Introduction to Discord.py
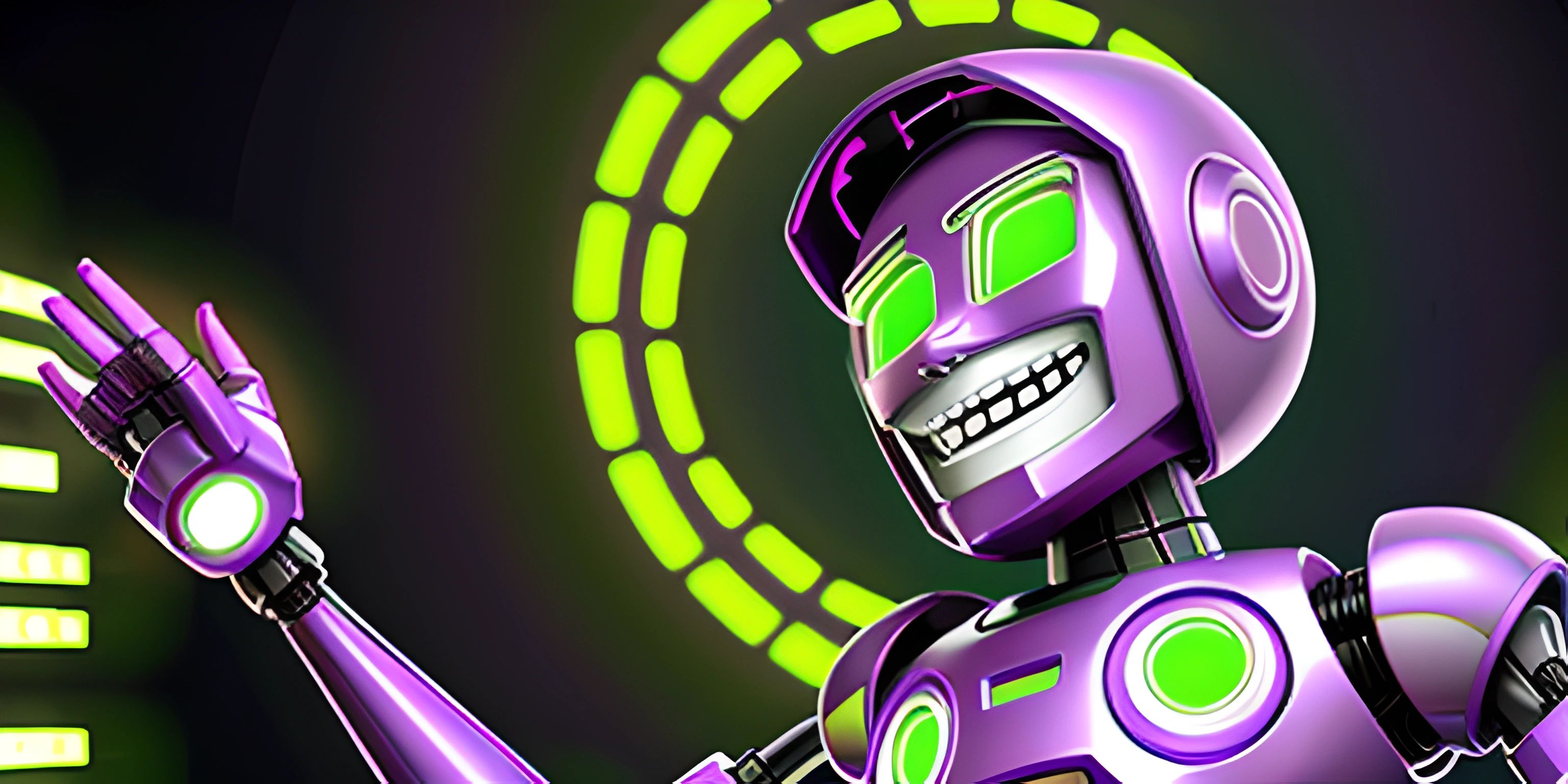
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
If you're looking to create a Discord bot that can interact with users, manage servers, or perform various tasks, then look no further than Discord.py. This popular Python library simplifies the process of creating bots, allowing you to focus on what truly matters: making your bot as engaging and delightful as a basket of digital kittens.
Installation
Before we can start using Discord.py, we'll need to install it. Make sure you have Python installed on your system (version 3.8 or newer is recommended). Then, open your terminal or command prompt and run the following command:
pip install discord.py
This will install Discord.py and its dependencies, such as aiohttp
and websockets
.
Creating Your First Bot
Now that we have Discord.py installed, it's time to unleash our inner bot-making wizard! First, we need to import the library and create a bot instance.
import discord from discord.ext import commands # Initialize the bot with the desired command prefix bot = commands.Bot(command_prefix="!") # Replace "your-bot-token" with your bot's token TOKEN = "your-bot-token" # Run the bot with the token bot.run(TOKEN)
Replace "your-bot-token"
with your actual bot token, which you can obtain by creating a new bot on the Discord Developer Portal.
Adding Commands
Commands are the bread and butter of Discord bots. They're the magical words that trigger your bot to perform a specific task. Let's create a simple ping
command that replies with "Pong!" when called.
@bot.command() async def ping(ctx): await ctx.send("Pong!")
Here, we use the @bot.command()
decorator to define a new command named ping
. The ctx
parameter is the context of the command, which contains information about the message, the author, and more.
Running the Bot
With your first command in place, it's time to bring your bot to life! Save your script and run it. If you've done everything correctly, you'll see your bot come online in your Discord server. Try typing !ping
and watch your bot reply with "Pong!".
Congratulations! You've just taken your first step into the wonderful world of Discord.py. There's so much more to explore, such as event listeners, embeds, and permissions. So, raise your wand and continue your journey to become a true Discord.py master!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Making a Basic Discord Bot in JavaScript (discord.js) (psst, it's free!).
FAQ
What is Discord.py and why should I use it?
Discord.py is a powerful and easy-to-use Python library for creating Discord bots. It allows you to build bots with various functionalities, such as moderating servers, playing music, or sending fun messages. By using Discord.py, you can create custom bots that cater to your server's specific needs and automate tasks, making your Discord experience more enjoyable and efficient.
How do I install Discord.py?
To install Discord.py, you will need Python 3.5.3 or higher. You can then install the library using pip, a package manager for Python. Run the following command in your terminal or command prompt:
pip install discord.py
This will install the latest version of Discord.py, allowing you to start building your Discord bot.
How do I create a simple Discord bot using Discord.py?
To create a simple Discord bot, follow these steps:
- Import the necessary libraries:
import discord from discord.ext import commands
- Create a bot instance and set a command prefix:
bot = commands.Bot(command_prefix="!")
- Define a simple command:
@bot.command() async def hello(ctx): await ctx.send("Hello, I'm your friendly Discord bot!")
- Add the bot's token and run the bot:
bot.run("your_bot_token_here")
Replace "your_bot_token_here" with your bot's actual token, which can be obtained from the Discord Developer Portal.
5. Invite the bot to your server and test the hello command by typing !hello
in a text channel.
Can I add more complex functionalities to my Discord bot using Discord.py?
Absolutely! Discord.py is a powerful library that allows you to create bots with various functionalities, from simple text responses to advanced features like playing music, moderating servers, or interacting with APIs. The more you explore the library and its documentation, the more complex and feature-rich bots you'll be able to create.
Where can I find resources and support for using Discord.py?
The official Discord.py documentation (https://discordpy.readthedocs.io/) is a great resource to start with. It provides comprehensive information about the library's functions and features. Additionally, you can join the Discord.py server (https://discord.gg/dpy) to ask questions, share your projects, and interact with other developers who are also working with Discord.py.