Code Generation in Compiler Design
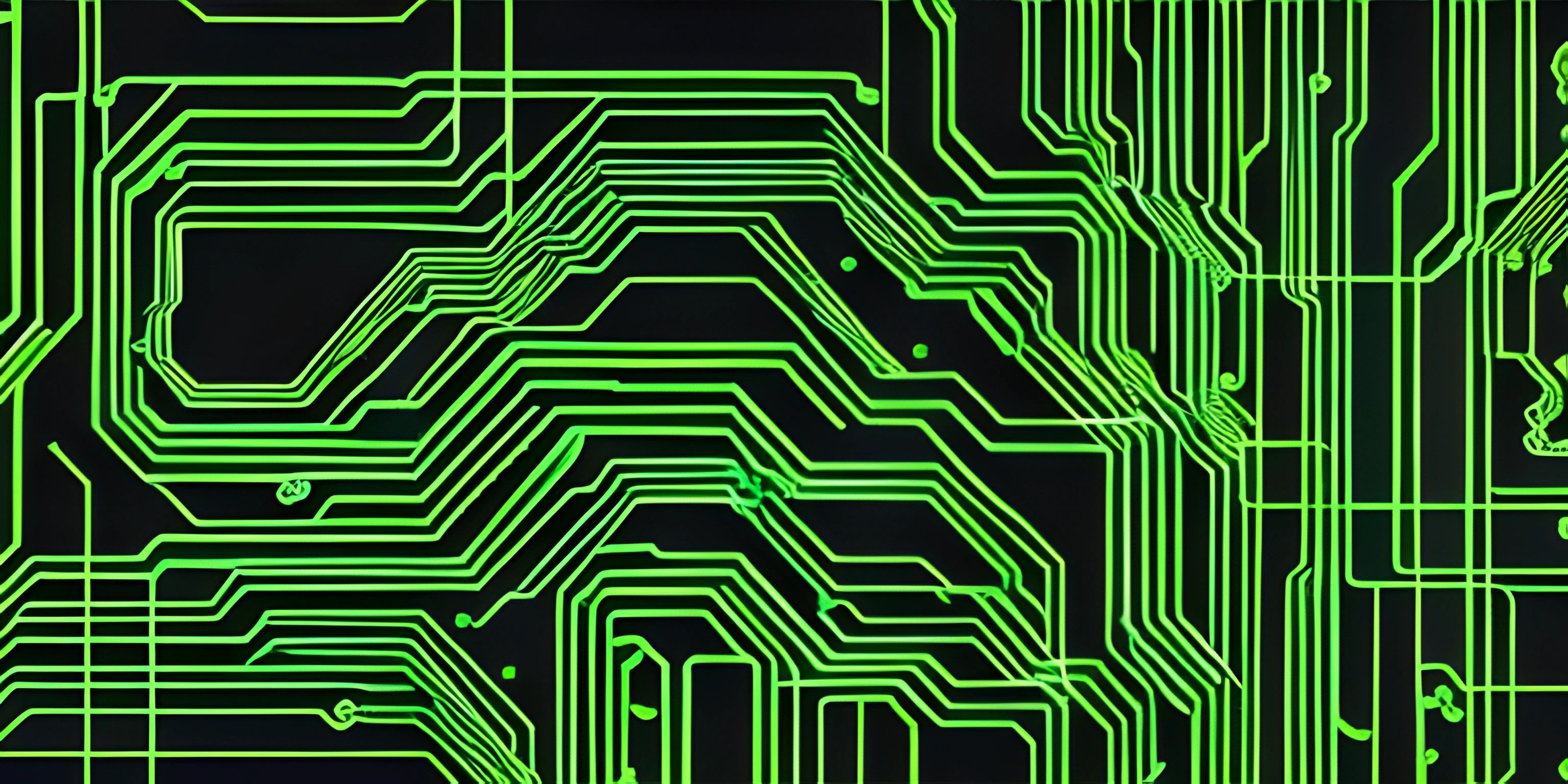
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
When diving into the world of programming, you might have come across the term "compiler" more than once. It's a crucial part of the software development process, as it takes your high-level code and translates it into machine-readable instructions. One significant aspect of compiler design is code generation, which is responsible for producing efficient and optimized code in the target language.
Intermediate Code
Before we delve into code generation, it's essential to understand the concept of intermediate code. As the name suggests, intermediate code is a middle ground between the high-level source code and the low-level target code. It's usually generated during the compilation process, and its primary purpose is to provide a platform-independent representation of the program.
By using intermediate code, compilers can focus on two different tasks: translating high-level code into intermediate code, and translating intermediate code into the target machine code. This separation simplifies the overall compilation process and allows for better optimization.
Three-Address Code
One popular intermediate code representation is the three-address code. This form of code typically has a single operation per instruction, and each instruction involves at most three memory locations or operands. Here's an example of three-address code for a simple expression:
a = b + c * d
The three-address code representation would be:
t1 = c * d a = b + t1
This intermediate code separates complex expressions into simpler instructions that can be easily optimized and translated into machine code.
Code Generation
Now that we have our intermediate code, it's time to generate the target code. The main objective of code generation is to produce efficient and optimized code. The process includes various techniques, such as register allocation, instruction selection, and optimizations that reduce the size and execution time of the generated code.
Register Allocation
One crucial aspect of code generation is register allocation. Registers are small, fast storage locations within the CPU that can hold data or operands for the instructions. Since they are limited in number and faster than memory, it's essential to use them efficiently. The register allocation process determines which variables should be assigned to registers and when to move data between registers and memory.
Instruction Selection
Another vital step in code generation is instruction selection. It involves choosing the most suitable machine instructions to represent the intermediate code. The goal is to minimize the size and improve the execution speed of the generated code. Instruction selection can be done using pattern matching, dynamic programming, or other optimization techniques.
Optimization Techniques
After generating the initial target code, compilers often apply various optimization techniques to improve its efficiency. Some common optimization techniques include:
- Constant folding: Evaluating constant expressions at compile-time, reducing the runtime overhead.
- Dead code elimination: Removing unreachable or unused code sections that do not affect the program's output.
- Loop optimizations: Applying transformations to loops, like loop unrolling or loop invariant code motion, to improve their execution speed.
These are just a few examples, and there are many other optimization techniques that compilers can employ to enhance the generated code performance.
In Conclusion
Code generation is a vital and fascinating aspect of compiler design. It takes intermediate code and converts it into efficient, optimized target code that the machine can understand and execute. By understanding the principles of code generation, you can gain valuable insights into how compilers work and appreciate the intricate process that turns your source code into a working program.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Async Rust (psst, it's free!).