Adding Reactions to Messages with Discord.js
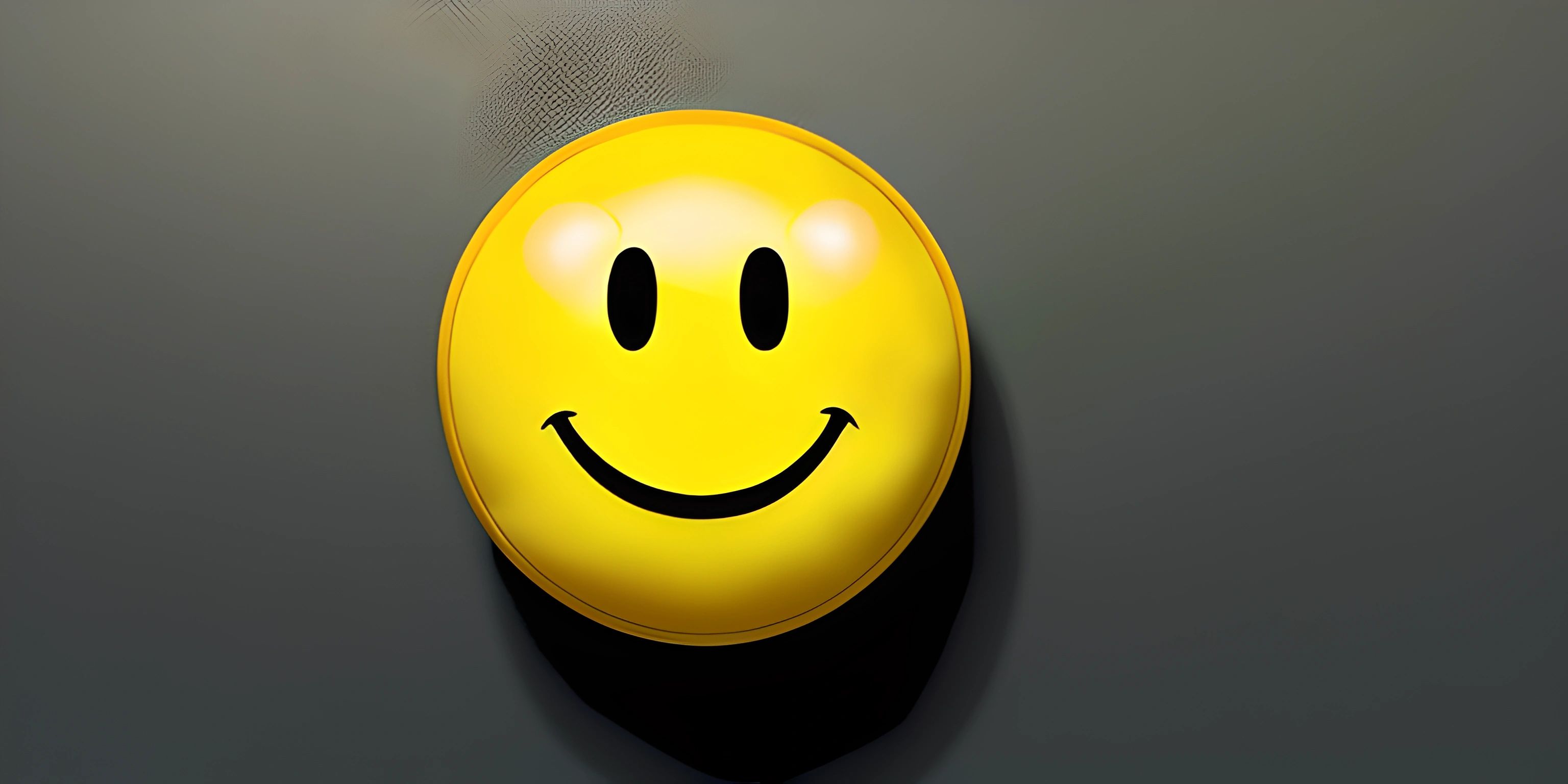
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
So you've got a Discord bot up and running, and now you want to give it the power to add some reactions to messages. Worry not, brave developer! We're about to dive into the wonderful world of message reactions using the popular Discord.js library.
Reacting to a Message
The first thing we need to do is teach our bot how to add reactions to messages. In Discord.js, we can use the Message
object's react()
method. For example, let's say we want to add a thumbs up (👍) reaction to a message:
// Assuming `message` is a Message object message.react("👍");
That's it! Our bot will now add a 👍 reaction to the message. But what if we want to use a custom emoji from the server? We'll need to fetch the emoji by its ID and then pass it to the react()
method:
// Assuming `emojiId` is the ID of a custom emoji const customEmoji = message.guild.emojis.cache.get(emojiId); message.react(customEmoji);
Now our bot can add custom emojis as reactions too!
Managing Reactions
Adding reactions is great, but sometimes we might want to remove them or check who reacted to a message. Discord.js has got us covered on that front as well.
Removing Reactions
To remove a specific reaction, we can use the remove()
method from the MessageReaction
object:
// Assuming `messageReaction` is a MessageReaction object messageReaction.remove();
If we want to remove all reactions from a message, we can use the removeAll()
method:
// Assuming `message` is a Message object message.reactions.removeAll();
Fetching Users Who Reacted
To see who reacted to a message, we can fetch the users from the MessageReaction
object:
// Assuming `messageReaction` is a MessageReaction object messageReaction.users.fetch().then(users => { console.log(`The following users reacted: ${users.map(u => u.username).join(", ")}`); });
This will log the usernames of all users who reacted with the specific emoji represented by the messageReaction
object.
Reacting to User Reactions
Now, let's say we want our bot to perform some action whenever a user reacts to a specific message. We can use the messageReactionAdd
event from the Client
object:
// Assuming `client` is a Discord.Client object client.on("messageReactionAdd", (reaction, user) => { // Check if the reaction is the one we're looking for if (reaction.emoji.name === "👍") { // Perform some action, e.g., sending a message to the user user.send("Thanks for the thumbs up!"); } });
With this event listener, our bot can now respond to user reactions in real-time!
That's a wrap on adding and managing reactions using Discord.js. With these tools in hand, you can create engaging and interactive bots for your Discord server. Happy coding!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Making a Basic Discord Bot in JavaScript (discord.js) (psst, it's free!).
FAQ
How can I add a reaction to a message using Discord.js?
To add a reaction to a message, you'll want to use the message.react(emoji)
method, where emoji
is the reaction you'd like to add. Here's a simple example:
client.on("message", async (message) => { if (message.content === "!react") { // React with a thumbs up emoji await message.react("👍"); } });
Can I add multiple reactions to a single message?
Yes, you can add multiple reactions to a single message by chaining the message.react(emoji)
method or using a loop. Here's an example:
client.on("message", async (message) => { if (message.content === "!multiReact") { // Array of emojis you'd like to add as reactions const emojis = ["👍", "👎", "❓"]; // Add reactions in order for (const emoji of emojis) { await message.react(emoji); } } });
How can I remove a specific reaction from a message?
To remove a specific reaction from a message, you need to use the message.reactions.resolve(emoji).remove()
method, where emoji
is the reaction you'd like to remove. Here's an example:
client.on("message", async (message) => { if (message.content === "!removeReaction") { // Remove the thumbs up reaction from the message await message.reactions.resolve("👍").remove(); } });
How can I clear all reactions from a message?
To clear all reactions from a message, use the message.reactions.removeAll()
method. Here's an example:
client.on("message", async (message) => { if (message.content === "!clearReactions") { // Clear all reactions from the message await message.reactions.removeAll(); } });
Can I count the number of users who reacted with a specific emoji?
Yes, you can count the number of users who reacted with a specific emoji using the message.reactions.resolve(emoji).count
property. Here's an example:
client.on("message", async (message) => { if (message.content === "!countReactions") { // Get the count of users who reacted with a thumbs up emoji const thumbsUpCount = message.reactions.resolve("👍").count; // Send the count in a message message.channel.send(`👍 reactions: ${thumbsUpCount}`); } });