Creating Animations in Python with py5
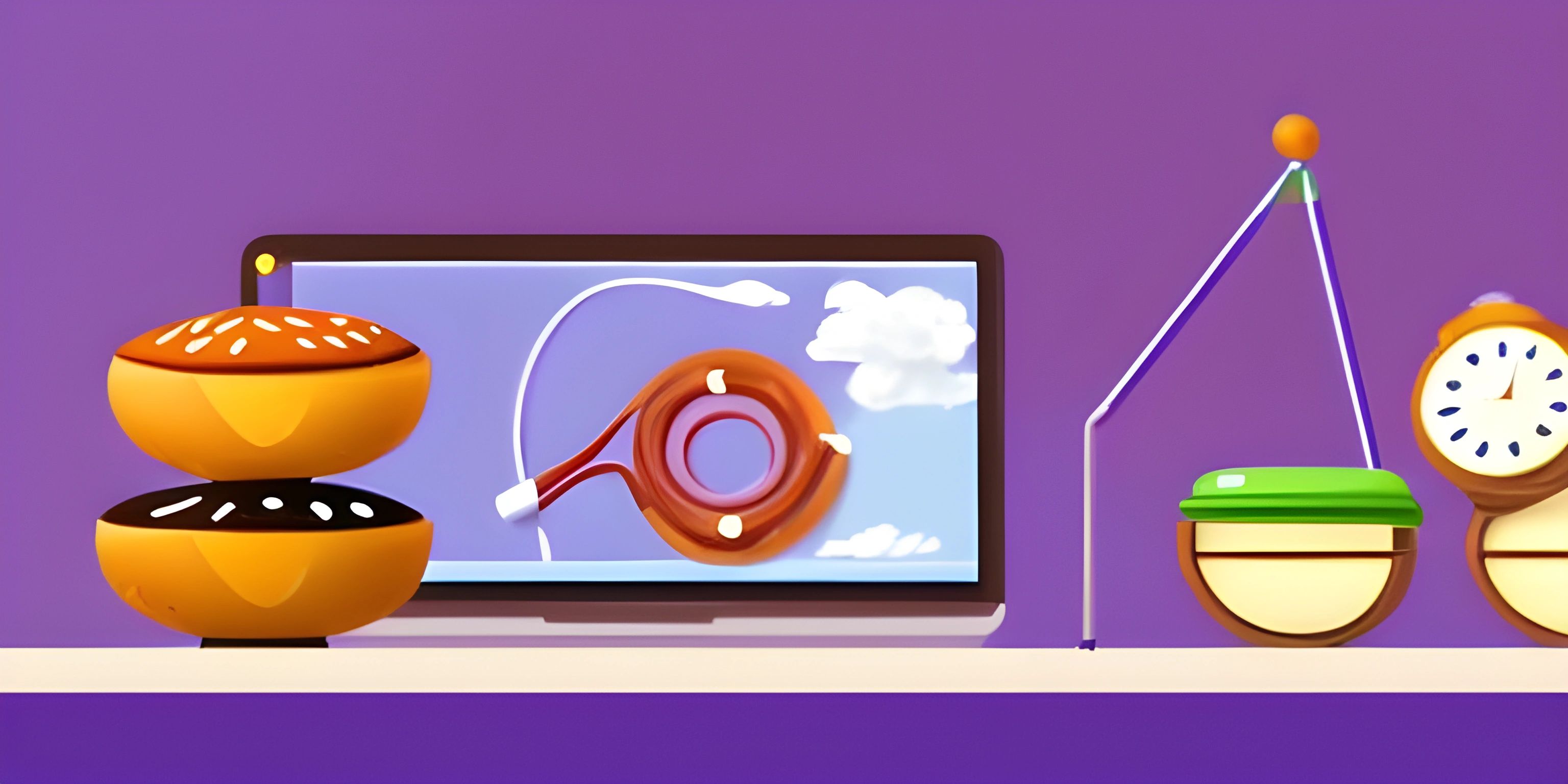
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Who doesn't love a good animation? From Disney classics to the latest Pixar movies, animations captivate us. But did you know you can create your very own animations using Python? Enter the py5 library, your new best friend for creating stunning visualizations and animations. In this guide, we'll explore how to animate objects on the screen, making them move, dance, and come to life. Grab some popcorn and let's dive into the world of py5 animations!
Getting Started with py5
Before we begin, you need to install py5. You can do this easily using pip:
pip install py5
Once installed, let's set up a basic py5 sketch. Think of a sketch as a canvas where all the magic happens.
import py5 def setup(): # Set up the canvas py5.size(800, 600) py5.background(255) def draw(): # This function runs continuously py5.ellipse(py5.mouse_x, py5.mouse_y, 50, 50) py5.run_sketch()
Here, we've created a simple sketch with a white background. The draw
function is called continuously, and it draws an ellipse where your mouse is located. It's as if the ellipse is chasing your cursor!
Basic Shapes
Animations begin with shapes. Let's start with some basic ones: circles, rectangles, and lines.
Drawing a Circle
def draw_circle(): py5.ellipse(400, 300, 100, 100)
Drawing a Rectangle
def draw_rectangle(): py5.rect(350, 250, 100, 100)
Drawing a Line
def draw_line(): py5.line(100, 100, 700, 500)
Each of these functions draws a shape on the canvas. You can call them in the draw
function to see them appear.
Animating Shapes
The real fun begins when we animate these shapes. Animation is simply drawing shapes at different positions over time.
Moving a Circle Across the Screen
x = 0 def draw(): global x py5.background(255) py5.ellipse(x, 300, 50, 50) x += 2 if x > py5.width: x = 0
In this example, a circle moves horizontally across the screen. We update the x
position in each frame, creating the illusion of movement.
Adding Color
Animations are more engaging with color. You can set the fill color using py5.fill
.
def draw(): py5.background(255) py5.fill(255, 0, 0) # Red color py5.ellipse(py5.mouse_x, py5.mouse_y, 50, 50)
Here, the circle follows your mouse and is colored red. You can also use py5.stroke
to change the outline color.
Interactive Animations
Make your animations interactive by responding to mouse and keyboard events.
Changing Color on Mouse Click
color = (0, 0, 0) def mouse_pressed(): global color color = (py5.random(255), py5.random(255), py5.random(255)) def draw(): py5.background(255) py5.fill(*color) py5.ellipse(py5.mouse_x, py5.mouse_y, 50, 50)
When you click the mouse, the ellipse changes to a random color. The mouse_pressed
function is called whenever the mouse is clicked.
Creating Complex Animations
Combine shapes, colors, and interactions to create complex animations.
A Bouncing Ball
x, y = 400, 300 x_speed, y_speed = 3, 2 def draw(): global x, y, x_speed, y_speed py5.background(255) py5.ellipse(x, y, 50, 50) x += x_speed y += y_speed # Bounce off edges if x > py5.width - 25 or x < 25: x_speed *= -1 if y > py5.height - 25 or y < 25: y_speed *= -1
This example features a ball that bounces around the screen. The ball reverses direction when it hits the edges of the canvas.
Conclusion
With py5, the possibilities for creating animations are endless. You can start with simple shapes and gradually build up to more complex animations. Whether you're creating a bouncing ball or an interactive art piece, py5 provides the tools you need to make your ideas come to life.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Putting It All Together (psst, it's free!).
FAQ
How do I install py5?
You can install py5 using pip. Open your terminal or command prompt and run the command pip install py5
.
Can I create animations without a mouse?
Absolutely! You can animate shapes based on variables and timers instead of mouse interactions. The bouncing ball example is a good starting point.
How can I save my animations?
You can save individual frames using the py5.save_frame
function. For example, py5.save_frame("frame-####.png")
saves frames as images. You can then compile these images into a video using external tools.
Is it possible to use py5 with other Python libraries?
Yes, py5 can be integrated with other Python libraries. For example, you can use numpy for mathematical calculations or pandas for data manipulation in your animations.
Can I create 3D animations with py5?
Yes, py5 supports 3D graphics. You can use functions like py5.box
and py5.sphere
to create and animate 3D shapes.