Diving into Django
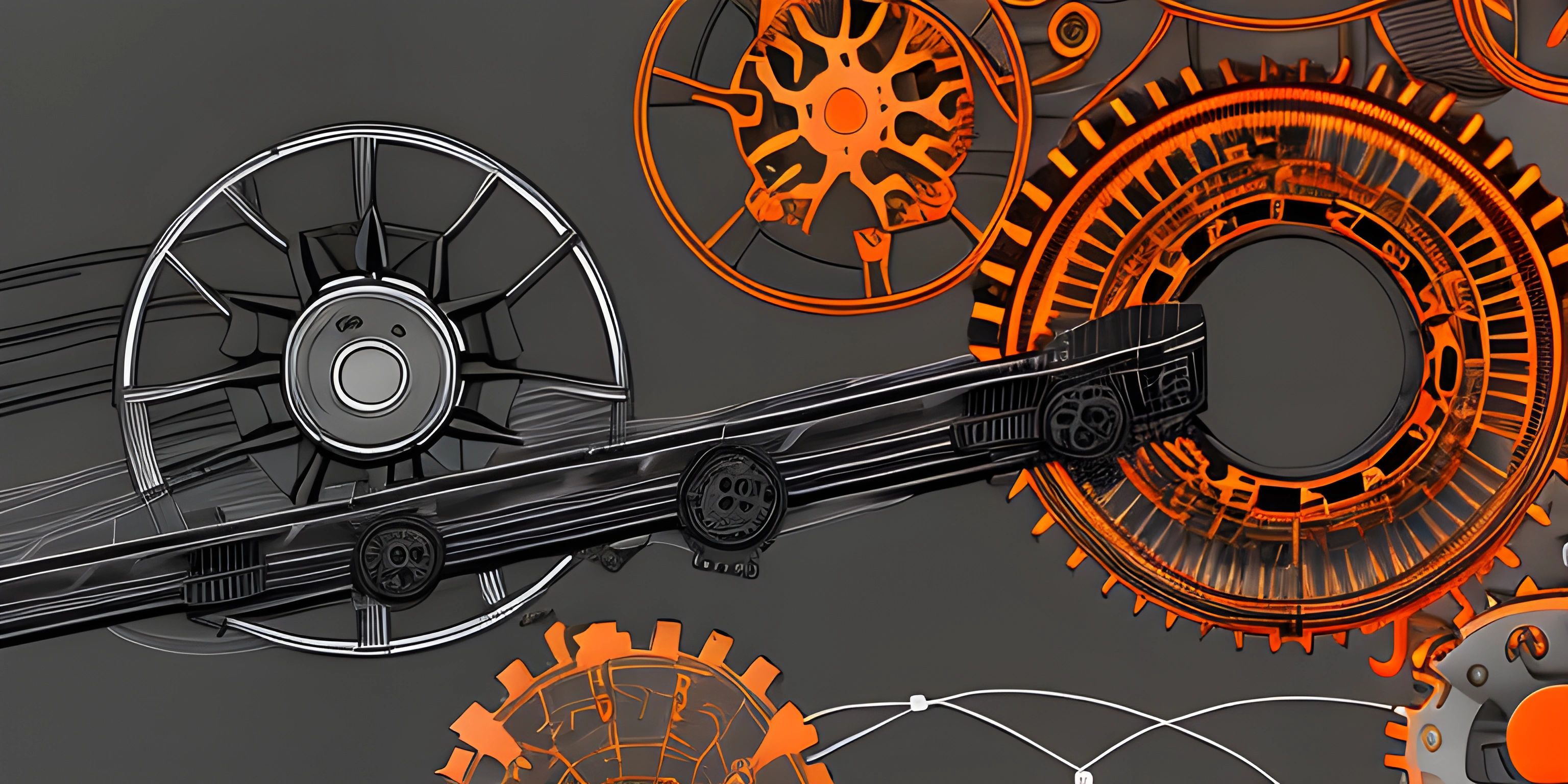
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Django is a high-level Python web framework that enables rapid development of secure and maintainable websites. Built by experienced developers, Django takes care of much of the hassle of web development, so you can focus on writing your app without needing to reinvent the wheel. In this article, we'll dive into Django's basics, from setting up your environment to creating your first project.
Setting Up Your Environment
Before diving into Django, you'll need to have Python installed on your machine. You'll also need to install Django using pip, the Python package manager. To install Django, open your terminal or command prompt and run the following command:
pip install django
This will install the latest version of Django. Now that you have Django installed, let's move on to creating your first project.
Creating Your First Django Project
To create a new Django project, open your terminal or command prompt and navigate to the directory where you want to create the project. Then, run the following command:
django-admin startproject my_project
Replace my_project
with the name you want for your project. This command will generate a new directory named after your project, containing the necessary files for a basic Django project. Let's take a look at the structure of a Django project.
Project Structure
A Django project is composed of several components, each serving a specific purpose:
my_project/
: The main project directory, containing your project's settings and configurations.manage.py
: A command-line utility used for various management tasks, such as running the development server or running tests.my_project/__init__.py
: An empty file that tells Python that this directory should be treated as a package.my_project/settings.py
: Contains settings and configurations for your project, such as database configurations and timezone settings.my_project/urls.py
: Defines the URL patterns for your project, which determine how URLs point to views.my_project/wsgi.py
: An entry point for WSGI (Web Server Gateway Interface) servers, which is a standard interface between web servers and Python web applications.
Now that you're familiar with the basic structure of a Django project, let's create a new Django app within the project.
Creating a Django App
A Django project is composed of one or more apps, each responsible for a specific functionality. To create a new app, open your terminal or command prompt, navigate to your project's root directory, and run the following command:
python manage.py startapp my_app
Replace my_app
with the name you want for your app. This command will generate a new directory named after your app, containing the necessary files for a basic Django app. Let's take a look at the structure of a Django app.
App Structure
A Django app is composed of several components, each serving a specific purpose:
my_app/
: The main app directory, containing your app's files.my_app/__init__.py
: An empty file that tells Python that this directory should be treated as a package.my_app/admin.py
: Contains configurations for Django's admin interface.my_app/apps.py
: Contains the app's configuration class.my_app/migrations/
: Stores migration files, which are used to manage changes to your app's database schema.my_app/models.py
: Contains your app's data models, which define the structure of your app's database tables.my_app/tests.py
: Holds your app's test cases.my_app/views.py
: Contains your app's views, which define how data is displayed and processed.
Now that you have a basic understanding of Django's structure, you can start building your web application. To run your project locally, use the following command:
python manage.py runserver
This will start the development server at http://127.0.0.1:8000/
. You can now start iterating on your project, adding models, views, and templates to build your web application.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Full-stack Web Frameworks (Next.js) (psst, it's free!).
FAQ
What is Django?
Django is a high-level Python web framework that allows for rapid development of secure and maintainable websites. It's built by experienced developers and takes care of much of the hassle of web development, allowing you to focus on writing your app without needing to reinvent the wheel.
How do I install Django?
To install Django, you first need to have Python installed on your machine. You can then install Django using pip, the Python package manager, by running the following command: pip install django
.
What are the main components of a Django project?
A Django project is composed of several components, such as the main project directory, manage.py
, and various configuration files like settings.py
, urls.py
, and wsgi.py
. These components serve different purposes, such as managing the project's settings, defining URL patterns, and providing an entry point for WSGI servers.
What is a Django app?
A Django app is a self-contained module within a Django project that is responsible for a specific functionality. A Django project can have one or more apps, each with its own models, views, and templates.
How do I create a new Django app?
To create a new Django app, open your terminal or command prompt, navigate to your project's root directory, and run the following command: python manage.py startapp my_app
, replacing my_app
with the name you want for your app. This will generate a new directory containing the necessary files for a basic Django app.