Semantic Analysis in Compiler Design
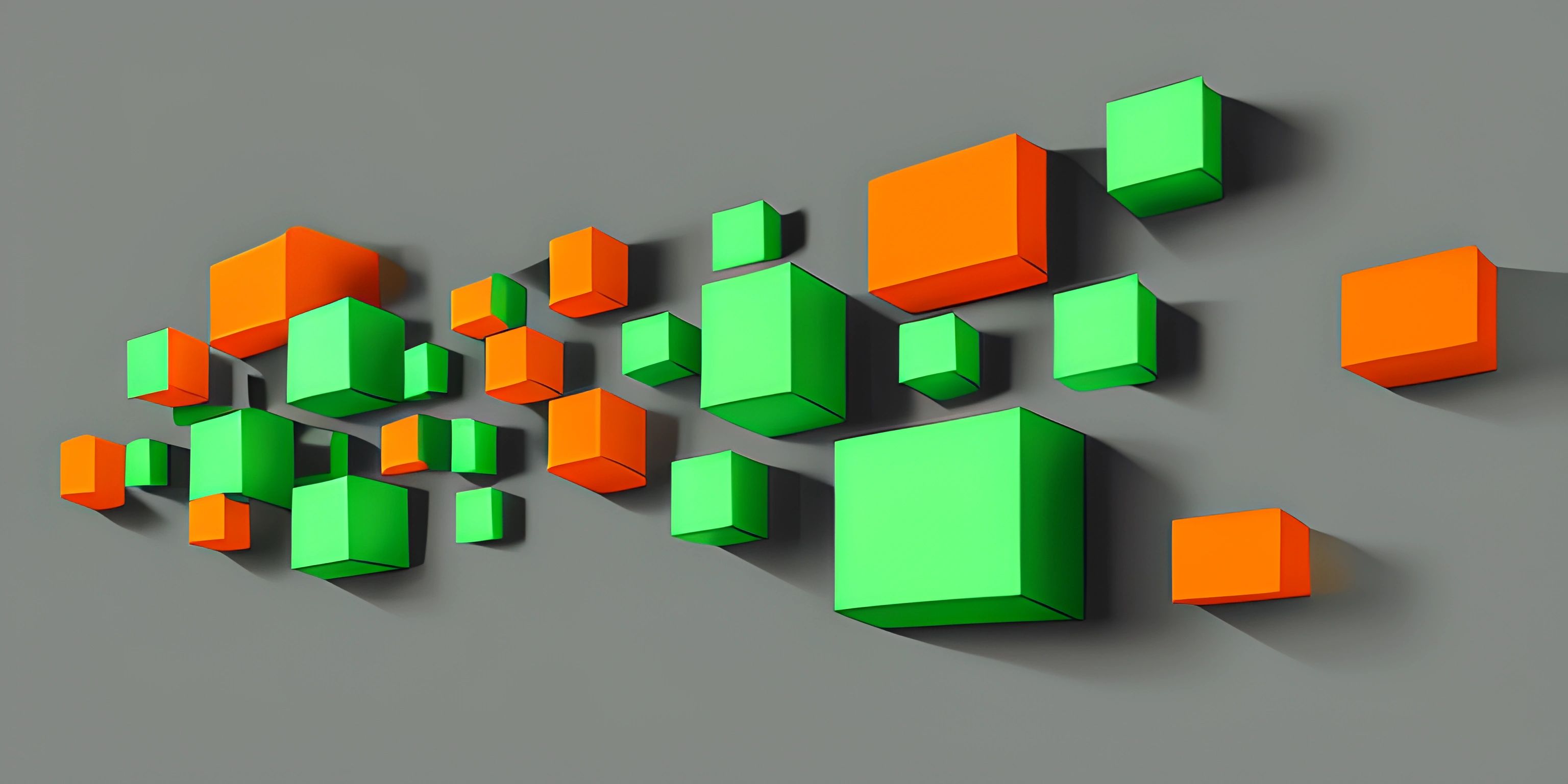
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Semantic analysis is a vital component in the compiler design process, ensuring that the code you write is not only syntactically correct but also semantically meaningful. So, buckle up as we dive into the world of semantic analysis and explore its importance in compiler design.
Syntax vs. Semantics
Before we understand semantic analysis, it's vital to distinguish between syntax and semantics. Syntax refers to the rules governing the structure of a code, dictating how different elements should be arranged. On the other hand, semantics deals with the meaning behind the code, ensuring that it makes sense in the given context.
Imagine a simple sentence: "The cat is on the mat." The syntax defines the order of the words and the use of punctuation, while the semantics ensures that the sentence makes sense.
The Role of Semantic Analysis
The primary goal of semantic analysis is to catch any errors in your code that are not related to syntax. While the syntax of your code might be perfect, it's still possible for it to be semantically incorrect. Semantic analysis checks your code to ensure it's logically sound and performs operations such as type checking, scope checking, and more.
Type Checking
One of the most crucial aspects of semantic analysis is type checking, which ensures that the types of variables and expressions used in your code are compatible. For example, attempting to add an integer and a string together would be a semantic error, as these data types are not compatible.
number = 42 text = "Hello, World!" result = number + text # Semantic error: Cannot add an integer and a string
Scope Checking
Scope checking is another essential function of semantic analysis. It ensures that variables and functions are used within their appropriate scope, preventing errors such as using a local variable outside its defined function.
def example_function(): local_variable = 10 print(local_variable) # Semantic error: local_variable is not defined in this scope
How Semantic Analysis Works
Semantic analysis is typically performed after the syntax analysis (also known as parsing) stage of the compiler design process. The syntax analysis generates an Abstract Syntax Tree (AST), which is a tree representation of the source code's structure.
The semantic analyzer then traverses the AST, checking for semantic errors and gathering necessary information about variables, functions, and their types. If any errors are detected, the process is halted, and an error message is provided to the developer.
Conclusion
Semantic analysis is a critical step in the compiler design process, ensuring that your code is not only syntactically correct but also semantically meaningful. By catching errors such as type and scope violations, semantic analysis helps developers write more robust and error-free code. So, the next time you see a compiler error, remember that it's the semantic analysis working hard to keep your code in tip-top shape!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Full-stack Web Frameworks (Next.js) (psst, it's free!).
FAQ
What is semantic analysis in compiler design?
In compiler design, semantic analysis refers to the process of examining the structure and meaning of source code to ensure its correctness. This step comes after the syntactic analysis (parsing) and focuses on checking for semantic errors, type checking, and validating the code against certain rules and constraints. Semantic analysis plays an essential role in producing error-free and efficient code.
How does semantic analysis help in ensuring code correctness?
Semantic analysis helps in ensuring code correctness by:
- Identifying semantic errors that may not be caught during syntax analysis, such as undefined variables or illegal operations.
- Performing type checking to ensure that the data types of variables and expressions are consistent and compatible.
- Enforcing language-specific rules and constraints that must be followed for the code to execute correctly. By performing these checks, semantic analysis aims to catch any potential issues that could lead to incorrect or unexpected behavior during code execution.
What are some common semantic errors in programming?
Some common semantic errors that may be identified during semantic analysis include:
- Using an undefined variable or function.
- Mismatched data types in expressions or assignments.
- Illegal operations, such as dividing by zero or using an invalid index in an array.
- Violating language-specific rules, like attempting to access a private member outside of its class.
- Incorrect usage of functions, such as passing incorrect arguments or forgetting to return a value.
What is type checking, and how does it relate to semantic analysis?
Type checking is a crucial aspect of semantic analysis that ensures the correct usage and compatibility of data types in a program. It checks the data types of variables, expressions, and function arguments to confirm that they are consistent with the expected data types. Type checking helps prevent various runtime errors, such as type conversion errors, and ensures that the code adheres to the language's type system.
Can you provide an example of a semantic error in a code snippet?
Sure! Here's an example of a semantic error in a Python code snippet:
def add_numbers(a, b): return a + b result = add_numbers("5", 10)
In this example, the add_numbers
function expects two numbers as arguments, but we've passed a string "5"
and an integer 10
. This code will run without syntax errors, but it will produce unexpected results due to the semantic error of passing incompatible types to the function.