Understanding and Implementing Depth First Search Algorithm
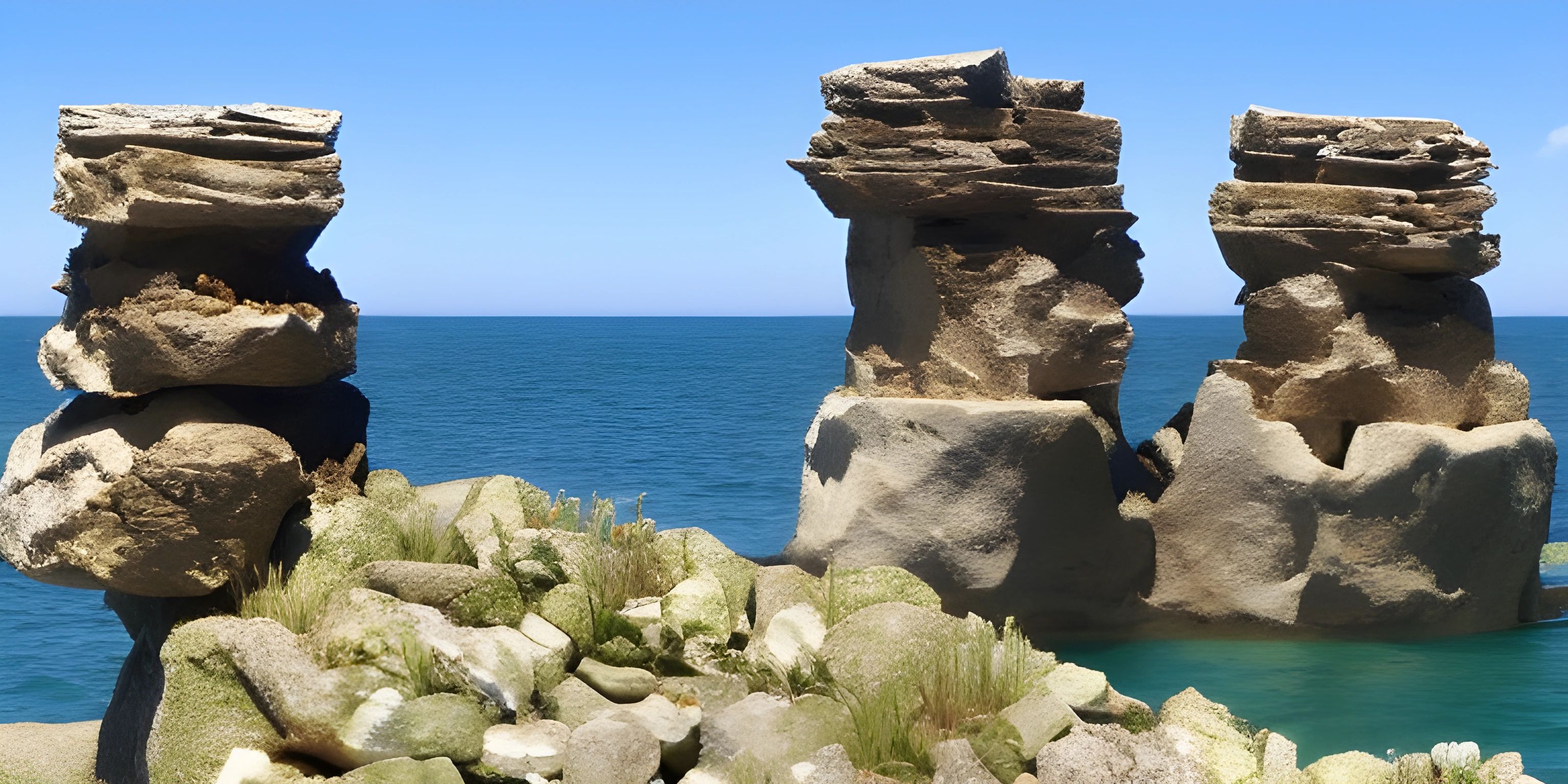
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Welcome to your new obsession: Depth First Search (DFS). This algorithm is like the Indiana Jones of graph traversal techniques. It ventures deep into the mysterious graphs, exploring one path exhaustively before backtracking to explore another. Whether you're into treasure hunting or just want to ace your next coding interview, understanding DFS is a key skill.
What is Depth First Search?
DFS is a graph traversal algorithm that starts at an arbitrary root node and explores as far as possible along each branch before backtracking. Imagine you're in a maze, and you decide to always go left until you hit a dead end. Then, you backtrack and try the next path on the right, and so on.
How Does DFS Work?
DFS uses a stack data structure—either implicitly with recursion or explicitly—to keep track of the nodes to be visited. Here’s a step-by-step process:
- Start at the root node: Add it to the stack (or call the DFS function on it).
- Visit the node: Mark it as visited and explore its adjacent nodes.
- Go deep: For each adjacent node, if it hasn't been visited, repeat the process.
- Backtrack: When you reach a node with no unvisited adjacent nodes, backtrack to the previous node.
DFS Pseudocode
Before diving into specific languages, let's look at the pseudocode:
DFS(node): if node is not visited: mark node as visited for each neighbor in node.neighbors: if neighbor is not visited: DFS(neighbor)
Implementing DFS in Python
Python is like a Swiss army knife for coding. Let's implement DFS using both recursive and iterative approaches.
Recursive DFS
Here's how you can implement DFS recursively in Python:
def dfs_recursive(graph, node, visited): if node not in visited: print(node) # Visit the node visited.add(node) for neighbor in graph[node]: dfs_recursive(graph, neighbor, visited) # Example usage graph = { 'A': ['B', 'C'], 'B': ['D', 'E'], 'C': ['F'], 'D': [], 'E': ['F'], 'F': [] } visited = set() dfs_recursive(graph, 'A', visited)
Iterative DFS
For those who prefer loops over recursion, here's the iterative version:
def dfs_iterative(graph, start): visited = set() stack = [start] while stack: node = stack.pop() if node not in visited: print(node) # Visit the node visited.add(node) stack.extend(reversed(graph[node])) # Add neighbors to the stack # Example usage graph = { 'A': ['B', 'C'], 'B': ['D', 'E'], 'C': ['F'], 'D': [], 'E': ['F'], 'F': [] } dfs_iterative(graph, 'A')
Implementing DFS in Java
Java's strict type system and syntax make it a bit more verbose but equally powerful. Let's see both recursive and iterative DFS in Java.
Recursive DFS
import java.util.*; public class DFS { private Map<String, List<String>> graph = new HashMap<>(); public void addEdge(String node, String neighbor) { graph.computeIfAbsent(node, k -> new ArrayList<>()).add(neighbor); } public void dfsRecursive(String node, Set<String> visited) { if (!visited.contains(node)) { System.out.println(node); // Visit the node visited.add(node); for (String neighbor : graph.getOrDefault(node, new ArrayList<>())) { dfsRecursive(neighbor, visited); } } } public static void main(String[] args) { DFS dfs = new DFS(); dfs.addEdge("A", "B"); dfs.addEdge("A", "C"); dfs.addEdge("B", "D"); dfs.addEdge("B", "E"); dfs.addEdge("C", "F"); Set<String> visited = new HashSet<>(); dfs.dfsRecursive("A", visited); } }
Iterative DFS
import java.util.*; public class DFS { private Map<String, List<String>> graph = new HashMap<>(); public void addEdge(String node, String neighbor) { graph.computeIfAbsent(node, k -> new ArrayList<>()).add(neighbor); } public void dfsIterative(String start) { Set<String> visited = new HashSet<>(); Stack<String> stack = new Stack<>(); stack.push(start); while (!stack.isEmpty()) { String node = stack.pop(); if (!visited.contains(node)) { System.out.println(node); // Visit the node visited.add(node); List<String> neighbors = graph.getOrDefault(node, new ArrayList<>()); Collections.reverse(neighbors); for (String neighbor : neighbors) { stack.push(neighbor); } } } } public static void main(String[] args) { DFS dfs = new DFS(); dfs.addEdge("A", "B"); dfs.addEdge("A", "C"); dfs.addEdge("B", "D"); dfs.addEdge("B", "E"); dfs.addEdge("C", "F"); dfs.dfsIterative("A"); } }
Implementing DFS in JavaScript
JavaScript, known for its flexibility, is also a great language for implementing DFS. Let's see how it can be done.
Recursive DFS
function dfsRecursive(graph, node, visited = new Set()) { if (!visited.has(node)) { console.log(node); // Visit the node visited.add(node); graph[node].forEach(neighbor => { dfsRecursive(graph, neighbor, visited); }); } } // Example usage const graph = { 'A': ['B', 'C'], 'B': ['D', 'E'], 'C': ['F'], 'D': [], 'E': ['F'], 'F': [] }; dfsRecursive(graph, 'A');
Iterative DFS
function dfsIterative(graph, start) { const visited = new Set(); const stack = [start]; while (stack.length) { const node = stack.pop(); if (!visited.has(node)) { console.log(node); // Visit the node visited.add(node); graph[node].slice().reverse().forEach(neighbor => { stack.push(neighbor); }); } } } // Example usage const graph = { 'A': ['B', 'C'], 'B': ['D', 'E'], 'C': ['F'], 'D': [], 'E': ['F'], 'F': [] }; dfsIterative(graph, 'A');
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Smiley Face (psst, it's free!).
FAQ
What are the main differences between DFS and BFS?
DFS (Depth First Search) explores as deep as possible along each branch before backtracking, while BFS (Breadth First Search) explores all neighbors at the present depth before moving on to nodes at the next depth level. DFS uses a stack, whereas BFS uses a queue.
When should I use DFS over BFS?
Use DFS when you need to explore all possible paths or when the solution is likely to be deep within the search space. DFS is also useful for topological sorting, solving puzzles like mazes, and finding strongly connected components in a graph.
What are some common applications of DFS?
DFS is commonly used for detecting cycles in graphs, pathfinding, topological sorting, finding connected components, solving puzzles, and in algorithms like Tarjan's and Kosaraju's for finding strongly connected components.
Can DFS be used on weighted graphs?
Yes, DFS can be used on weighted graphs, but it does not take weights into account. For pathfinding in weighted graphs, algorithms like Dijkstra's or A* are more appropriate.
What is the time complexity of DFS?
The time complexity of DFS is O(V + E), where V is the number of vertices and E is the number of edges in the graph. This is because each vertex and edge is explored once.