Introduction to NumPy
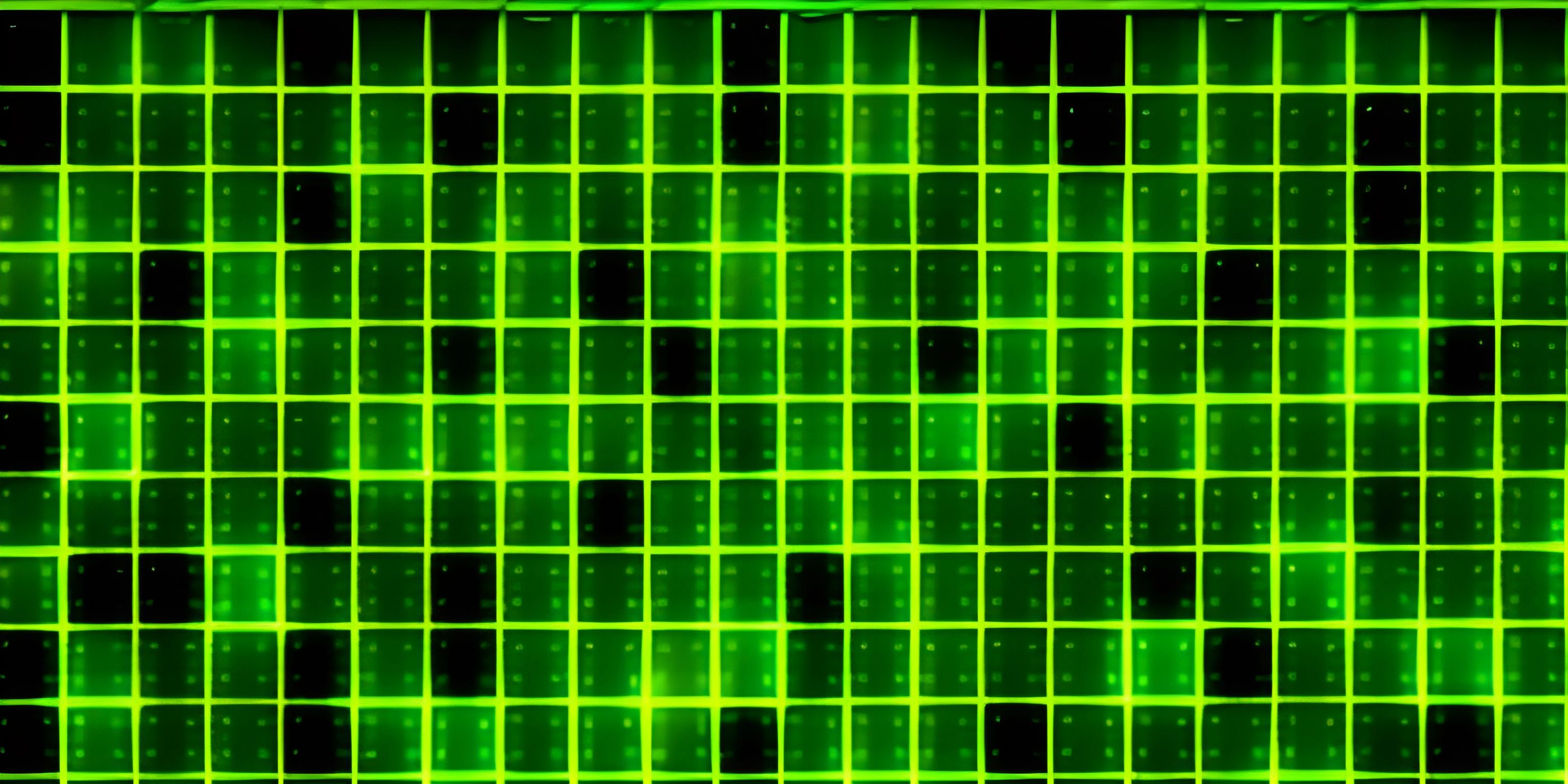
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Welcome to the world of NumPy, a powerful and versatile library for numerical computing in Python. NumPy stands for "Numerical Python" and is the go-to library for handling arrays, performing mathematical operations, and dealing with complex data types.
NumPy Arrays
The core of NumPy is its n-dimensional array, also known as the ndarray
. NumPy arrays are a lot like lists in Python, but with some significant differences. For starters, they are more efficient for numerical operations, allowing for better performance and memory usage.
Here's how you can create a simple NumPy array:
import numpy as np arr = np.array([1, 2, 3, 4, 5]) print(arr)
This code imports the NumPy library and creates a one-dimensional array with five elements. You can also create multi-dimensional arrays, like this 2x3 matrix:
matrix = np.array([[1, 2, 3], [4, 5, 6]]) print(matrix)
Array Operations
NumPy arrays make it incredibly simple to perform mathematical operations on your data. You can perform element-wise addition, subtraction, multiplication, and more without having to write complex loops.
Here's an example of adding two arrays together:
import numpy as np arr1 = np.array([1, 2, 3]) arr2 = np.array([4, 5, 6]) result = arr1 + arr2 print(result)
This code creates two arrays and adds them together. The output will be [5, 7, 9]
.
Array Functions
NumPy also comes with a plethora of built-in functions to help you analyze and manipulate your data. Some of the most common functions include:
np.sum()
: Compute the sum of all elements in an array.np.mean()
: Calculate the mean (average) value of an array.np.std()
: Compute the standard deviation of an array.np.reshape()
: Change the shape of an array without altering its data.np.dot()
: Calculate the dot product of two arrays.
Here's a quick example that calculates the sum and mean of an array:
import numpy as np arr = np.array([1, 2, 3, 4, 5]) sum_value = np.sum(arr) mean_value = np.mean(arr) print("Sum:", sum_value) print("Mean:", mean_value)
This code will output:
Sum: 15 Mean: 3.0
Broadcasting
One of the most powerful features of NumPy is broadcasting, which allows you to perform operations on arrays with different shapes and sizes. NumPy automatically handles the resizing and alignment of the arrays to make the operation possible.
For example, let's say you want to add a constant value to all elements in an array:
import numpy as np arr = np.array([1, 2, 3, 4, 5]) constant = 5 result = arr + constant print(result)
Here, NumPy takes care of broadcasting the constant value 5
to match the shape of the arr
array. The output will be [6, 7, 8, 9, 10]
.
In Conclusion
NumPy is an essential tool for anyone working with numerical data in Python. Its arrays, functions, and broadcasting capabilities make it a powerful and flexible library for a wide range of applications, from data analysis to machine learning. So, roll up your sleeves and dive into the world of NumPy for a truly numerical adventure!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Syntax (psst, it's free!).