Compiler Code Optimization
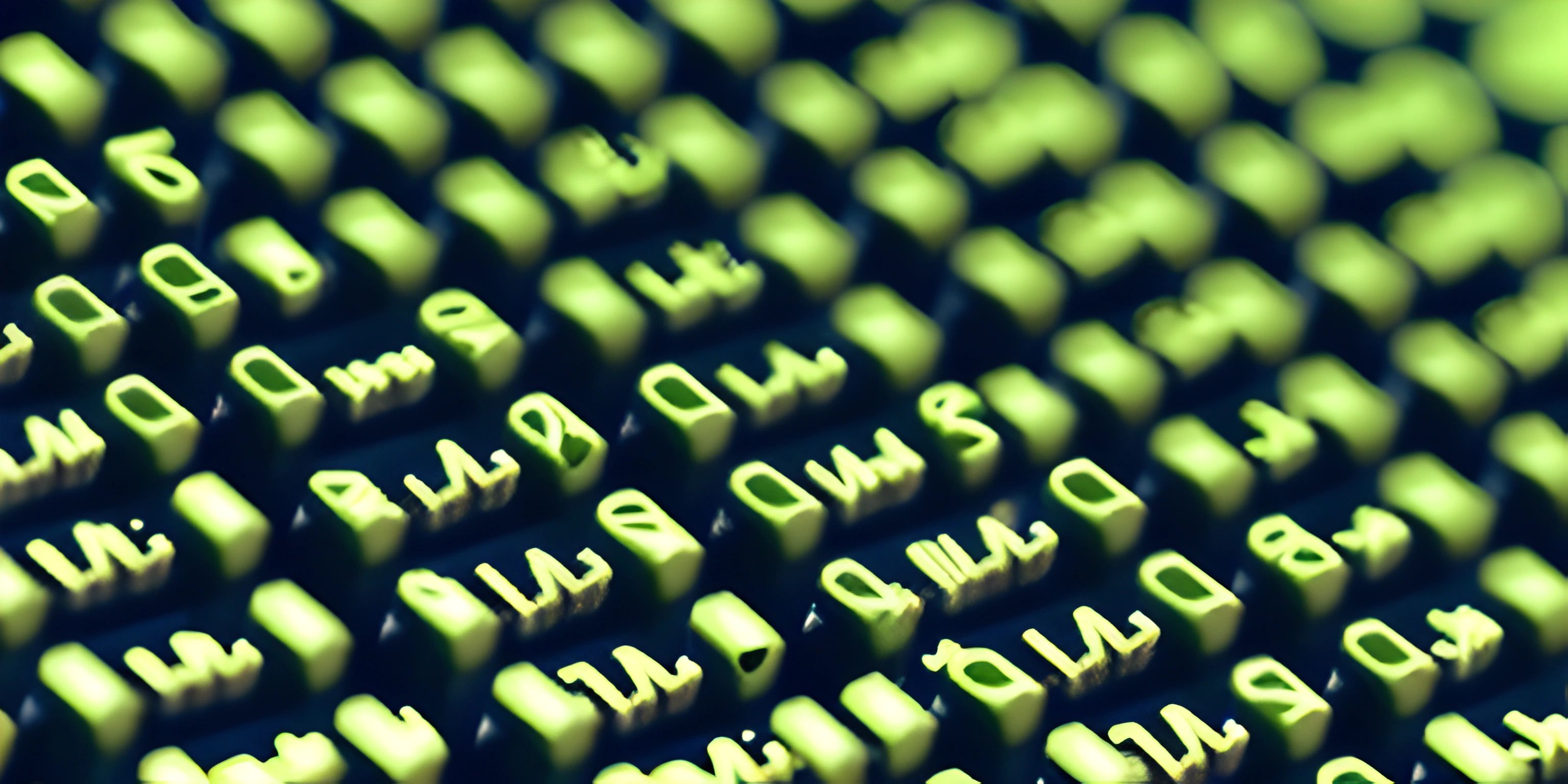
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
When working with compilers, an important aspect to consider is code optimization. Code optimization is the process of tweaking and modifying the source code to improve its efficiency and reduce the required execution time without affecting its functionality. The primary goal of code optimization is to enhance the performance of a program and minimize its resource consumption. In this article, we'll dive into the concept of code optimization in compiler design and explore some common techniques used to achieve it.
Why Code Optimization Matters
Code optimization is essential because it can lead to significant improvements in the performance and efficiency of a program. This becomes especially important when dealing with large-scale applications or systems with limited resources, such as embedded systems or mobile devices.
Efficient code can reduce the overall execution time, memory usage, and power consumption, leading to a more responsive application and improved user experience. Additionally, optimized code can help developers identify and eliminate potential bugs and bottlenecks in the system.
Compiler Optimization Techniques
There are several code optimization techniques used in compiler design. Some of these techniques work at the source code level, while others operate at the intermediate code or machine code level. Let's explore a few commonly used compiler optimization techniques.
1. Constant Folding
Constant folding is an optimization technique that evaluates constant expressions during the compile-time rather than runtime. This approach reduces the overall execution time, as the compiler can replace the constant expressions with their corresponding values directly in the compiled code.
For example, consider the following code snippet:
int a = 5 * 10;
With constant folding, the compiler can compute the result of 5 * 10
during compilation and replace it with 50
in the compiled code:
int a = 50;
2. Dead Code Elimination
Dead code elimination involves identifying and removing code statements that have no impact on the program's output. Dead code can occur due to unused variables, unreachable code, or redundant operations.
For instance, let's look at this code snippet:
int a = 5; int b = 10; int c = a * b; b = 20;
In this example, the assignment b = 20
has no impact on the value of c
and can be considered dead code. A compiler can safely remove the dead code without affecting the program's functionality.
3. Loop Unrolling
Loop unrolling is a technique that aims to reduce the overhead associated with loop structures. This optimization involves replicating the loop body multiple times with adjusted loop control variables, reducing the number of iterations required for the loop to execute.
For example, consider the following loop:
for (int i = 0; i < 4; i++) { print("Hello, world!"); }
An unrolled version of this loop would look like this:
print("Hello, world!"); print("Hello, world!"); print("Hello, world!"); print("Hello, world!");
By unrolling the loop, the compiler can eliminate the loop control structure and improve the execution speed.
Conclusion
Compiler code optimization plays a crucial role in enhancing the performance and efficiency of a program. By employing techniques such as constant folding, dead code elimination, and loop unrolling, compilers can generate more efficient and faster-executing code. As a programmer, understanding these optimization techniques can help you write better code and optimize your applications for various platforms and environments.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What is compiler code optimization?
Compiler code optimization is a process in which a compiler modifies the generated code to improve its efficiency and execution speed. This can involve various techniques like eliminating redundant code, reordering statements, and selecting faster alternatives. The main goal is to produce optimized code without affecting the correctness of the program, ensuring that it runs faster and uses fewer resources.
Why is code optimization important in compiler design?
Code optimization is crucial in compiler design because it can significantly impact the performance of a program. Efficient code can reduce execution time, lower memory usage, and even save on energy consumption. Optimized code is particularly important for resource-constrained systems, such as embedded devices or high-performance computing environments, where efficient resource utilization is critical to achieving the desired results.
Can you give examples of common compiler optimization techniques?
Certainly! Some common compiler optimization techniques include:
- Dead code elimination: This technique identifies and removes code that doesn't affect the program's output or doesn't get executed under any circumstances.
- Constant folding: This optimization involves evaluating constant expressions at compile time, reducing the amount of computation required at runtime.
- Loop unrolling: This technique duplicates the body of a loop a certain number of times, reducing the overhead of loop control and improving cache locality.
- Function inlining: This optimization replaces a function call with the actual code of the called function, eliminating the overhead of function calls and allowing further optimizations.
- Register allocation: This technique assigns frequently used values to CPU registers to minimize the number of memory accesses required during execution.
Do all compilers perform code optimization?
Most modern compilers, like GCC and LLVM, have built-in code optimization capabilities. However, the extent of optimization may vary depending on the compiler's settings and the target platform. Some compilers offer optimization levels that can be selected by the programmer, allowing them to balance code optimization with factors like compilation time and code size.
Can compiler optimization have any negative effects on the code?
While compiler optimization generally aims to improve code efficiency, there can be potential drawbacks:
- Increased code size: Some optimization techniques, like loop unrolling or function inlining, can lead to an increased code size, which might not be desirable for certain systems with limited memory.
- Difficulty in debugging: Optimized code can be harder to debug, as the optimized code may not correspond directly to the original source code.
- Possible correctness issues: Although rare, aggressive optimizations might introduce subtle bugs or change the behavior of the program in unexpected ways. To avoid these issues, it's essential to thoroughly test and verify the correctness of the optimized code and choose the appropriate optimization level depending on the specific requirements of the project.