Getting Started with Pandas
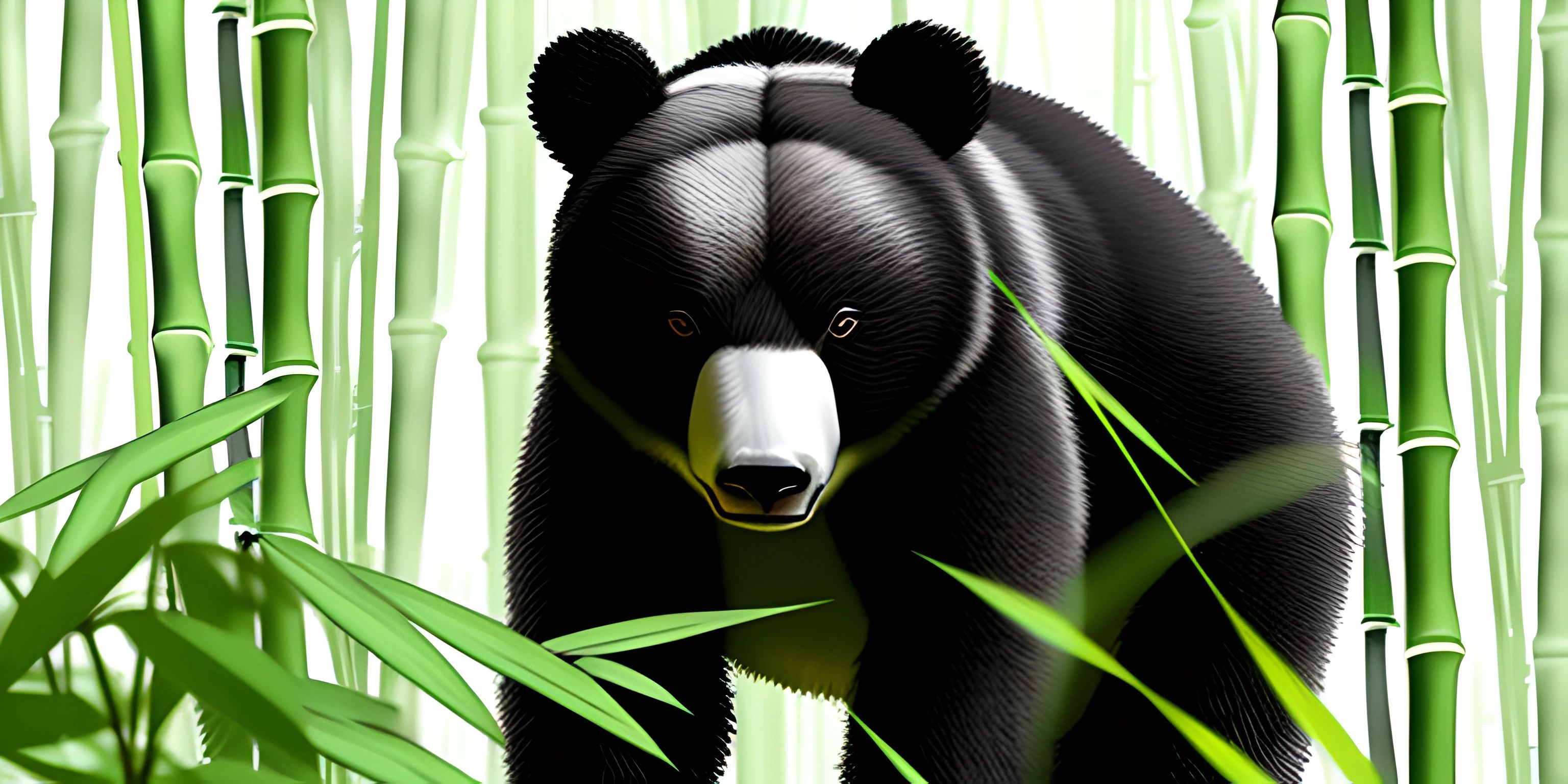
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Pandas is a popular and powerful library in Python that makes data manipulation a breeze. In a world where data is king, this library is the knight in shining armor, ready to tackle any data-related quest.
What is Pandas?
Pandas is an open-source data manipulation library built on top of the Python programming language. It provides data structures and functions needed to manipulate and analyze structured data, like spreadsheets and SQL tables. Pandas is known for its two core data structures: the Series and the DataFrame.
Installation
Before diving into Pandas, you'll need to install it. You can install Pandas using pip, the package installer for Python.
pip install pandas
Once Pandas is installed, you can import it into your Python script like so:
import pandas as pd
The "pd" alias is a convention and makes it easier to reference Pandas functions and structures as you work.
Series and DataFrames
A Series is a one-dimensional labeled array capable of holding any data type, while a DataFrame is a two-dimensional data structure, like a table in a spreadsheet or an SQL database, with labeled axes (rows and columns). Both Series and DataFrames can handle a variety of data, including numbers, strings, and even other Python objects.
Creating Series and DataFrames
You can create a Series from a list or dictionary:
import pandas as pd my_list = [1, 2, 3, 4] my_series = pd.Series(my_list)
Similarly, you can create a DataFrame from a list, dictionary, or other data structures:
import pandas as pd my_dict = {"Name": ["Alice", "Bob", "Charlie"], "Age": [25, 30, 35]} my_dataframe = pd.DataFrame(my_dict)
Data Manipulation
Pandas' real strength is in data manipulation. With a few lines of code, you can easily sort, filter, and transform your data.
Sorting Data
You can sort a DataFrame by the values in one or more columns using the sort_values()
method:
sorted_df = my_dataframe.sort_values("Age")
Filtering Data
You can filter the data using boolean indexing:
over_25 = my_dataframe[my_dataframe["Age"] > 25]
Transforming Data
You can also apply functions to transform data, like adding a new column that's a function of existing columns:
my_dataframe["Age in Months"] = my_dataframe["Age"] * 12
Wrapping Up
Pandas is a powerful and flexible library that makes data manipulation in Python simple and efficient. By mastering Series and DataFrames, you'll be well on your way to becoming a data-wrangling wizard. So saddle up, grab your lance, and get ready to joust with your data!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Advanced Data Types (psst, it's free!).
FAQ
What is the Pandas library, and why is it useful for data manipulation in Python?
Pandas is an open-source library in Python that provides data manipulation and data analysis tools. It is built on top of the NumPy library and is designed to make working with structured data (such as spreadsheets and SQL tables) easy and efficient. With Pandas, you can clean, transform, and analyze data in a way that is both powerful and user-friendly.
How do I install Pandas in my Python environment?
To install Pandas, you can use the pip package manager by running the following command:
pip install pandas
Or, if you're using Anaconda, you can install it with:
conda install pandas
How can I create a Pandas DataFrame from a CSV file?
To create a DataFrame from a CSV file, you'll first need to import the Pandas library, and then use the read_csv()
function. Here's an example:
import pandas as pd filename = "your_csv_file.csv" df = pd.read_csv(filename)
What are some basic operations I can perform on a Pandas DataFrame?
With a Pandas DataFrame, you can perform various operations such as:
- Viewing the first or last few rows with
head()
andtail()
methods - Getting summary statistics with
describe()
- Selecting specific columns or rows using indexing and slicing
- Filtering data based on conditions
- Sorting data by column values
- Merging or joining multiple DataFrames
How can I save my Pandas DataFrame to a new CSV file?
To save your DataFrame to a new CSV file, you can use the to_csv()
method. Here's an example:
output_filename = "output_csv_file.csv" df.to_csv(output_filename, index=False)
The index=False
parameter prevents the DataFrame index from being included in the output file.