Optimizing Code Performance
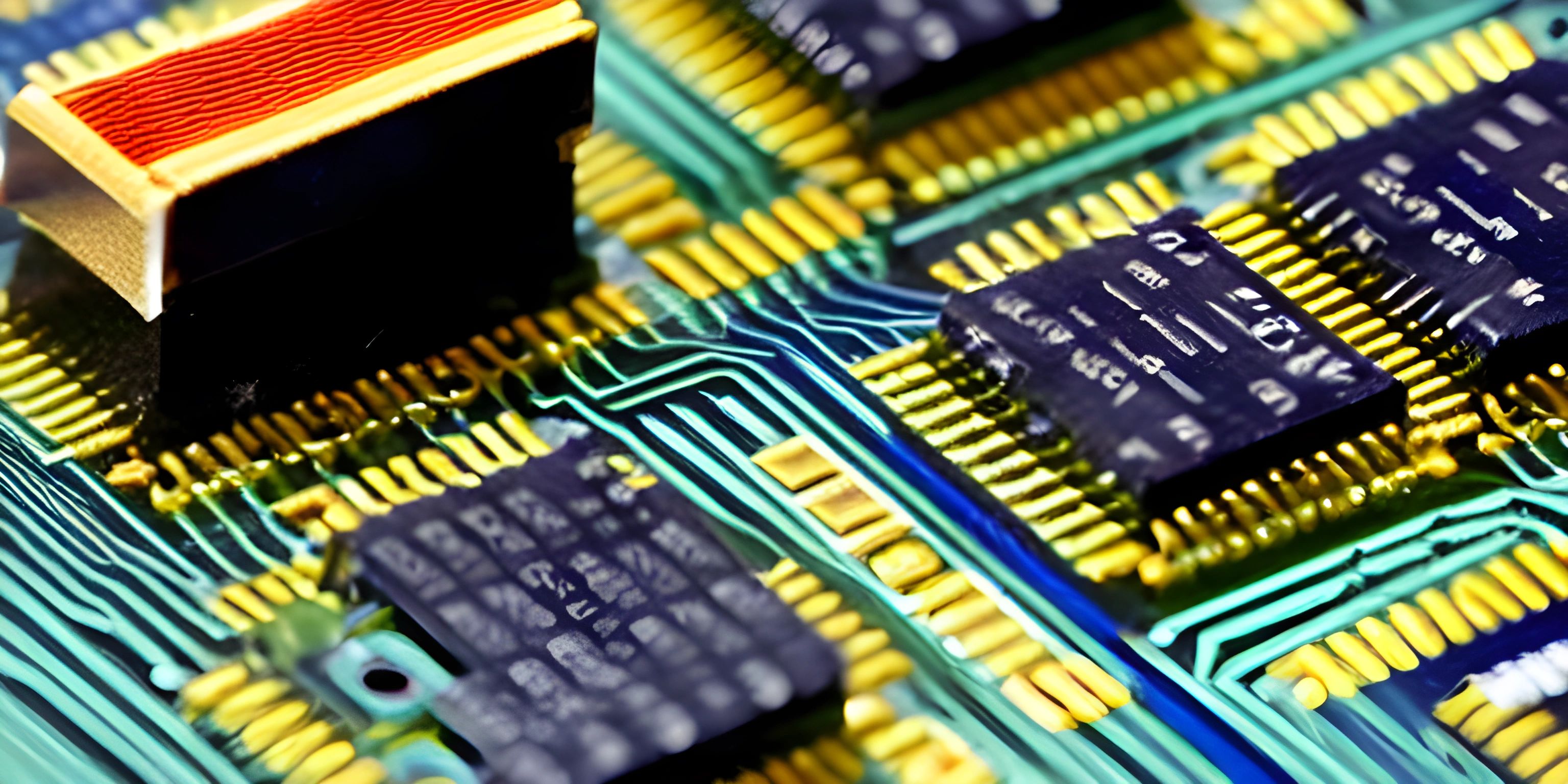
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
One important aspect of programming is writing code that runs quickly and efficiently. Just like a finely-tuned machine, efficient programs can save time, resources, and provide a better user experience. You don't want your users waiting for ages while your code executes, right? Let's dive into some techniques for optimizing code performance!
Time Complexity & Big O Notation
Understanding the concept of time complexity is essential when optimizing code performance. Time complexity represents the amount of time an algorithm takes to run relative to its input size. In programming, we often use Big O notation to describe an algorithm's time complexity.
For example, suppose we have an algorithm that takes an array of numbers as input and adds them up. The time complexity of this algorithm is O(n), where n is the number of elements in the array. As the input size increases, the time taken by the algorithm increases linearly.
def sum_array(numbers): total = 0 for num in numbers: total += num return total
In the example above, we loop through each element in the array, so the time complexity is O(n). When optimizing code, we aim to reduce the time complexity of our algorithms.
Using the Right Data Structures
Another critical aspect of optimizing code performance is choosing the right data structures. Data structures are the foundation upon which efficient algorithms are built. They dictate how data is organized, accessed, and manipulated.
For example, suppose we are searching for an element in a list. Using a linear search with a list, we have a time complexity of O(n). However, if we use a binary search on a sorted list, the time complexity reduces to O(log n).
Using data structures like hash tables can significantly improve the performance of operations like searching, inserting, and deleting elements.
Caching & Memoization
Caching is a technique where we store the results of expensive computations and reuse them later without having to recompute them. A specific form of caching, called memoization, is often used in recursion to optimize performance.
For example, let's look at a naive implementation of the Fibonacci sequence calculation:
def fib(n): if n <= 1: return n else: return fib(n - 1) + fib(n - 2)
The time complexity of this implementation is O(2^n). By using memoization, we can store the results of our previous calculations and reduce the time complexity to O(n).
def fib_memo(n, memo={}): if n <= 1: return n elif n not in memo: memo[n] = fib_memo(n - 1) + fib_memo(n - 2) return memo[n]
Profiling & Benchmarking
To optimize code performance, we must first identify bottlenecks in our code. Profiling involves measuring the time and resources used by our code, helping us understand where most of the time is spent. With this information, we can focus on optimizing these areas to yield the most significant performance improvements.
Benchmarking is another useful technique that involves comparing the performance of different implementations or algorithms, helping us make informed decisions on which approach to use.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What is time complexity?
Time complexity is a measure of the amount of time an algorithm takes to run relative to the size of its input. It helps us understand how the performance of an algorithm scales as the input size increases.
Why is it important to choose the right data structures?
Choosing the right data structures can significantly impact the efficiency of your code. Different data structures have varying performance characteristics, and using the appropriate one can help reduce the time complexity of your algorithms and improve overall code performance.
What is caching?
Caching is a technique where we store the results of expensive computations and reuse them later without having to recompute them. It helps in reducing the time taken by our code to execute and can significantly improve performance.
How can profiling and benchmarking help optimize code performance?
Profiling helps identify bottlenecks in our code by measuring the time and resources used, allowing us to focus on optimizing these areas. Benchmarking involves comparing the performance of different implementations or algorithms, helping us make informed decisions on which approach to use for better performance.