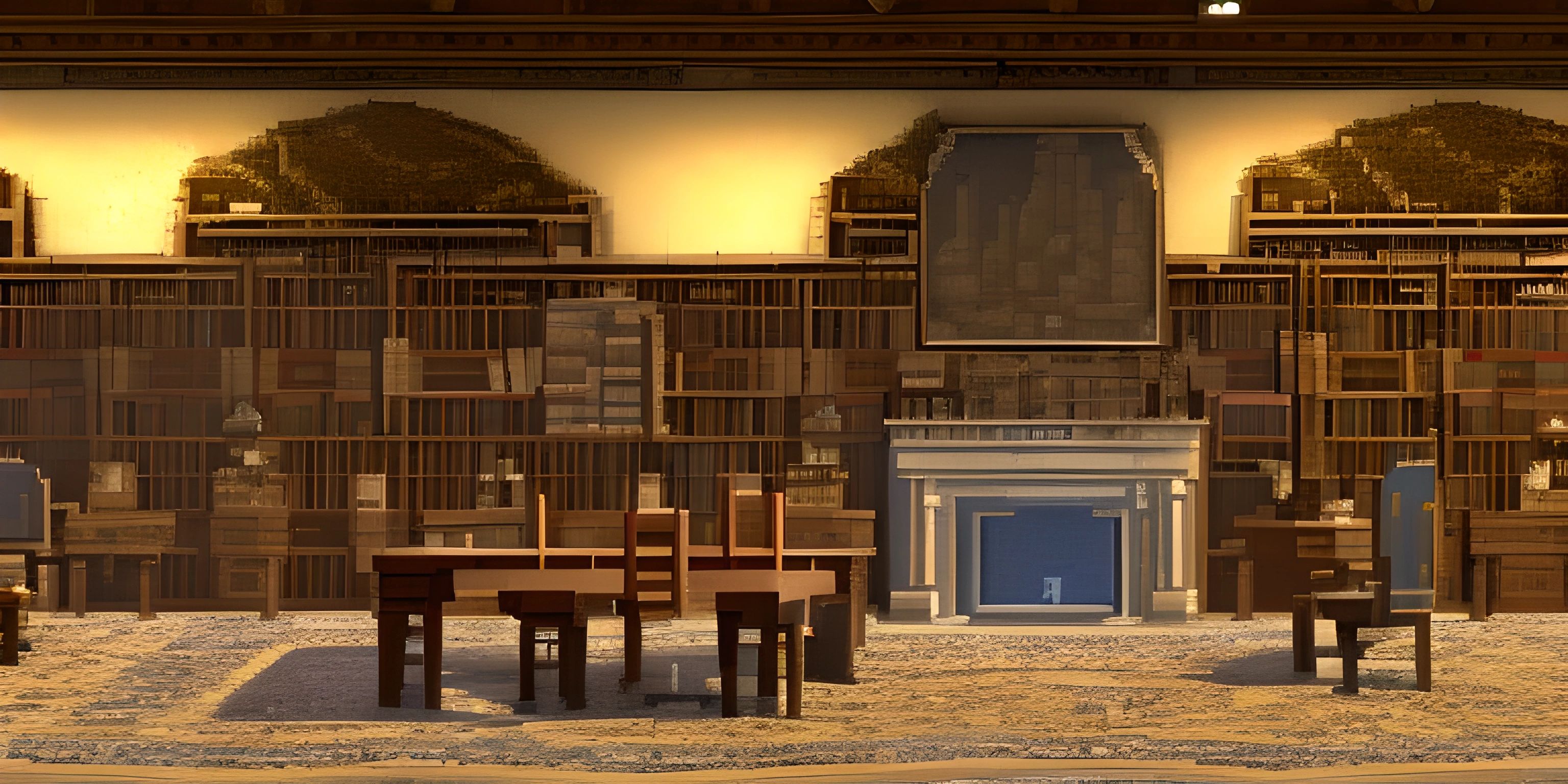
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Asyncio is a powerful library built into Python to help you write asynchronous, non-blocking code. It's like giving your Python program superpowers to handle multiple tasks at once, eliminating the need to wait for one task to complete before starting another. Sounds cool, right? Let's dive in!
What is asynchronous programming?
Asynchronous programming, or async for short, is a programming paradigm that allows multiple tasks to run concurrently. This is particularly useful when tasks are I/O-bound, meaning they spend a lot of time waiting for input and output operations to complete, such as reading from a file or making an HTTP request.
In contrast, synchronous programming runs tasks one after another, waiting for each to complete before starting the next. This can lead to inefficiencies when tasks are I/O-bound, as the program is sitting idle while waiting for the I/O operation to complete.
Asyncio brings the power of asynchronous programming to Python, allowing you to write concurrent and non-blocking code that can handle a large number of I/O-bound tasks efficiently.
The basics of asyncio
The main building blocks of asyncio are coroutines. Coroutines are special Python functions that can be paused and resumed, allowing other tasks to run in the meantime. They're defined using the async def
keyword, like this:
async def my_coroutine(): print("Hello from the coroutine!")
To run a coroutine, you need an event loop. The event loop is the heart of asyncio, managing the execution of coroutines and handling I/O operations. Here's how you can create and run a simple event loop:
import asyncio async def my_coroutine(): print("Hello from the coroutine!") # Create an event loop loop = asyncio.get_event_loop() # Run the coroutine using the event loop loop.run_until_complete(my_coroutine()) # Close the event loop loop.close()
Awaiting other coroutines
One of the most powerful features of coroutines is their ability to call other coroutines using the await
keyword. This allows one coroutine to pause and wait for another coroutine to finish, without blocking the entire program. Here's an example:
import asyncio async def my_coroutine(): print("Starting the coroutine...") await asyncio.sleep(1) print("Coroutine finished!") loop = asyncio.get_event_loop() loop.run_until_complete(my_coroutine()) loop.close()
In this example, my_coroutine()
calls asyncio.sleep(1)
, which is a coroutine that sleeps for one second. The await
keyword tells the event loop to pause my_coroutine()
and let other tasks run while waiting for the sleep to finish. After one second, the event loop resumes my_coroutine()
and prints "Coroutine finished!".
Running multiple coroutines concurrently
The real power of asyncio comes when you want to run multiple coroutines concurrently. For example, let's say you want to fetch data from several websites at once. With asyncio, you can do this efficiently by running multiple coroutines concurrently, like this:
import asyncio import aiohttp async def fetch(url): async with aiohttp.ClientSession() as session: async with session.get(url) as response: return await response.text() async def main(): urls = ["https://www.example.com", "https://www.example.org", "https://www.example.net"] tasks = [fetch(url) for url in urls] results = await asyncio.gather(*tasks) for url, result in zip(urls, results): print(f"{url}: {len(result)} bytes") loop = asyncio.get_event_loop() loop.run_until_complete(main()) loop.close()
In this example, we use the aiohttp
library to fetch data from multiple websites concurrently. We create a list of tasks, each calling the fetch()
coroutine with a different URL, and then use asyncio.gather()
to run all the tasks concurrently and collect their results.
By running the coroutines concurrently, we can fetch data from multiple websites much faster than if we fetched them one by one using synchronous programming.
Conclusion
Asyncio is a powerful library that brings the power of asynchronous programming to Python, allowing you to write concurrent, non-blocking code that can handle a large number of I/O-bound tasks efficiently. By leveraging coroutines, event loops, and the await
keyword, you can harness the full power of async programming in your Python projects. Happy coding!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Syntax (psst, it's free!).
FAQ
What is asyncio and why should I use it in Python?
Asyncio is a powerful library in Python that allows you to write asynchronous code. Asynchronous programming enables you to execute multiple tasks concurrently without blocking the execution of other tasks. This can greatly improve the performance and efficiency of your code, especially when dealing with I/O-bound tasks, such as fetching data from an API or reading from a file.
How do I install asyncio and set up my Python environment?
Asyncio is included in the Python Standard Library starting from Python 3.4, so there's no need to install it separately. However, to use asyncio effectively, it's recommended that you use Python 3.7 or later, as it introduces important features and improvements like the async
and await
keywords.
How do I create a simple asynchronous function with asyncio?
To create an asynchronous function, you need to use the async def
syntax, followed by the function name. Inside the function, you can use the await
keyword to call other asynchronous functions or coroutines. Here's a simple example:
import asyncio async def hello_world(): print("Hello") await asyncio.sleep(1) print("World!") asyncio.run(hello_world())
What is an event loop and how does it work in asyncio?
An event loop is the core component of the asyncio library. It's responsible for scheduling and executing tasks concurrently, managing I/O operations, and handling timeouts and delays. When you run your asyncio-based application, you generally create an event loop instance, which schedules the tasks you define and runs them asynchronously. The asyncio.run()
function, introduced in Python 3.7, creates an event loop, runs the given coroutine, and closes the loop when the coroutine is complete.
How do I run multiple asynchronous tasks concurrently using asyncio?
To run multiple asynchronous tasks concurrently, you can use the asyncio.gather()
function. This function takes a list of coroutines and returns a single coroutine, which completes when all the input coroutines are done. Here's an example:
import asyncio async def task1(): print("Task 1 started") await asyncio.sleep(2) print("Task 1 completed") async def task2(): print("Task 2 started") await asyncio.sleep(1) print("Task 2 completed") async def main(): await asyncio.gather(task1(), task2()) asyncio.run(main())
This code runs both task1()
and task2()
concurrently, making the overall execution faster.