Code Optimization Techniques
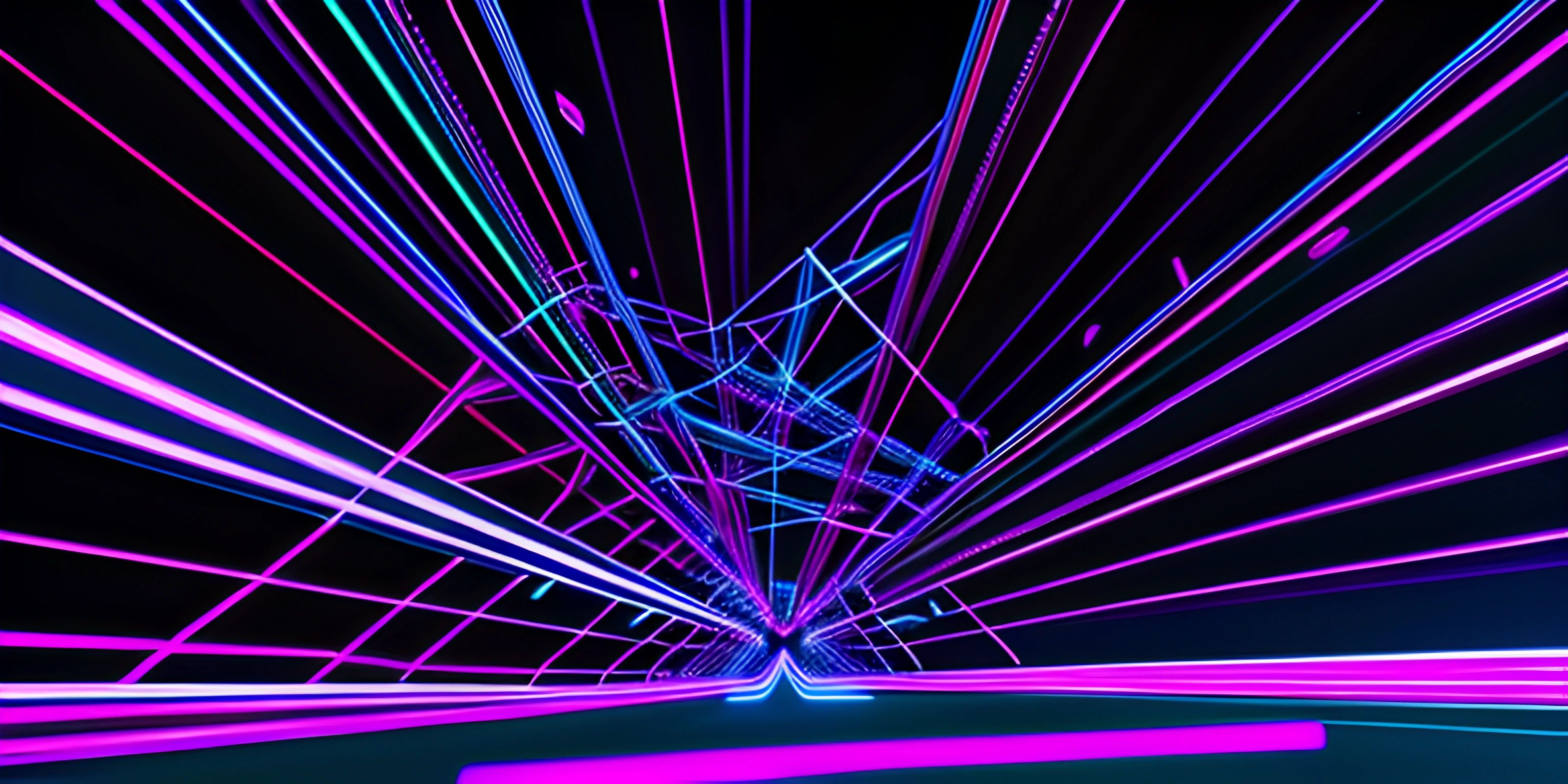
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
In this article, we will discuss various code optimization techniques that can help you improve the performance and efficiency of your programs. These techniques apply to both server-side (e.g., NodeJS) and client-side (e.g., browser JS) code.
1. Use Efficient Algorithms and Data Structures
Choosing the right algorithm and data structure can significantly impact the performance of your program. Make sure to analyze the time and space complexity of your algorithms and select the most appropriate data structure for your specific use case.
2. Minimize DOM Manipulation
When working with browser-based JavaScript, avoid excessive DOM manipulation, as it can lead to slow rendering and decreased performance. Instead, use efficient DOM manipulation libraries like jQuery or perform operations in memory before updating the DOM.
3. Use RequestAnimationFrame for Animations
For browser-based animations, use requestAnimationFrame
instead of setTimeout
or setInterval
. This function allows the browser to optimize the animation, leading to smoother animations and reduced CPU usage.
function animate() { // Update animation state // ... // Request the next frame requestAnimationFrame(animate); } requestAnimationFrame(animate);
4. Optimize Loops
Loops are often the source of performance bottlenecks. To optimize loops, consider:
- Using
for
loops instead offorEach
or other higher-order functions, as they tend to be faster. - Caching array lengths in a variable instead of calling the
length
property on each iteration. - Using reverse loops, which can sometimes be faster due to reduced overhead.
5. Minimize Global Variables
Global variables can slow down your code, as they require a longer scope chain traversal. Use local variables whenever possible, and consider using closures or modules to encapsulate your code.
6. Use Lazy Loading
Lazy loading is a technique where you only load resources (e.g., images, scripts, modules) when they are needed. This can help reduce the initial load time of your application and improve overall performance.
7. Minify and Compress Your Code
Minifying and compressing your code can help reduce file sizes and improve load times. Use tools like UglifyJS for JavaScript minification and gzip for compression.
8. Use Browser Caching
Leverage browser caching to store static assets like images, stylesheets, and scripts, so they don't have to be fetched on every page load. This can significantly improve load times and reduce server load.
9. Debounce and Throttle Functions
Debounce and throttle functions help control the rate at which a function is executed. This can be particularly useful for event handlers that might be triggered frequently, such as scroll or resize events.
function debounce(func, wait) { let timeout; return function() { clearTimeout(timeout); timeout = setTimeout(() => func.apply(this, arguments), wait); }; } function throttle(func, limit) { let inThrottle; return function() { if (!inThrottle) { func.apply(this, arguments); inThrottle = true; setTimeout(() => (inThrottle = false), limit); } }; }
By applying these code optimization techniques, you can improve the performance and efficiency of your programs, leading to better user experiences and more scalable applications.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Full-stack Web Frameworks (Next.js) (psst, it's free!).
FAQ
What are some common code optimization techniques?
Some common code optimization techniques include:
- Removing unnecessary code, also known as dead code elimination
- Simplifying complex expressions and calculations
- Loop unrolling to reduce the number of iterations
- Function inlining to avoid the overhead of function calls
- Memoization to store the results of expensive function calls and reuse them later
How can I optimize my code for better performance?
To optimize your code for better performance, you can:
- Profile your code to identify performance bottlenecks
- Apply optimization techniques like loop unrolling, memoization, and function inlining
- Optimize your data structures and algorithms, choosing the most efficient ones for your specific use case
- Utilize compiler optimizations, such as enabling optimization flags during compilation
- Consider parallel programming and hardware acceleration where applicable
What is the role of a compiler in code optimization?
A compiler plays a significant role in code optimization by automatically applying certain optimizations during the compilation process. Some of these optimizations include:
- Dead code elimination
- Constant folding and propagation
- Common subexpression elimination
- Loop optimizations, such as loop invariant code motion
- Function inlining Compiler optimization levels can usually be adjusted through compiler flags or options, allowing you to balance between fast compilation and optimized code.
Are there any downsides to aggressive code optimization?
Yes, there are potential downsides to aggressive code optimization:
- Code readability and maintainability might be sacrificed for performance gains
- Over-optimization can sometimes lead to unexpected bugs or issues
- Optimizations may be platform-specific, making the code less portable It's crucial to consider these trade-offs and ensure that optimizations are necessary and beneficial for your specific use case.
How does loop unrolling impact code performance?
Loop unrolling is a code optimization technique that reduces the overhead of loop iterations by replicating the loop's body multiple times, reducing the number of iterations. This can lead to improved code performance by:
- Reducing the number of loop control instructions executed (e.g., incrementing loop counter, checking loop condition)
- Allowing for better instruction-level parallelism
- Reducing branch mispredictions in modern processors However, excessive loop unrolling can increase code size and may not always lead to significant performance gains, so it's essential to use this technique judiciously.