Search Algorithms: Binary and Linear
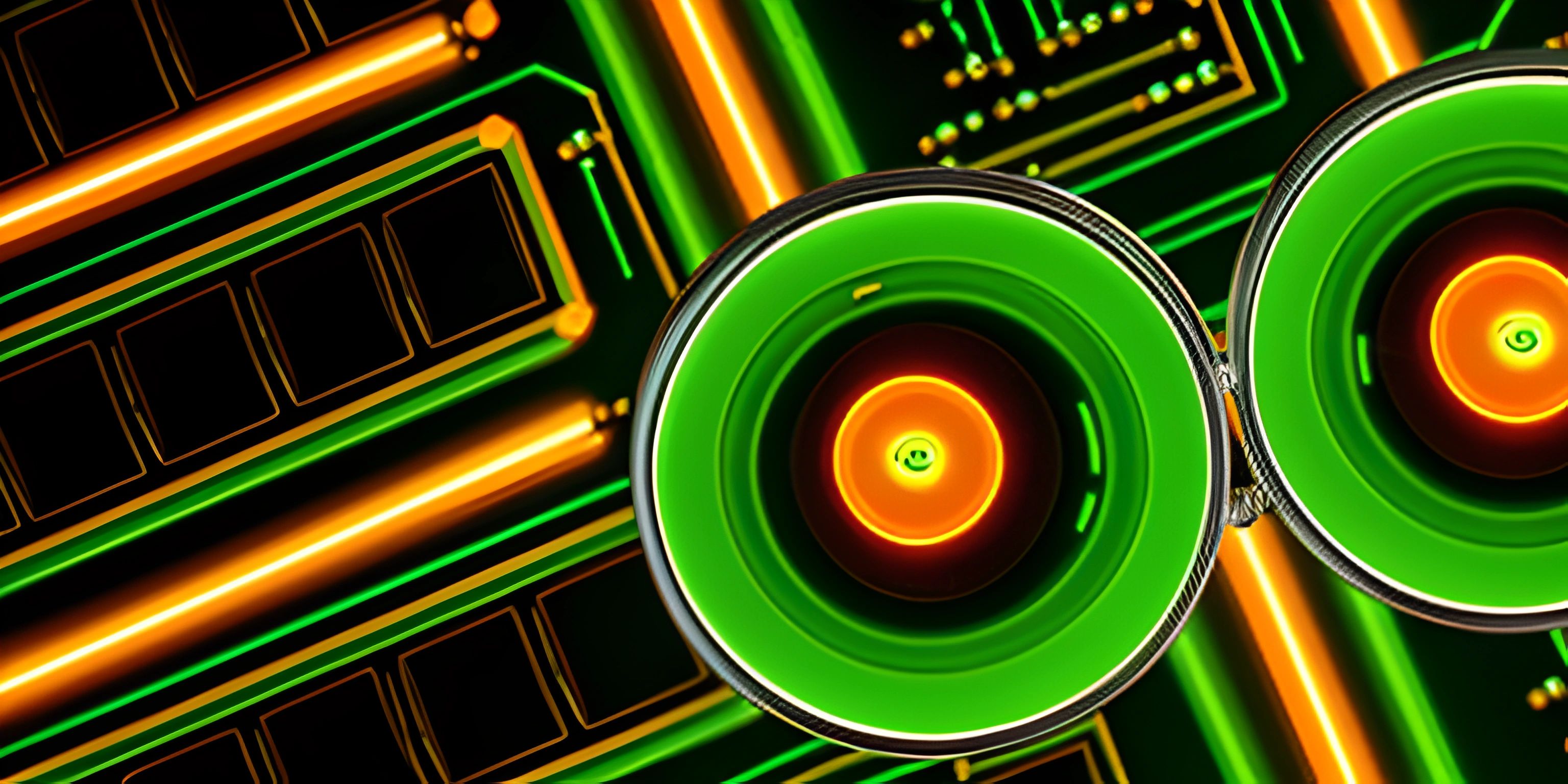
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Searching for specific items in a collection is a common task in programming, and having the right tool for the job is essential. In this case, the tools are search algorithms, and we're going to explore two popular ones: binary search and linear search. By the end of this article, you'll have a better understanding of their mechanics and when to use each one.
Linear Search
A linear search is the simplest and most intuitive search algorithm. It involves going through each item in a collection, one by one, until the desired item is found or the list ends. Let's see how it works using a list of numbers:
def linear_search(numbers, target): for index, number in enumerate(numbers): if number == target: return index return -1 numbers = [4, 2, 7, 1, 9, 3, 6] result = linear_search(numbers, 7) print(result) # Output: 2
In this example, the linear_search
function goes through each element in the numbers
list. When it finds the target number (7), it returns the index (2) at which it was found. If the target is not in the list, the function returns -1, indicating that the search was unsuccessful.
Linear search's simplicity makes it a good choice when you don't have any information about the order of the items in the collection or when you're dealing with small datasets.
Binary Search
For more substantial and sorted collections, the binary search algorithm proves to be much more efficient. Binary search works by repeatedly dividing the search interval in half, allowing it to quickly narrow down the location of the desired item.
Here's how binary search works with a sorted list of numbers:
def binary_search(numbers, target): left, right = 0, len(numbers) - 1 while left <= right: middle = (left + right) // 2 middle_value = numbers[middle] if middle_value == target: return middle elif middle_value < target: left = middle + 1 else: right = middle - 1 return -1 numbers = [1, 2, 3, 4, 6, 7, 9] result = binary_search(numbers, 4) print(result) # Output: 3
In this example, the binary_search
function starts with the entire sorted list and calculates the middle index. If the middle value is equal to the target, the search is successful, and the index is returned. If the middle value is less than the target, the search continues on the right side of the list, discarding the left side. Conversely, if the middle value is greater than the target, the search continues on the left side, discarding the right side. If the search reaches a point where the left and right boundaries cross, it means the target is not in the list, and the function returns -1.
Binary search is much faster than linear search for large datasets and when the collection is sorted. However, it's essential to keep in mind that binary search is not suitable for unsorted lists, as it relies on the sorted nature of the collection to efficiently locate the target item.
Conclusion
Both linear and binary search algorithms have their use cases, strengths, and weaknesses. Linear search is simple and suitable for small or unordered datasets, while binary search leverages the sorted nature of a collection to vastly improve search efficiency for larger datasets. By understanding their mechanics and when to use each one, you'll be better equipped to tackle search-related problems in your programming projects.
FAQ
What are the differences between binary search and linear search algorithms?
Binary search and linear search are both algorithms used to search for a particular element in a list or array. Here are the main differences between the two:
- Binary search is an efficient algorithm that works on sorted lists by repeatedly dividing the search interval in half, whereas linear search works on both sorted and unsorted lists by checking each element sequentially.
- Binary search has a faster average case time complexity of O(log n), while linear search has an average case time complexity of O(n).
- Binary search requires the list to be sorted before performing the search, whereas linear search can work with both sorted and unsorted lists.
How does binary search work, and when is it best to use it?
Binary search works by repeatedly dividing the search interval in half. At each step, the algorithm compares the middle element of the interval to the target value. If the middle element is the target, the search is successful. If the middle element is less than the target, the search continues in the right half of the interval. If the middle element is greater than the target, the search continues in the left half of the interval. Binary search is best to use when:
- The list is already sorted or can be sorted quickly.
- You need to perform a large number of searches on the same list.
- Time complexity is an important factor, as binary search has a faster average case time complexity of O(log n).
How does linear search work, and when is it best to use it?
Linear search works by checking each element of the list sequentially, starting from the first element, until it finds the target value or reaches the end of the list. Linear search is best to use when:
- The list is small or the search is performed infrequently.
- You don't know if the list is sorted or not.
- The cost of sorting the list before using binary search is too high.
Can binary search be used with unsorted lists?
No, binary search cannot be used with unsorted lists. Binary search relies on the fact that the list is sorted to efficiently divide the search interval in half at each step. Using binary search on an unsorted list may lead to incorrect results or failure to find the target element even if it exists in the list.