Introduction to Python
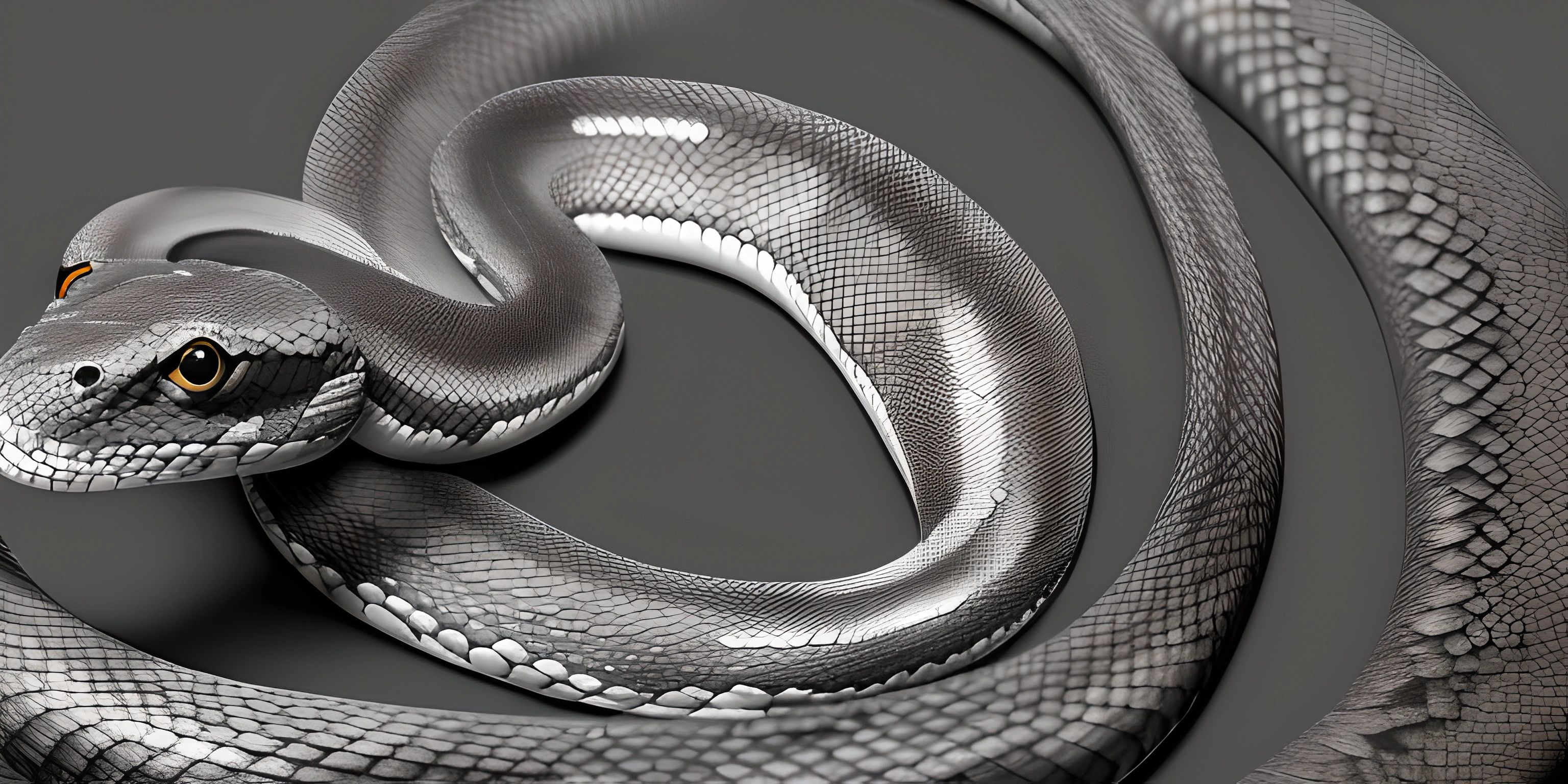
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Python is a programming language that's widely loved for its simplicity, readability, and versatility. It's like the Swiss army knife of programming languages. From web development to data analysis, machine learning to game development, Python has something for everyone.
Why Python?
There are several reasons why Python has become one of the most popular programming languages in the world:
- Easy-to-learn syntax: Python's syntax is designed to be readable and intuitive, making it a great choice for beginners.
- Extensive library support: Python has a vast ecosystem of libraries and frameworks that can help you accomplish almost any task.
- Cross-platform compatibility: Python code runs on just about any platform, including Windows, macOS, and Linux.
- Strong community: Python has a large, diverse, and welcoming community that's continually growing and evolving.
Getting Started with Python
Installing Python
To start using Python, you'll first need to install it on your computer. The official Python website provides detailed instructions for various operating systems, so you'll be up and running in no time.
Writing Your First Python Program
Once you have Python installed, it's time to write your first program! Open up a text editor, and type the following line:
print("Hello, World!")
Save this file with a .py
extension (for example, hello_world.py
) and then open up your terminal or command prompt. Navigate to the directory where you saved your file and run the following command:
python hello_world.py
If everything went smoothly, you should see "Hello, World!" printed on your screen. Congratulations, you've just written your first Python program!
Basic Python Concepts
Now that you've written your first Python program, let's take a look at some of the basic concepts you'll need to become more proficient in Python.
- Variables: In Python, you can use variables to store and manipulate data. For example:
name = "Alice" age = 30
-
Data Types: Python has several built-in data types, such as strings, integers, floats, lists, and dictionaries, which allow you to work with different types of data.
-
Control Structures: Python supports common control structures, like if-else statements, for loops, and while loops, that control the flow of your program.
-
Functions: You can use functions to group together lines of code that perform a specific task. Functions can help make your code more modular and reusable.
-
Classes and Objects: Python is an object-oriented programming language, which means you can use classes to create your own custom data structures and objects.
These are just a few of the many concepts you'll encounter as you learn Python. With a little practice, you'll soon be ready to tackle more advanced topics and build some amazing projects. So grab your favorite text editor, and let's get coding!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What is Python and why is it popular?
Python is a high-level, versatile programming language that's known for its readability, simplicity, and wide range of applications. It's perfect for beginners and experts, as it's easy to learn but powerful enough to handle complex tasks. Python's popularity is due to its extensive library support, developer community, and ease of integration with other technologies.
How do I install Python on my computer?
To install Python, follow these steps:
- Visit the official Python website at python.org.
- Click on "Downloads" in the top navigation bar.
- Choose the version appropriate for your operating system (Windows, macOS, or Linux).
- Follow the installation instructions specific to your chosen version and operating system.
How do I write and run my first Python program?
To write and run a Python program, follow these steps:
- Open a text editor (e.g., Notepad, Sublime Text, or Visual Studio Code).
- Type the following code:
print("Hello, World!")
- Save the file with a
.py
extension (e.g.,hello_world.py
). - Open a terminal or command prompt and navigate to the directory where you saved the file.
- Run the Python script by entering the following command:
python hello_world.py
- You should see "Hello, World!" printed in the terminal or command prompt.
What are some common Python data types?
Python has several built-in data types, including:
- Strings: A sequence of characters, e.g.,
"Hello, World!"
- Integers: Whole numbers, e.g.,
42
- Floats: Decimal numbers, e.g.,
3.14
- Lists: Ordered, mutable collections of values, e.g.,
[1, 2, 3]
- Tuples: Ordered, immutable collections of values, e.g.,
(1, 2, 3)
- Dictionaries: Unordered collections of key-value pairs, e.g.,
{"key": "value"}
How can I learn more about Python programming?
There are many resources available for learning Python. Some popular options include:
- The official Python documentation
- Online tutorials and courses, such as Real Python and Codecademy
- Python programming books, like "Python Crash Course" by Eric Matthes and "Automate the Boring Stuff with Python" by Al Sweigart
- Joining a Python community, such as the Python Reddit community or the Python Discord server for discussions and help with your Python journey.