Graph Algorithms
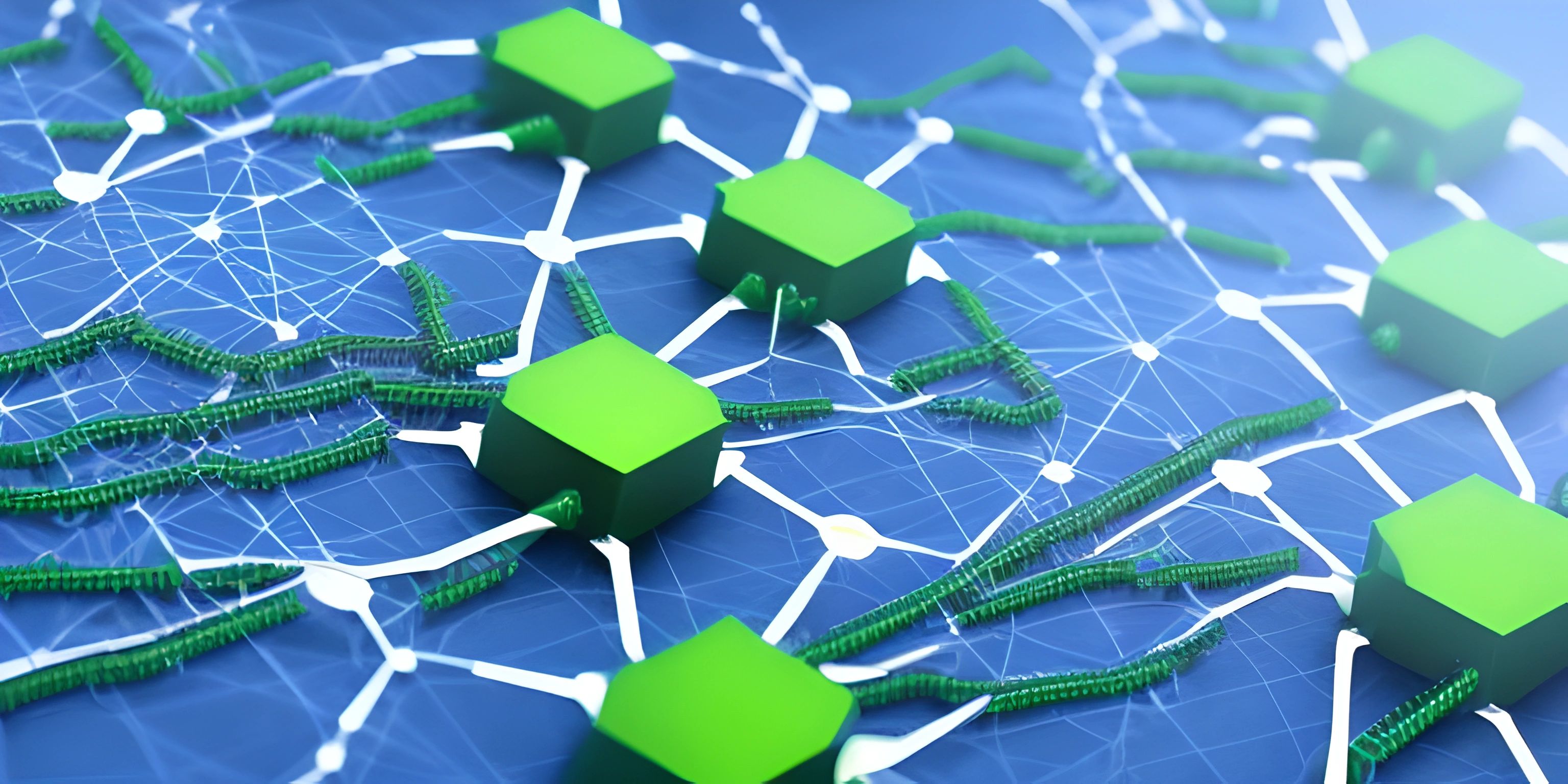
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Graphs are a powerful and versatile data structure that can be used to represent various relationships and connections. As a result, many problems can be modeled using graphs, and graph algorithms are essential tools in a programmer's toolbox. In this article, we'll cover some of the most common graph algorithms, their implementations, and their applications in solving real-world problems.
Graph Basics
A graph is a collection of nodes (also called vertices) connected by edges. There are two main types of graphs - directed and undirected. In a directed graph, edges have a direction, which means that the connection between two nodes has a specific order. In an undirected graph, edges don't have a direction, so the connection between two nodes is bidirectional.
Representing Graphs
There are two common ways to represent graphs in code: adjacency lists and adjacency matrices.
An adjacency list is a collection of lists, one for each node in the graph. Each list contains the neighbors of the corresponding node. This representation is space-efficient and is typically used when the graph is sparse (i.e., has few edges).
An adjacency matrix is a two-dimensional array, with the number of rows and columns equal to the number of nodes in the graph. Each entry at position (i, j)
indicates the presence (or absence) of an edge between nodes i
and j
. This representation is more suitable for dense graphs (i.e., with many edges) but consumes more memory.
Depth-First Search (DFS)
Depth-first search (DFS) is a graph traversal algorithm that starts at a given node and explores as deep as possible along each branch before backtracking. It can be implemented using recursion or with an explicit stack. Here's an example implementation using recursion:
def dfs(graph, node, visited): if node in visited: return visited.add(node) print("Visiting node", node) for neighbor in graph[node]: dfs(graph, neighbor, visited) graph = { 'A': ['B', 'C'], 'B': ['D', 'E'], 'C': ['F'], 'D': [], 'E': ['F'], 'F': [] } visited = set() dfs(graph, 'A', visited)
Breadth-First Search (BFS)
Breadth-first search (BFS) is another graph traversal algorithm that starts at a given node and explores all the neighbors of the node before moving on to explore their neighbors. BFS can be implemented using a queue. Here's an example implementation:
from collections import deque def bfs(graph, start): visited = set() queue = deque([start]) while queue: node = queue.popleft() if node not in visited: visited.add(node) print("Visiting node", node) queue.extend(neighbor for neighbor in graph[node] if neighbor not in visited) bfs(graph, 'A')
Shortest Path Algorithms
Finding the shortest path between two nodes in a graph is a common problem. There are several algorithms for solving this problem, such as Dijkstra's algorithm and the Bellman-Ford algorithm. These algorithms can handle graphs with weighted edges, where each edge has an associated cost.
Dijkstra's algorithm is efficient for graphs with non-negative edge weights, while the Bellman-Ford algorithm can handle graphs with negative edge weights but is less efficient.
Conclusion
There are many graph algorithms available to solve a wide variety of problems. Understanding and implementing these algorithms can greatly expand your problem-solving capabilities as a programmer. Don't be afraid to dive deeper into each algorithm, as it will only make you a more effective and versatile programmer.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Recursion Intro (psst, it's free!).